In the software development lifecycle (SDLC), testing is a critical step that ensures the quality and reliability of an application before it reaches users. Among the various testing methodologies, functional testing and unit testing play vital roles in verifying that software meets the required standards. Understanding the difference between functional testing and unit testing is essential for developers, testers, and project managers to ensure that each component and the overall application function as expected.
This comprehensive guide dives into the definitions, differences, examples, and best practices of functional testing and unit testing. By the end of this article, you'll know how each type of testing fits into the development process and when to apply them for maximum effectiveness.
What is Functional Testing?
Functional Testing is a software testing type that validates the functionality of an application based on the business requirements. It ensures that the application performs its intended functions and meets user expectations. Functional testing involves testing the entire application from an end-to-end perspective, focusing on user interactions and the overall behavior of the system.
Key Aspects of Functional Testing:
Objective: To verify that the application functions according to business requirements.
Scope: Tests are conducted on the entire application or specific modules to ensure user flows, data input and output, and UI functionality.
User Perspective: Functional testing simulates how a user would interact with the software, checking if the user experience is smooth and intuitive.
Not Focused on Code: Unlike unit testing, functional testing does not concern itself with the internal code structure; it only checks if the output meets the expected result.
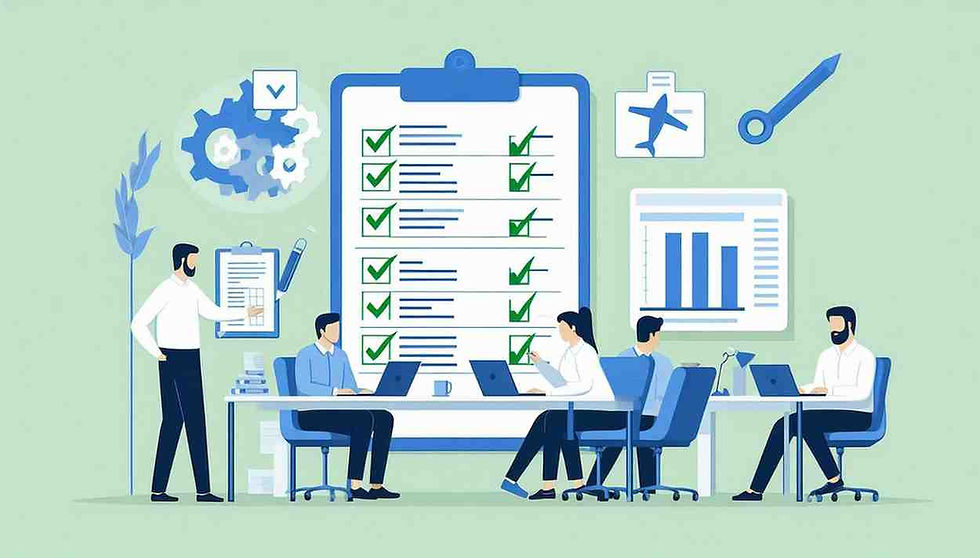
Types of Functional Testing:
Functional testing encompasses various types, including:
Unit Testing (as a subset of functional testing)
Integration Testing
System Testing
User Acceptance Testing (UAT)
Regression Testing
Sanity and Smoke Testing
These testing methods together ensure that the software behaves correctly under different conditions and meets user needs.
Functional Testing Example:
Let’s consider an e-commerce website and test the order history functionality:
Test Case 1: Logging in to the Application
Step 1: Enter a valid username and password.
Step 2: Click on "Log in."
Expected Result: The user should be redirected to the dashboard page, displaying the "My Account" and "Logout" buttons.
Test Case 2: Viewing Order History
Step 1: Click on "My Account."
Step 2: Select "Order History."
Expected Result: The user should see a list of past orders with details like order number, date, and status.
Test Case 3: Checking Order Details
Step 1: Click on an order from the list.
Step 2: Verify that the details such as product name, price, and shipping address are displayed.
Expected Result: Order details should match the information provided during the purchase.
Functional testing focuses on validating the entire order process, ensuring that the user experience is seamless.
Best Practices for Functional Testing:
Understand Business Requirements: Always start by thoroughly understanding the requirements and user stories.
Use Automation Tools: Automate repetitive functional tests using tools like Selenium or Cucumber for efficient testing.
Create Detailed Test Cases: Write clear and detailed test cases that cover all possible user scenarios.
Test Complex Features First: Begin with high-risk and complex functionalities before moving on to simpler ones.
Regularly Update Test Cases: Keep test cases up-to-date as new features and updates are introduced.
What is Unit Testing?
Unit Testing involves testing individual components or units of a software application to ensure that each part works as expected. It is typically conducted by developers during the coding phase and focuses on the smallest testable parts of an application, such as functions, methods, and classes.
Key Aspects of Unit Testing:
Objective: To validate that each unit of the software code performs correctly.
Scope: Focuses on individual elements, like buttons, text boxes, or functions.
Developer’s Perspective: Written and executed by developers to ensure that each component performs as designed.
Code-Centric: Unit testing is highly code-focused, as it examines the correctness of individual functions.
Unit Testing Example:
Using the same e-commerce application example, here are some unit test cases:
Test Case 1: Username Input Text Box
Step 1: Verify that the "Username" field accepts input.
Step 2: Ensure it allows only alphanumeric characters.
Step 3: Verify that it does not accept special characters.
Expected Result: The field should accept usernames up to 15 characters long and reject invalid inputs.
Test Case 2: Password Validation
Step 1: Verify that the "Password" field accepts input.
Step 2: Ensure it requires at least one special character.
Step 3: Verify that passwords below 8 characters are rejected.
Expected Result: The field should accept passwords that meet the criteria and display an error for others.
Test Case 3: Submit Button Functionality
Step 1: Verify that clicking the "Submit" button initiates a validation process.
Step 2: Validate that the "Username" and "Password" fields are not empty.
Expected Result: If the fields are valid, the user is redirected to the homepage; otherwise, an error message is displayed.
Unit testing ensures that each function of the login page works independently before being integrated into the larger system.
Best Practices for Unit Testing:
Start Early: Begin unit testing during the development phase to catch issues early.
Automate Unit Tests: Use frameworks like JUnit (Java), PyTest (Python), or Jest (JavaScript) to automate unit tests.
Test One Functionality at a Time: Each unit test should focus on one specific function or method to ensure precision.
Write Reusable Tests: Write unit tests that can be reused across similar functions.
Maintain Independence: Ensure that each unit test is independent and does not rely on external databases or networks.
Difference Between Functional Testing and Unit Testing
Understanding the differences between functional testing and unit testing is crucial for applying each effectively during the testing process:
Aspect | Functional Testing | Unit Testing |
Scope | Tests entire user flows and features | Tests individual components or units |
Focus | Validates overall system functionality | Validates internal code logic |
Tested By | Typically performed by QA testers | Performed by developers |
Perspective | User-centered approach | Developer-centered approach |
Speed | Slower due to testing broader functionality | Faster as it targets specific functions |
Automation | Can be automated for large applications using frameworks | Typically automated using unit testing frameworks |
Examples | Testing a complete login process | Testing input validation of a login form |
Error Identification | Helps identify issues in the entire application’s functionality | Pinpoints specific code-level bugs |
When to Use Functional Testing vs Unit Testing?
Use Functional Testing:
When validating end-to-end scenarios like user log-in, data processing, and order management.
When ensuring that the software meets business requirements and user needs.
For acceptance testing and usability testing.
Use Unit Testing:
During the development phase to catch issues in individual functions or methods.
When checking that each component behaves as expected in isolation.
For identifying code-specific errors before integrating components into the system.
Conclusion
In summary, the difference between functional testing and unit testing lies in their focus, scope, and objectives. Functional testing ensures that an application behaves as expected from a user’s perspective, while unit testing focuses on verifying that individual components work correctly from a developer’s point of view. Both are indispensable in the testing process, providing unique advantages that, when combined, result in a high-quality software product.
Integrating both testing approaches into your workflow helps ensure a robust, bug-free application that delivers a seamless experience to users. Using unit tests to catch errors early and functional tests to validate the entire user journey will optimize the quality assurance process.
Key Takeaways:
Functional testing focuses on user experience and end-to-end flows, while unit testing targets specific code components.
Functional testing is slower and broader, while unit testing is faster and more focused.
Unit testing helps catch bugs early in the development phase, while functional testing ensures the final product meets user expectations.
Use functional testing for comprehensive validation and unit testing for in-depth code analysis.
Both testing types are essential for delivering reliable and high-quality software.
FAQs
1. What is the main purpose of unit testing?
The primary purpose of unit testing is to validate that individual components of software, such as functions or methods, work as intended.
2. Can functional testing replace unit testing?
No, functional testing and unit testing serve different purposes. Functional testing validates the overall application, while unit testing focuses on individual components.
3. Which one is more time-consuming: functional testing or unit testing?
Functional testing is generally more time-consuming because it involves testing the application as a whole, while unit testing is faster due to its focus on specific elements.
4. What tools are used for functional testing?
Popular tools for functional testing include Selenium, Cucumber, and QTP. These tools can automate end-to-end testing of user flows.
5. When should you perform unit testing?
Unit testing should be performed during the development phase to catch bugs in individual functions before integrating them into the larger application.
6. How is integration testing different from unit testing?
Integration testing focuses on testing how different modules interact, while unit testing verifies the functionality of individual components.
7. Can functional testing be automated?
Yes, functional testing can be automated using tools like Selenium and TestComplete to ensure efficiency, especially for regression testing.
8. Why is functional testing important?
Functional testing ensures that the software meets business requirements and delivers a seamless user experience, making it critical for software quality assurance.
Comments