Introduction
In the world of web development and testing, automation is key to efficiency and accuracy. With Continuous Integration (CI) and Continuous Delivery (CD) becoming standard practices, tools like Selenium have become indispensable for automated testing. However, automating the testing of browser extensions, particularly Chrome extensions, presents unique challenges.
This comprehensive guide will delve into the specifics of using the ChromeDriver Plugin to test Chrome extensions. We'll explore the difficulties, provide solutions, and offer a detailed, step-by-step process to streamline your testing efforts. Whether you're a seasoned developer, a QA tester, or a newcomer to automated testing, this guide will equip you with the knowledge and tools to master Chrome extension testing using Selenium and ChromeDriver.
What is ChromeDriver Plugin?
Overview of Selenium and ChromeDriver
Selenium is a popular open-source tool for automating web browsers. It supports multiple programming languages and can control browsers through programs and record/playback features. ChromeDriver is a standalone server that implements the WebDriver's wire protocol for Chromium, enabling automated control over the Google Chrome browser.
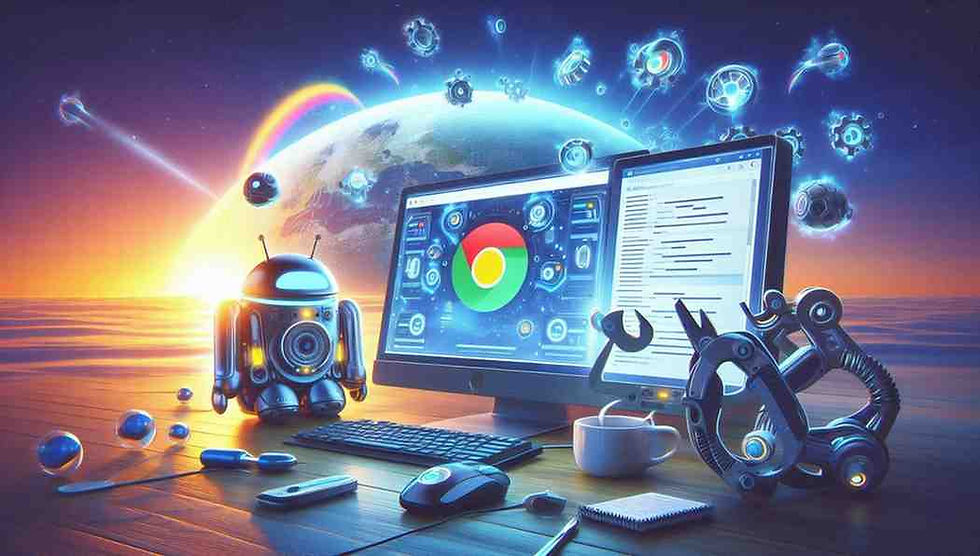
Importance of ChromeDriver Plugin
The ChromeDriver Plugin is crucial for testing Chrome extensions because it allows developers and testers to automate interactions with Chrome extensions, just as they would with regular web pages. This capability is essential for ensuring that extensions function correctly and provide a seamless user experience.
Why Test Chrome Extensions with ChromeDriver?
Challenges in Testing Browser Extensions
Testing browser extensions can be challenging because they are not regular HTML files but embedded add-ons. This makes it difficult to simulate user actions, inspect web elements, and automate interactions. Extensions often involve unique pages and behaviors that are out of the typical web page scope.
Benefits of Automated Extension Testing
Automated testing of Chrome extensions using ChromeDriver offers several benefits:
Efficiency: Automates repetitive tasks, saving time and effort.
Accuracy: Reduces human error, ensuring consistent test results.
Coverage: Allows comprehensive testing across different scenarios and configurations.
CI/CD Integration: Easily integrates with CI/CD pipelines for continuous testing and delivery.
Step-by-Step Guide to Testing Chrome Extensions with chromedriver
Step 1: Download Chrome Extension
To begin testing, you need the CRX file of the Chrome extension. Follow these steps to download it:
Obtain the webstore URL or extension ID from the Google Web Store.
Enter the URL/ID into the main field on Chrome Extension Downloader.
Click "Download" and save the CRX file in the same location as your script.
Step 2: Download Chrome Extension Source Viewer
Install the Chrome Extension Source Viewer from the Google Web Store to view the contents of the CRX file.
Step 3: View Source of the CRX File
To inspect the CRX file:
Open the Chrome Extensions page in the Google Web Store.
Click the yellow icon in the browser and select "View Source."
If the CRX file is not in the Web Store, manually upload it here.
Step 4: Identify the Page to be Tested
Locate the specific page within the extension to be tested by following these steps:
Extract the unique ID of the Chrome extension.
Right-click on the extension and select "Options" to get the unique ID page URL: chrome-extension://UNIQUEID/options.html.
Change the URL to point to the specific page, e.g., chrome-extension://UNIQUEID/SPECIFICPAGE.html.
Copy this URL for use in the WebDriver code.
Step 5: Initiate Selenium Script to Create a New ChromeDriver
Create a new ChromeDriver instance with the extension loaded. Below is the code syntax in various programming languages:
Java
java
ChromeOptions options = new ChromeOptions();
options.addExtensions(new File("/path/to/extension.crx"));
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(ChromeOptions.CAPABILITY, options);
ChromeDriver driver = new ChromeDriver(capabilities);
Python
python
from selenium import webdriver
chop = webdriver.ChromeOptions()
chop.add_extension('/path/to/extension.crx')
driver = webdriver.Chrome(options=chop)
JavaScript
javascript
var webdriver = require('selenium-webdriver');
var chrome = require('selenium-webdriver/chrome');
var options = new chrome.Options();
options.addExtensions('/path/to/extension.crx');
var driver = new webdriver.Builder().forBrowser('chrome').setChromeOptions(options).build();
PHP
php
use Facebook\WebDriver\Chrome\ChromeOptions;
use Facebook\WebDriver\Remote\DesiredCapabilities;
use Facebook\WebDriver\Remote\RemoteWebDriver;
$options = new ChromeOptions();
$options->addExtensions(['/path/to/extension.crx']);
$caps = DesiredCapabilities::chrome();
$caps->setCapability(ChromeOptions::CAPABILITY, $options);
$driver = RemoteWebDriver::create('http://localhost:4444', $caps);
Step 6: Navigate to the Chrome Extension Page
Use the ChromeDriver to navigate to the extension page:
python
driver.get('chrome-extension://UNIQUEID/SPECIFICPAGE.html')
Step 7: Interact with the Extension
Once on the extension page, you can interact with it as you would with a normal HTML webpage. If the extension page includes an iframe, switch to it using the following syntax:
PHP
php
$this->selectFrame("FRAME_NAME");
Python
python
driver.switch_to.frame("FRAME_NAME");
JavaScript
javascript
driver.switchTo().frame("FRAME_NAME");
Java
java
driver.switchTo().frame("FRAME_NAME");
Best Practices for Testing Chrome Extensions
Test on Multiple Versions
Ensure compatibility by testing on multiple versions of Chrome and the extension. Users may not always update to the latest version, so covering different versions is essential.
Performance Testing
Test the extension's performance to ensure it doesn't slow down the browser or consume excessive resources.
Security Testing
Ensure the extension doesn't introduce security vulnerabilities, such as phishing risks or malware.
User Experience
Test the extension's usability to ensure it provides a seamless user experience. This includes checking for intuitive navigation, clear instructions, and responsive design.
Troubleshooting Common Issues
Extension Not Loading
Ensure the CRX file is correctly downloaded and added to the ChromeDriver instance. Check for any errors in the path or file permissions.
Element Not Found
If WebDriver cannot locate elements, ensure the correct iframe is selected, and the element locators are accurate.
Performance Issues
Optimize the extension code and reduce resource consumption. Use performance profiling tools to identify bottlenecks.
Conclusion
Automating the testing of Chrome extensions using Selenium and ChromeDriver can significantly streamline the testing process, ensuring accuracy and efficiency. By following the step-by-step guide outlined in this article, developers and testers can overcome the unique challenges of extension testing and deliver high-quality extensions that provide a seamless user experience.
Key Takeaways
ChromeDriver Plugin: Essential for automating the testing of Chrome extensions with Selenium.
Efficiency: Automation saves time and reduces human error.
Coverage: Comprehensive testing across different scenarios and configurations.
Performance: Ensure extensions do not slow down the browser.
Security: Identify and fix security vulnerabilities.
User Experience: Provide a seamless and intuitive user experience.
FAQs
Why is testing your Chrome extension critical?
Testing Chrome extensions ensures they function correctly and provide a good user experience. It helps identify and fix bugs, performance issues, and security vulnerabilities.
How can I download a Chrome extension for testing?
You can download a Chrome extension from the Google Web Store using its URL or ID. Use tools like Chrome Extension Downloader to save the CRX file.
What is the ChromeDriver Plugin?
The ChromeDriver Plugin is a tool that allows automated control over the Chrome browser, enabling the testing of Chrome extensions with Selenium.
How do I view the source of a CRX file?
Use the Chrome Extension Source Viewer to inspect the contents of a CRX file. Alternatively, manually upload the CRX file to view its source.
How do I initiate a Selenium script with a Chrome extension?
Create a new ChromeDriver instance and add the extension to the ChromeOptions. Use this instance to automate interactions with the extension.
What are common issues when testing Chrome extensions?
Common issues include the extension not loading, elements not found, and performance problems. Ensure correct file paths, element locators, and optimize the extension code.
How can I improve the performance of my Chrome extension?
Optimize the extension's code, reduce resource consumption, and use performance profiling tools to identify and address bottlenecks.
What are the benefits of automated extension testing?
Automated testing saves time, reduces human error, provides comprehensive coverage, and integrates with CI/CD pipelines for continuous testing and delivery.
Comments