Mastering API Schema Validation: Your Ultimate Guide
- Gunashree RS
- Dec 27, 2024
- 5 min read
Introduction to API Schema Validation
APIs (Application Programming Interfaces) play a vital role in modern software development, enabling communication between systems and applications. API schema validation ensures that APIs adhere to predefined structures and rules, which safeguards their functionality, consistency, and reliability.
Whether you're an experienced developer or a beginner in the tech world, understanding API schema validation is critical for building robust and scalable applications. In this guide, we’ll dive deep into the concept, its importance, best practices, tools, and much more.
What is API Schema Validation?
API schema validation is the process of verifying that the data sent or received through an API complies with a predefined schema. A schema defines the structure, format, and constraints of the data, ensuring standardization and preventing errors during data exchange.
For example, when a client sends data to an API endpoint, schema validation ensures that the data matches the expected fields, data types, and constraints.
Key Components of a Schema
Fields: Specify the data attributes (e.g., name, email).
Data Types: Define the type of each field (e.g., string, integer).
Constraints: Establish rules (e.g., required fields, maximum length).
Why is Schema Validation Essential?
Error Prevention: Catch errors early by enforcing structure and format.
Security: Prevent malicious or malformed data from entering the system.
Consistency: Ensure seamless communication between API consumers and providers.
Efficiency: Save debugging time by identifying issues upfront.
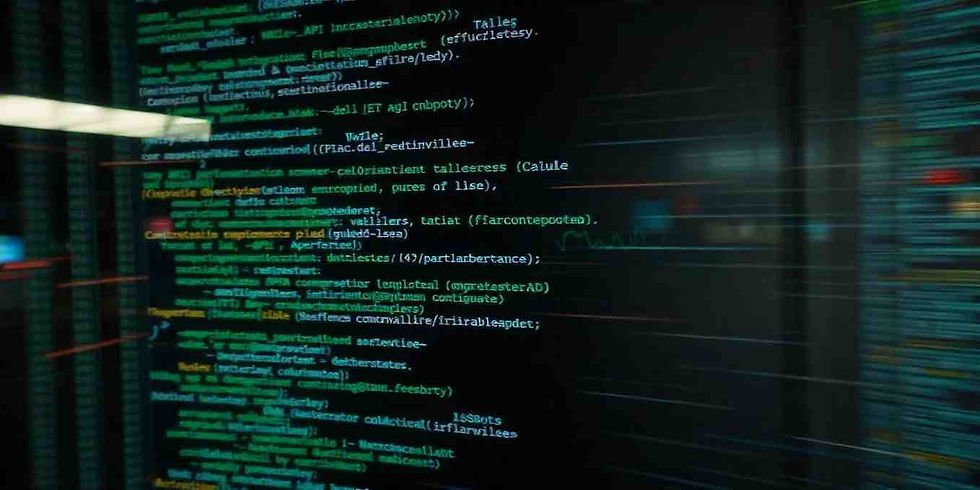
Benefits of API Schema Validation
1. Enhanced Security
By validating input and output data, schema validation acts as the first line of defense against SQL injections, cross-site scripting (XSS), and other cyberattacks.
2. Improved API Reliability
Ensuring that APIs only process valid data prevents crashes and runtime errors.
3. Faster Development
Schema validation allows developers to identify inconsistencies early, reducing time spent on troubleshooting.
4. Simplified Debugging
Validation errors provide clear feedback, making it easier to pinpoint and resolve issues.
5. Better API Documentation
Schemas serve as documentation for the API's data structure, simplifying integration for consumers.
How API Schema Validation Works
1. Define the Schema
Create a schema using standards like JSON Schema or OpenAPI. For example:
JSON
{
"type": "object",
"properties": {
"name": { "type": "string" },
"age": { "type": "integer" }
},
"required": ["name", "age"]
}
2. Implement Validation
Integrate validation logic in the API. Most frameworks provide middleware or libraries for this purpose.
3. Validate Requests and Responses
Validate incoming requests before processing and verify outgoing responses to ensure compliance.
4. Handle Validation Errors
Return meaningful error messages to guide users and developers:
JSON
{
"error": "Validation failed",
"details": ["'name' is required", "'age' must be an integer"]
}
Common Tools for API Schema Validation
1. JSON Schema Validator
A popular choice for validating JSON data against schemas.
2. OpenAPI (Swagger)
Provides comprehensive support for defining and validating API schemas.
3. AJV
A fast JSON Schema validator for JavaScript.
4. Joi
A powerful library for schema validation in Node.js.
5. Postman
Enables validation during API testing.
6. SOAPUI
For validating APIs based on SOAP or REST protocols.
7. RAML (RESTful API Modeling Language)
A specification tool that assists in schema validation and API design.
Best Practices for API Schema Validation
1. Use Standardized Formats
Stick to widely accepted standards like JSON Schema or OpenAPI for better compatibility.
2. Validate Both Requests and Responses
Ensure that APIs are handling data correctly on both ends.
3. Keep Schemas Updated
As APIs evolve, regularly update schemas to reflect new requirements.
4. Provide Clear Error Messages
Make validation errors descriptive and user-friendly.
5. Test Extensively
Run automated and manual tests to identify edge cases and ensure validation effectiveness.
6. Implement Security Checks
Combine schema validation with authentication and authorization mechanisms.
Challenges in API Schema Validation
1. Complex Data Structures
Validating deeply nested or complex schemas can be challenging.
2. Performance Overheads
Frequent validation checks may impact API performance, especially for large datasets.
3. Handling Backward Compatibility
When updating schemas, ensure compatibility with older versions of the API.
4. Dynamic APIs
For APIs that accept dynamic or flexible data structures, validation can be more complex.
Examples of API Schema Validation
Example 1: Validating a User Registration API
JSON
{
"type": "object",
"properties": {
"username": { "type": "string", "minLength": 5 },
"password": { "type": "string", "minLength": 8 },
"email": { "type": "string", "format": "email" }
},
"required": ["username", "password", "email"]
}
This schema ensures all necessary fields are provided and meet the required format and length.
Example 2: Validating a Product API
JSON
{
"type": "object",
"properties": {
"id": { "type": "integer" },
"name": { "type": "string" },
"price": { "type": "number", "minimum": 0 },
"tags": {
"type": "array",
"items": { "type": "string" }
}
},
"required": ["id", "name", "price"]
}
This schema ensures that each product has an ID, name, and valid price.
Future Trends in API Schema Validation
1. AI-Powered Validation Tools
Emerging tools leverage AI to optimize schema validation, identify anomalies, and predict errors.
2. Increased Adoption of GraphQL
GraphQL APIs rely on type systems for validation, offering a unique approach compared to REST.
3. Enhanced Tool Integration
Future tools will provide seamless integration with CI/CD pipelines for automated schema validation.
FAQs
1. What is the purpose of API schema validation?
API schema validation ensures that the data exchanged through APIs adheres to a predefined structure, preventing errors and enhancing security.
2. Which tools are best for API schema validation?
Popular tools include JSON Schema, OpenAPI, Postman, AJV, Joi, and RAML.
3. Can schema validation improve API performance?
While it may introduce slight overhead, schema validation prevents runtime errors and ensures data integrity, leading to better overall performance.
4. How often should API schemas be updated?
Schemas should be updated whenever there are changes in API requirements or functionality.
5. Is schema validation necessary for all APIs?
While not mandatory, schema validation is strongly recommended for APIs that handle critical or sensitive data.
6. How does schema validation enhance API security?
By filtering invalid or malicious inputs, schema validation reduces vulnerabilities to cyberattacks like SQL injection and XSS.
7. What happens if schema validation fails?
The API should return a descriptive error message, guiding the user or developer to fix the issue.
8. Can schema validation be automated?
Yes, many tools support automated schema validation as part of CI/CD workflows.
Conclusion
API schema validation is a cornerstone of modern API development, ensuring data consistency, security, and seamless integration. By adhering to best practices and leveraging the right tools, developers can build reliable and scalable APIs that stand the test of time.
Whether you're starting your journey in API development or looking to enhance existing systems, investing in schema validation will pay dividends in reliability and user satisfaction.
Key Takeaways
API schema validation ensures data consistency and security.
Use tools like JSON Schema, OpenAPI, and Postman for validation.
Follow best practices like updating schemas and providing clear error messages.
Validate both requests and responses for comprehensive data integrity.
Comments