Introduction
End-to-end (E2E) testing is an essential part of the software development lifecycle, particularly in agile methodologies. It involves testing the entire application from start to finish to ensure that all components and systems work together as intended. This comprehensive approach mimics real-world user scenarios, ensuring that the application behaves as expected in production. By integrating various testing types, such as GUI, database, and security testing, E2E testing helps identify issues early and enhances the overall user experience. In this guide, we will delve into the intricacies of E2E testing, its types, tools, and best practices to ensure robust and reliable software delivery.
What is End to End Testing?
End-to-end testing is a comprehensive validation method that tests an application’s workflow from start to finish. It verifies that each component functions correctly within the entire system in real-world scenarios, ensuring the software behaves as intended from the user’s perspective. E2E testing checks the system’s overall behavior against user expectations, identifying system-wide issues before user acceptance testing.
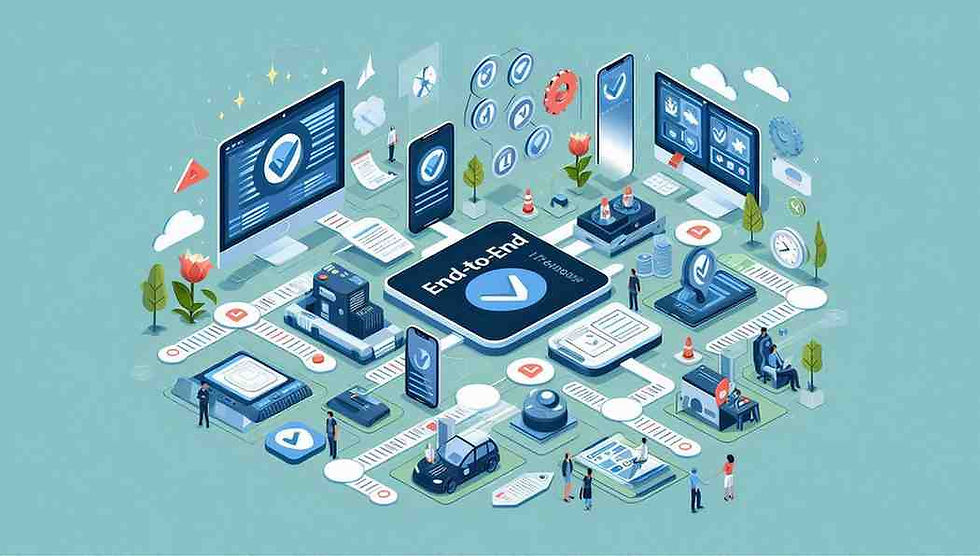
Importance of End to End Testing
End-to-end testing is crucial for several reasons:
Holistic Validation: Evaluates the entire application, ensuring all parts work together seamlessly.
User Experience: Verifies the system from a user’s perspective, enhancing overall user satisfaction.
Early Defect Detection: Identifies issues early, reducing the cost and effort of later fixes.
Performance Assurance: Validates performance under real-world conditions, ensuring the application can handle expected traffic and data loads.
Security Checks: Confirms that security protocols are effective across all parts of the application.
Reliability: Establishes confidence in the stability and reliability of the application before it goes live.
Compliance with Requirements: Ensures that the final product aligns with both technical and business requirements.
Types of End to End Testing
End-to-end testing encompasses various testing types to cover all aspects of the application:
Functional Testing
Functional testing verifies that each feature works according to the requirements. It focuses on the functionality of the software, ensuring that all functions are working as expected.
Performance Testing
Performance testing assesses the application’s responsiveness, stability, and scalability under load. It ensures that the software performs well under expected traffic conditions.
Usability Testing
Usability testing ensures the application is intuitive and user-friendly. It evaluates the ease with which users can navigate and interact with the application.
Security Testing
Security testing checks for vulnerabilities and ensures data protection measures are effective. It identifies security flaws that could be exploited by malicious users.
Compatibility Testing
Compatibility testing confirms that the application works across different devices, browsers, and operating systems. It ensures that users have a consistent experience regardless of their setup.
Disaster Recovery Testing
Disaster recovery testing assesses the application’s ability to recover from crashes, failures, or disasters. It ensures that the system can return to normal operations quickly after an issue.
Compliance Testing
Compliance testing ensures the application adheres to industry standards and regulations. It verifies that the software meets legal and regulatory requirements.
End to End Testing Tools
End-to-end testing tools are essential for automating the testing process, ensuring efficiency and accuracy. These tools can simulate user interactions, verify system integrations, and report on performance. Here are some widely used E2E testing tools:
Selenium
Selenium is a powerful tool for automating web browsers. It allows testers to write scripts in various programming languages and supports multiple browsers and operating systems.
Cypress
Cypress offers fast, easy, and reliable testing for anything that runs in a browser. It provides a comprehensive framework for end-to-end testing, including built-in support for assertions and debugging.
TestComplete
TestComplete provides a comprehensive set of features for web, mobile, and desktop testing. It supports a wide range of scripting languages and integrates with various CI/CD tools.
Robot Framework
Robot Framework is a generic test automation framework for acceptance testing and acceptance test-driven development (ATDD). It uses a keyword-driven approach, making it easy to create readable and maintainable test cases.
Protractor
Protractor is an end-to-end test framework for Angular and AngularJS applications. It supports automatic synchronization with Angular applications, making it ideal for testing dynamic web applications. Note: With Protractor development ending in 2022, it is crucial to explore Protractor alternatives to ensure continued support and updates.
How to Perform End to End Testing?
Performing end-to-end testing involves a series of steps to ensure that every aspect of the application is evaluated:
Requirement Analysis
Understand the application’s functionality and user flow. Identify the critical paths and user interactions that need to be tested.
Test Planning
Define the scope, objectives, and schedule of the testing process. Plan the resources, tools, and environments needed for testing.
Test Environment Setup
Create a testing environment that mimics the production setting. Ensure that the environment includes all necessary components, such as databases, servers, and external services.
Test Case Design
Develop test cases that cover all possible user scenarios. Include both positive and negative test cases to thoroughly evaluate the application.
Test Execution
Run the test cases, either manually or using automation tools. Monitor the execution and capture any defects or anomalies.
Result Analysis
Evaluate the test results to identify any defects or areas for improvement. Analyze the root cause of issues and prioritize them for resolution.
Reporting
Document the findings and share them with the development team for further action. Include detailed descriptions of defects, steps to reproduce, and severity.
End to End Testing on Real Devices
Performing end-to-end testing on real devices is crucial as it ensures that your software behaves as expected in real-world conditions, capturing device-specific issues and user interactions.
Prerequisites
Node.js: Install Node.js which includes npm (Node Package Manager).
Selenium WebDriver: Install the selenium-webdriver package.
Assert: Install the assert package for writing assertions.
BrowserStack Account: Sign up for BrowserStack and obtain your Automate Key.
Steps to Perform End-to-End Testing
Step 1: Setup a New Node.js Project
Initialize a new Node.js project and install the necessary packages.
Step 2: Add Test Configurations
Create a file named browserstack.yml at the root of your project with the following configuration:
yaml
userName: <your-user-name>
accessKey: <your-access-key>
platforms:
- os: Windows
osVersion: 10
browserName: Chrome
browserVersion: 120.0
- os: OS X
osVersion: Monterey
browserName: Safari
browserVersion: 15.6
- deviceName: iPhone 13
osVersion: 15
browserName: Chromium
deviceOrientation: portrait
parallelsPerPlatform: 1
browserstackLocal: true
buildName: browserstack-build-1
projectName: BrowserStack Sample
debug: false
networkLogs: false
consoleLogs: errors
Step 3: Write Test Script
Create a test script (test/sample_test.js):
javascript
const assert = require('assert');
const { Builder, By, Capabilities, until } = require("selenium-webdriver");
var buildDriver = function() {
return new Builder().
usingServer('http://localhost:4444/wd/hub').
withCapabilities(Capabilities.chrome()).
build();
};
describe('BStack\'s Cart Functionality', async function() {
this.timeout(0);
var driver;
before(function() {
driver = buildDriver();
});
it('should allow items to be added to the cart', async function() {
await driver.get('https://bstackdemo.com/');
await driver.wait(until.titleMatches(/StackDemo/i), 10000);
const productSelector = By.xpath('//*[@id="1"]/p');
const addToCartButtonSelector = By.xpath('//*[@id="1"]/div[4]');
const cartContentSelector = By.className("float-cart__content");
const productInCartSelector = By.xpath('//*[@id="__next"]/div/div/div[2]/div[2]/div[2]/div/div[3]/p[1]');
await driver.wait(until.elementLocated(productSelector));
const productText = await driver.findElement(productSelector).getText();
await driver.wait(until.elementLocated(addToCartButtonSelector));
await driver.findElement(addToCartButtonSelector).click();
await driver.wait(until.elementLocated(cartContentSelector));
await driver.wait(until.elementLocated(productInCartSelector));
const productCartText = await driver.findElement(productInCartSelector).getText();
assert.strictEqual(productText, productCartText, 'The product name should match the name in the cart.');
});
after(async function() {
await driver.quit();
});
});
Step 4: Run Test
Move inside the test folder and run the following command:
bash
npm run sample_test
Step 5: Review Results
Review the test results on the BrowserStack Automate dashboard.
Challenges in End to End Testing
End-to-end testing can be complex and demanding due to several factors:
Complex User Scenarios: Simulating real-world user interactions can be challenging.
Test Environment: Setting up a test environment that accurately reflects production can be difficult.
Data Management: Ensuring test data is consistent and reliable requires careful planning.
System Integrations: Testing the interactions between different systems and components can reveal unexpected issues.
Resource Allocation: E2E testing often requires significant time and resources to execute effectively.
Flakiness in Automation: Automated tests can sometimes be unreliable, requiring regular maintenance and updates.
Best Practices for End to End Testing
Adopting best practices in end-to-end testing is crucial for delivering a reliable and user-friendly application. These practices help streamline the testing process and ensure that the final product meets quality standards.
Design Comprehensive Test Cases
Create test cases that cover all functionalities and user scenarios. Include both positive and negative test cases to ensure thorough evaluation.
Use Automation Tools
Leverage automation tools to increase efficiency and coverage. Automate repetitive and time-consuming tests to save time and resources.
Maintain Relevance
Keep test cases current with application changes to maintain their relevance. Update tests regularly to reflect new features and changes in the application.
Focus on Critical Workflows
Prioritize testing the most important workflows that users are likely to perform. Ensure that these critical paths function correctly and deliver a seamless user experience.
Test with Realistic Data
Use data that closely mimics what will be used in production. This ensures that the tests are relevant and realistic, providing accurate results.
Collaborate with Stakeholders
Work closely with developers, business analysts, and users to understand requirements and expectations. This collaboration ensures that the tests align with business goals and user needs.
Regularly Review Test Results
Regularly review test results and update strategies as needed. Analyze defects and improve test cases to enhance the testing process.
Conclusion
End-to-end testing is a comprehensive approach that ensures an application behaves as expected in real-world scenarios, from start to finish. It is crucial for verifying the integrity of application workflows and enhancing user experience. By rigorously testing all components and user flows, end-to-end testing helps teams deliver robust and user-centric software products.
Testing on real devices and browsers ensures that you can check end-to-end user flows in your application from the user’s perspective. Real device clouds like BrowserStack Automate allow you to test under real user conditions, helping you find issues before making your application live.
BrowserStack Automate integrates well with all popular frameworks like Selenium, Cypress, Playwright, Puppeteer, NightwatchJS, and CI/CD tools like Jenkins, CircleCI, Azure, and many more to deliver a seamless testing experience with rich artifacts and enhanced debugging capabilities.
Key Takeaways
End-to-end testing ensures software integrity and user satisfaction.
It involves various types of testing, including functional, performance, and security testing.
Automation tools enhance efficiency and coverage in end-to-end testing.
Testing on real devices and browsers ensures accurate and realistic results.
Adopting best practices improves the effectiveness of end-to-end testing.
FAQs
What is end-to-end testing?
End-to-end testing is a comprehensive validation method that tests an application’s workflow from start to finish, ensuring that each component functions correctly within the entire system.
Why is end-to-end testing important?
End-to-end testing is important because it ensures that all components of an application interact correctly and that the system as a whole meets design and user requirements.
What are the types of end-to-end testing?
The main types of end-to-end testing include functional testing, performance testing, usability testing, security testing, compatibility testing, disaster recovery testing, and compliance testing.
What tools are used for end-to-end testing?
Popular end-to-end testing tools include Selenium, Cypress, TestComplete, Robot Framework, and Protractor.
How do you perform end-to-end testing?
Performing end-to-end testing involves requirement analysis, test planning, test environment setup, test case design, test execution, result analysis, and reporting.
What are the challenges in end-to-end testing?
Challenges in end-to-end testing include complex user scenarios, test environment setup, data management, system integrations, resource allocation, and flakiness in automation.
What are the best practices for end-to-end testing?
Best practices for end-to-end testing include designing comprehensive test cases, using automation tools, maintaining test case relevance, focusing on critical workflows, testing with realistic data, collaborating with stakeholders, and regularly reviewing test results.
Why should end-to-end testing be done on real devices?
End-to-end testing on real devices ensures that the software behaves as expected in real-world conditions, capturing device-specific issues and user interactions.
Comments