Introduction
In the ever-evolving landscape of software development, the need for efficient and effective testing frameworks is paramount. Cucumber, an open-source tool written in Ruby, has emerged as a popular choice among developers and testers alike. It facilitates Behavior-driven Development (BDD), enabling teams to write test cases in plain language that both technical and non-technical stakeholders can understand. This article delves into the intricacies of the Cucumber Framework, its benefits, its working mechanism, and its integration with other tools like Selenium.
What is the Cucumber Framework?
The Cucumber Framework is an automated testing tool designed to execute acceptance tests written in a domain-specific language called Gherkin. Gherkin's syntax allows the creation of test scenarios in plain English, making it accessible to all stakeholders, including business analysts, developers, and testers. The framework serves as a bridge between business and technical teams, fostering better communication and collaboration.
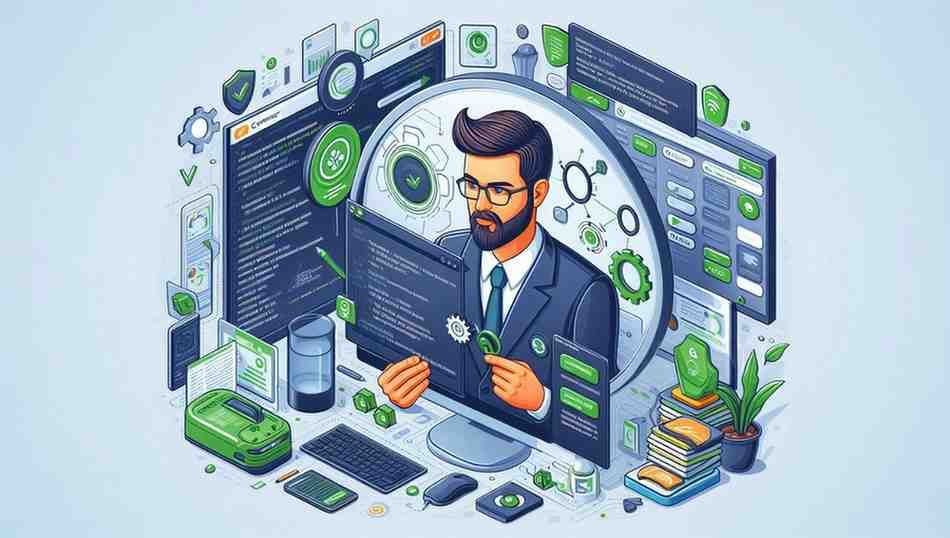
Components of Cucumber Framework
Feature File: This file contains scenarios written in Gherkin, describing the application's behavior in a business-readable format.
Step Definition File: This file maps the steps in the feature file to the actual code that performs the operations.
Test Runner File: This file is responsible for executing the Cucumber tests by coordinating the feature files and step definitions.
Benefits of Using Cucumber Testing Tools
Cucumber offers a myriad of advantages that make it a preferred choice for automated testing.
Involving Stakeholders
One of the most significant benefits of Cucumber is its ability to involve stakeholders regardless of their programming knowledge. Since test cases are written in plain English, business analysts, product owners, and other non-technical team members can easily understand and contribute to the testing process.
Ease of Writing Test Scripts
Testers can write test scripts without having in-depth knowledge of programming. The Gherkin syntax simplifies the creation of test cases, enabling testers to focus on the test logic rather than the underlying code.
Speed and Efficiency
Plugins in Cucumber are faster compared to other frameworks like Selenium. This speed enhances the efficiency of the testing process, allowing for quicker feedback and iterations.
Support for Multiple Languages
Cucumber supports various programming languages, including Java, Ruby, and Python. This flexibility allows teams to choose the language they are most comfortable with, integrating Cucumber seamlessly into their existing workflows.
Reusability of Code
Cucumber promotes code reusability by allowing the same step definitions to be used across multiple test scenarios. This reduces redundancy and improves the maintainability of the test suite.
Simple Setup
Setting up Cucumber is straightforward and quick. It integrates well with different software platforms like Selenium, Ruby on Rails, Watir, and Spring Framework, making it a versatile tool for various testing needs.
Cross-Platform Flexibility
Cucumber is flexible with different software platforms, enabling integration with tools like Selenium, Watir, Ruby on Rails, and Spring Framework. This versatility makes it a valuable asset in any testing toolkit.
How Does Cucumber Work?
Cucumber operates on a simple yet powerful mechanism, leveraging three main components to execute tests.
Feature File
Cucumber tests are written in plain text files called feature files, which have the ".feature" extension. These files describe the software's behavior using Gherkin syntax, making the documentation more legible. Gherkin uses keywords like Given, When, and Then to define the steps of a test scenario.
Step Definitions
Each step in a feature file is associated with a step definition implemented in the code. Step definitions define the actions or operations that must be executed for each step of the test scenario. They map the plain text steps in the feature file to the corresponding code implementation.
Test Runner File
The test runner file in Cucumber is responsible for executing the Cucumber feature files and coordinating the steps defined in those feature files with the corresponding step definitions. It acts as the central point of execution for Cucumber tests.
Example of Cucumber Test
To better understand how Cucumber works, let's look at an example of a Cucumber test for checking the login functionality of an existing user.
Feature File
gherkin
Feature: Login functionality
Scenario: As an existing user, I want to log in successfully
Given the user is on the Home page
When the user navigates to the Login page
The user enters the username and password
Then the successful login message is displayed
Step Definition File
java
public class LoginSteps {
@Given("^the user is on the Home page$")
public void userOnHomePage() {
// Code to navigate to Home page
}
@When("^the user navigates to the Login page$")
public void userNavigatesToLoginPage() {
// Code to navigate to the Login page
}
@When("^the user enters the username and password$")
public void user enters credentials() {
// Code to enter username and password
}
@Then("^the successful login message is displayed$")
public void successfulLoginMessageDisplayed() {
// Code to verify the successful login message
}
}
Test Runner File
java
@RunWith(Cucumber.class)
@CucumberOptions(
features = "src/test/resources/features",
glue = "stepDefinitions"
)
public class TestRunner {
}
BDD in Cucumber Automation
Behavior-driven Development (BDD) is a software development technique that extends Test-driven Development (TDD) by emphasizing collaboration between developers, testers, and business stakeholders. BDD uses a shared language to enhance communication and ensure that all team members have a clear understanding of the requirements.
Given-When-Then Syntax
The Given-When-Then syntax is a cornerstone of BDD, providing a structured way to write test scenarios.
Given describes the initial context of the system.
When specifies the action or event that triggers the scenario.
Then defines the expected outcome or result.
Example of BDD in Cucumber
Consider the following example for a better understanding of BDD in Cucumber:
gherkin
Scenario: Invalid login attempt
Given the user has entered invalid credentials
When the user clicks the submit button
Then display the proper validation message
Benefits of BDD in Cucumber Framework
BDD in Cucumber offers several benefits that enhance the software development process.
Focus on Behavior
BDD emphasizes defining the system's behavior rather than writing tests. This approach ensures that all team members have a clear understanding of how the system should perform from both the developer's and customer's perspectives.
Improved Communication
By using a shared language, BDD enhances communication among the members of a cross-functional product team. It bridges the gap between technical and non-technical team members, fostering better collaboration.
Wider Audience Reach
The non-technical language used in BDD allows a broader audience to participate in the testing process. Business analysts, product owners, and other stakeholders can easily understand and contribute to the test scenarios.
Reduced Maintenance Costs
The improvement in code quality resulting from BDD reduces the costs of maintenance and minimizes the project's associated risks. BDD promotes the development of robust and reliable software.
Limitations of Behavior-Driven Development
While BDD offers numerous benefits, it also has some limitations that should be considered.
Prerequisite Knowledge of TDD
Testers must have prior experience in TDD to work effectively in BDD. The transition from TDD to BDD can be challenging for those unfamiliar with test-driven development practices.
Requirement Analysis
BDD can be ineffective if the requirements are not correctly analyzed. Clear and precise requirements are essential for creating meaningful test scenarios.
Technical Skills
Testers working with BDD must possess sufficient technical skills to write and maintain step definitions. The collaborative nature of BDD requires a balance of technical and business knowledge.
Cucumber with Selenium
Many organizations prefer integrating Cucumber with Selenium for cross-browser compatibility testing. Selenium's robust browser automation capabilities, combined with Cucumber's easy-to-understand syntax, make it a powerful combination for automated testing.
Benefits of Integrating Cucumber with Selenium
Readability: Gherkin syntax involves simple and plain text, making it easier for team members to understand test cases.
Cross-Browser Testing: Selenium allows testing across multiple browsers and platforms, ensuring a consistent user experience.
Real-Device Testing: BrowserStack supports running Selenium tests on real devices, enhancing the reliability of test results.
Running Cucumber Selenium Tests
Set Up BrowserStack Account: Sign up for BrowserStack and choose the required device-browser-OS combination.
Upload Test Cases: Use BrowserStack Test Management to upload BDD-JSON based reports using Cucumber.
Execute Tests: Run Selenium Cucumber tests on real browsers and devices to validate the application's behavior.
Conclusion
Cucumber Framework Testing is a powerful approach to automated testing that promotes collaboration, readability, and efficiency. By enabling stakeholders to understand and contribute to the testing process, Cucumber bridges the gap between business and technical teams. Its integration with tools like Selenium further enhances its capabilities, making it a versatile choice for modern software development.
Key Takeaways
Cucumber Framework: An automated testing tool that executes acceptance tests written in Gherkin.
Benefits: Improved stakeholder involvement, ease of writing test scripts, support for multiple languages, and code reusability.
How It Works: Utilizes feature files, step definitions, and test runner files to execute tests.
Example: Illustrated with a login functionality test scenario.
BDD in Cucumber: Emphasizes behavior over tests, improves communication, and reduces maintenance costs.
Limitations: Requires knowledge of TDD, effective requirement analysis, and technical skills.
Integration with Selenium: Enhances readability and supports cross-browser testing on real devices.
Conclusion: Cucumber promotes collaboration, readability, and efficiency in automated testing.
FAQs
What is the Cucumber Framework?
The Cucumber Framework is an automated testing tool that executes acceptance tests written in Gherkin, a plain text language. It facilitates Behavior-driven Development (BDD) by enabling stakeholders to write test cases in a language they can understand.
What are the benefits of using Cucumber Testing Tools?
Benefits include improved stakeholder involvement, ease of writing test scripts without deep programming knowledge, faster plugins, support for multiple programming languages, code reusability, simple setup, and cross-platform flexibility.
How does Cucumber work?
Cucumber works by using feature files written in Gherkin, step definition files that map the steps to code, and test runner files that execute the tests. It coordinates the actions and verifies the expected outcomes.
Can you give an example of a Cucumber test?
An example is a login functionality test for an existing user. The feature file describes the scenario, the step definition file maps the steps to code, and the test runner file executes the test.
What is BDD in Cucumber Automation?
BDD in Cucumber Automation is a development approach that focuses on defining the system's behavior rather than writing tests. It uses a shared language to enhance communication and collaboration among team members.
What are the benefits of BDD in the Cucumber Framework?
Benefits include a focus on behavior, improved communication, wider audience reach, reduced maintenance costs, and enhanced code quality.
What are the limitations of Behavior-driven Development?
Limitations include the need for prior knowledge of TDD, effective requirement analysis, and sufficient technical skills for testers.
How does Cucumber integrate with Selenium?
Cucumber integrates with Selenium for cross-browser testing. Selenium's browser automation capabilities combined with Cucumber's easy-to-understand syntax make it a powerful combination for automated testing.
Comments