Introduction: Why API Rate Limiting is Crucial?
APIs (Application Programming Interfaces) are the backbone of modern applications, enabling seamless communication between different services. However, without proper rate limiting, APIs can suffer from excessive requests, leading to server overload, downtime, and security vulnerabilities.
API rate limiting is a critical mechanism that controls how many requests a client can make to an API within a specific time period. It ensures fair usage, prevents abuse, and optimizes server performance.
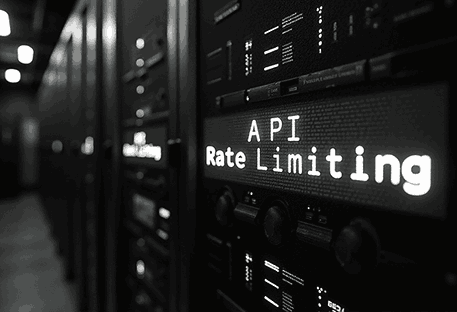
In this guide, we’ll explore:
What API rate limiting is and why it matters
Different types of rate limiting techniques
How to implement rate limiting in APIs
Best practices to prevent API abuse
Common challenges and solutions in rate limiting
By the end, you’ll have a deep understanding of API rate limiting and how to implement it effectively in your applications.
1. What is API Rate Limiting?
API rate limiting is a control mechanism that restricts the number of API requests a client (user, application, or IP) can make in a given period. This prevents servers from being overwhelmed and protects against Denial of Service (DoS) attacks, bot abuse, and system crashes.
Key Components of Rate Limiting:
Request Limit: The maximum number of requests allowed per second, minute, or hour.
Time Window: The duration in which the request limit applies.
Client Identifier: API key, IP address, or user account used to track request counts.
For example, an API might allow 100 requests per minute per user. Once the limit is exceeded, further requests may be blocked, delayed, or charged extra.
2. Why is API Rate Limiting Important?
API rate limiting is essential for:
✅ Preventing API Abuse & DDoS Attacks
Protects APIs from malicious traffic spikes
Stops automated bots from flooding the system
✅ Ensuring Fair Usage
Prevents a single user from consuming all available resources
Ensures equal API access to all users
✅ Maintaining Server Performance & Scalability
Helps APIs handle high traffic loads efficiently
Reduces server downtime and slow response times
✅ Enforcing API Monetization Models
Supports tiered pricing plans (e.g., free users get 1,000 requests/month, premium users get unlimited access)
Helps control costs and improve revenue
3. Types of API Rate Limiting Strategies
There are several methods used to implement API rate limiting. The choice depends on your application’s needs and traffic patterns.
3.1 Fixed Window Rate Limiting
Limits requests based on a fixed time window (e.g., 100 requests per hour).
Simple but can lead to request spikes at the start of each window.
3.2 Sliding Window Rate Limiting
Allows requests based on a moving time window (e.g., last 60 minutes).
Provides smoother request distribution over time.
3.3 Token Bucket Algorithm
Uses a bucket filled with tokens that represent allowed API calls.
Each request removes one token; tokens are replenished at a fixed rate.
Ensures controlled request bursts without overloading the system.
3.4 Leaky Bucket Algorithm
Similar to the token bucket but leaks requests at a constant rate.
Prevents sudden traffic spikes by processing requests evenly over time.
3.5 Rate Limiting by User/IP
Limits requests based on API key, user ID, or IP address.
Useful for multi-tenant applications with different usage tiers.
4. How to Implement API Rate Limiting
4.1 Implementing Rate Limiting in Different API Frameworks
🔹 Node.js (Express.js) Example
Using the express-rate-limit package:
javascript
CopyEdit
const rateLimit = require("express-rate-limit");
const limiter = rateLimit({
windowMs: 15 60 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per window
message: "Too many requests from this IP, please try again later."
});
app.use(limiter);
🔹 Python (Flask) Example
Using the Flask-Limiter package:
python
CopyEdit
from flask import Flask
from flask_limiter import Limiter
app = Flask(__name__)
limiter = Limiter(app, key_func=lambda: request.remote_addr)
@app.route("/api")
@limiter.limit("10 per minute")
def api():
return "API Response"
4.2 Using Cloud-Based Rate Limiting Services
AWS API Gateway – Provides built-in rate limiting.
Cloudflare Rate Limiting – Protects APIs from bot traffic.
Google Cloud API Gateway – Controls request quotas.
5. Best Practices for API Rate Limiting
✅ Use tiered rate limits for different users (e.g., free vs. premium plans).
✅ Provide clear error messages when users exceed rate limits.
✅ Allow users to monitor their API usage via dashboards.
✅ Implement retry mechanisms (e.g., exponential backoff).
✅ Log and analyze API traffic to identify potential abuse patterns.
6. Challenges in API Rate Limiting & How to Overcome Them
Challenge 1: Handling Spikes in Traffic
Solution: Use dynamic rate limits that adjust based on real-time server load.
Challenge 2: Managing Multiple Clients with Different Usage Needs
Solution: Implement customized rate limits based on API keys or user plans.
Challenge 3: Preventing Legitimate Users from Being Blocked
Solution: Allow grace periods or request queuing instead of outright blocking.
7. Conclusion
API rate limiting is a crucial technique for maintaining API reliability, preventing abuse, and optimizing performance. By implementing smart rate-limiting strategies like token buckets, sliding windows, and cloud-based solutions, businesses can protect their APIs from overload and ensure fair usage for all users.
Following best practices such as clear error messages, tiered limits, and retry mechanisms can further enhance the API user experience.
8. FAQs
1. What happens if I exceed an API rate limit?
Most APIs return an HTTP 429 Too Many Requests error. Some may throttle or block your requests temporarily.
2. Can I bypass API rate limits?
No. Bypassing rate limits violates API terms of service and may lead to IP bans or legal consequences.
3. How do I know my API’s ideal rate limit?
Analyze traffic patterns and server capacity to set balanced rate limits that prevent abuse while ensuring smooth user experience.
4. How do cloud providers handle API rate limiting?
AWS, Google Cloud, and Azure provide built-in rate-limiting features that allow developers to define usage policies.
Key Takeaways
✔ API rate limiting controls how many requests a client can make in a given period.
✔ Prevents server overload, DDoS attacks, and API abuse.
✔ Implemented using fixed windows, token buckets, and sliding windows.
✔ Best practices include clear error messages, retry mechanisms, and tiered limits.
✔ Cloud-based solutions offer scalable rate limiting without infrastructure overhead.
For example, 99papers.com offers writing services, including articles, scripts, and marketing materials. https://99papers.com/ This can be useful if you need to write a press release, prepare a review, or write text for a gaming blog.
API rate limiting ensures fair usage and prevents server overload, much like streaming services manage traffic for Watch anime online. Developers set limits to balance performance and security, avoiding excessive requests that could crash systems. Just as anime fans need smooth playback, APIs need controlled access for optimal functionality.
iron4d
| situs slot gacor terpercaya | situs slot gacor terpercaya | situs slot gacor terpercaya |
Hongtogel - Slot Gacor 💋 Situs Toto Bet 200 Rupiah 4D Maxwin Hari Ini
Jika Anda mencari situs untuk bermain Hongtogel, di sini Anda dapat menikmati berbagai permainan menarik dengan Slot Gacor yang menawarkan peluang menang besar. Situs Toto Bet ini juga memiliki pilihan 4D Maxwin untuk para pemain yang mencari keuntungan maksimal.
HONGTOGEL — Situs Toto 4D Terpercaya Bandar Togel Online Resmi
HONGTOGEL merupakan salah satu Situs Toto 4D yang terpercaya, menyediakan berbagai permainan dengan sistem yang aman dan hadiah besar. Jika Anda mencari Bandar Togel Online Resmi, maka HONGTOGEL adalah pilihan tepat.
HONGTOGEL > Situs Toto 4D dan Bandar Togel HK Terpercaya Togel Hongkong
Di HONGTOGEL, Anda dapat menikmati permainan Situs Toto 4D terbaik yang juga menawarkan Bandar Togel HK dengan reputasi tinggi, memberikan para…
ARIZONA88 ⚡ Situs Toto 4D Bandar Togel Online Mudah Menang Tiap Hari
Jika Anda mencari ARIZONA88, situs terbaik untuk bermain Situs Toto 4D dan Bandar Togel Online yang selalu memberikan kesempatan menang setiap hari, ini adalah pilihan yang tepat untuk Anda.
Toto Macau § Bandar Toto Macau Keluaran Togel Macau
Untuk penggemar Toto Macau, situs ini menyediakan informasi lengkap mengenai Bandar Toto Macau dan Keluaran Togel Macau terbaru yang sangat akurat.
Hongtogel - Bandar Togel Online dan Situs Toto 4D Terbaik 2025
Hongtogel merupakan pilihan utama bagi pemain yang mencari Bandar Togel Online dan Situs Toto 4D terpercaya dengan berbagai keuntungan menarik.
HONGTOGEL ⭐ Situs Toto 4D dan Bandar Togel Online Hadiah Terbesar
HONGTOGEL adalah salah satu situs Situs Toto 4D terbaik, menyediakan berbagai Bandar Togel Online dengan hadiah terbesar…