Introduction
Go, often referred to as Golang, is a popular programming language known for its simplicity, efficiency, and strong support for concurrency. However, until the release of Go 1.18, it lacked one significant feature that many developers desired—generics. With Go 1.18, generics have finally made their way into the language, offering developers a powerful tool to write more reusable and type-safe code.
Generics in Golang allow developers to write functions, data structures, and types that work with any data type. This flexibility not only reduces code duplication but also improves type safety, leading to more robust and maintainable software. However, the implementation of generics in Go is unique and comes with its own set of performance considerations that every developer should be aware of.
In this comprehensive guide, we will dive deep into generics in Golang, exploring how they are implemented, their benefits, potential pitfalls, and best practices to get the most out of this new feature.
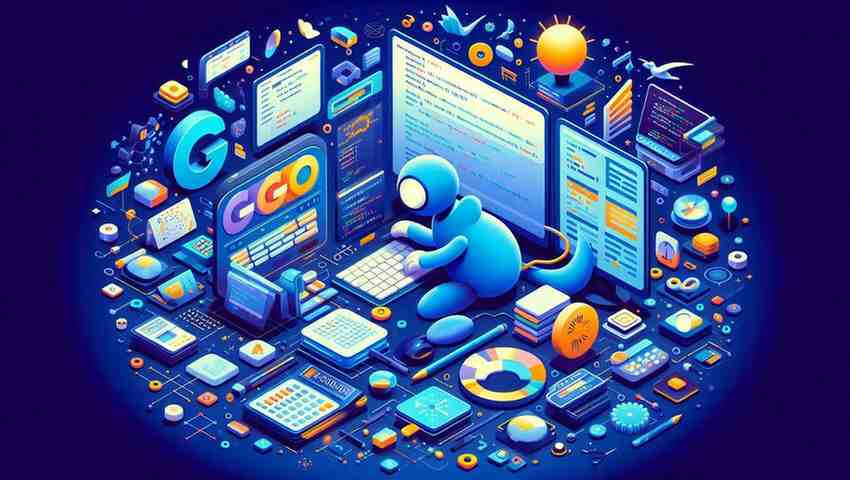
1. What Are Generics in Golang?
Generics in Golang are a way to write code that works with any data type. Instead of writing multiple versions of a function or data structure for different types, you can write one generic version that works for all types. This is achieved by defining type parameters that can represent any type, and these type parameters are specified when the generic code is invoked.
For example, with generics, you can write a single function to sort slices of any type, rather than writing separate functions for slices of int, float64, or string.
Example of Generics in Go
go
package main
import "fmt"
func PrintSlice[T any](s []T) {
for _, v := range s {
fmt.Println(v)
}
}
func main() {
ints := []int{1, 2, 3}
strings := []string{"Go", "Generics", "Are", "Here"}
PrintSlice(ints)
PrintSlice(strings)
}
In this example, PrintSlice is a generic function that works with any slice type, as denoted by the T type parameter.
2. The Need for Generics in Go
Before Go 1.18, developers often used interfaces to achieve a form of polymorphism in Go. However, interfaces come with some downsides, such as runtime overhead due to dynamic dispatch and loss of type safety. Additionally, without generics, developers had to resort to code duplication or reflection to handle multiple data types, leading to less maintainable code.
Generics address these issues by allowing developers to write functions and data structures that are both reusable and type-safe. This reduces the amount of boilerplate code and eliminates the need for type assertions or conversions, leading to more concise and reliable code.
Key Benefits of Generics in Go
Code Reusability: Write once, use anywhere with any data type.
Type Safety: Catch errors at compile-time rather than runtime.
Reduced Boilerplate: Eliminate the need for repetitive code.
Performance: Potential for performance improvements by avoiding dynamic dispatch.
3. How Go 1.18 Introduced Generics
The introduction of generics in Go 1.18 was a monumental change, as it required a significant redesign of the Go compiler and runtime. The Go team approached this carefully, ensuring that the addition of generics would not compromise Go’s core principles of simplicity, efficiency, and ease of use.
Go 1.18 introduced two new keywords, type and any, to support generics. The type keyword is used to define type parameters, while any is a predeclared identifier representing the interface{} type, making it easier to define generic functions that can accept any type.
Basic Syntax of Generics in Go
go
func Foo[T any](param T) T {
return param
}
In this example, T is a type parameter that can represent any type, allowing Foo to accept and return values of any type.
Constraints on Type Parameters
Go also allows you to constrain type parameters, ensuring that they satisfy certain conditions, such as implementing a specific interface or being one of several types. This adds more flexibility and power to generics.
go
func Min[T constraints.Ordered](a, b T) T {
if a < b {
return a
}
return b
}
In this example, constraints.Ordered is a constraint that ensures T can be compared using the < operator.
4. Understanding Parametric Polymorphism in Go
Generics in Golang are a form of parametric polymorphism, where the same code can be used with different types. This concept is implemented in various ways in different programming languages. The two most common approaches are monomorphisation and boxing.
Monomorphisation
Monomorphisation involves generating a separate version of the generic code for each type that it is used with. This approach is used by languages like C++ and Rust, and it often results in faster runtime performance because the code is fully specialized for each type. However, it can lead to larger binaries and longer compile times due to code duplication.
Boxing
Boxing involves treating all values as references to a generic "box" that can hold any type. This is how interfaces work in Go. Boxing leads to smaller binaries and faster compile times, but it can introduce runtime overhead due to the need for type checks and dynamic dispatch.
5. Go’s Implementation of Generics: Stenciling and Dictionaries
Go’s implementation of generics is a hybrid approach that combines elements of both monomorphisation and boxing. This is achieved using GCshape stenciling and dictionaries.
GCshape Stenciling
GCshape stenciling is a technique where Go generates different versions of a function or data structure based on the "shape" of the types involved. A type's shape is determined by how it is represented in memory and how it interacts with Go’s garbage collector. Types with the same shape share the same code, while types with different shapes generate separate versions.
For example, int and string have different shapes, so Go generates separate versions of a generic function for each. However, pointer types like int and string share the same shape, so they use the same code.
Dictionary-Based Method Resolution
When Go encounters a generic function, it passes a dictionary to the function at runtime. This dictionary contains metadata about the type parameters, including how to resolve method calls. This is necessary for cases where a type parameter is constrained by an interface, and Go needs to determine the correct method to call.
The dictionary-based approach allows Go to maintain fast compile times and small binaries, but it can introduce some runtime overhead, especially in cases where interface types are passed as type parameters.
6. Performance Implications of Using Generics in Go
One of the main concerns with generics is their potential impact on performance. While generics can improve performance by eliminating the need for dynamic dispatch through interfaces, they can also introduce new overhead due to dictionary lookups and method resolution.
Benchmarking Generic vs. Non-Generic Code
Let’s compare the performance of a generic function with a traditional interface-based function.
go
package main
import "io"
type DummyWriter struct {
buf []byte
}
func (d *DummyWriter) Write(data []byte) (int, error) {
d.buf = append(d.buf, data...)
return len(data), nil
}
func FooIFace(w io.Writer, data byte) {
w.Write([]byte{data})
}
func FooGeneric[T io.Writer](w T, data byte) {
w.Write([]byte{data})
}
func main() {
w := &DummyWriter{}
FooIFace(w, 42)
FooGeneric(w, 42)
}
In benchmarking, FooIFace (the interface-based function) performs faster than FooGeneric (the generic function). The reason is that the generic function involves additional steps to resolve the type and method using the dictionary, whereas the interface-based function benefits from Go’s inlining optimizations.
Inlining and Its Impact on Performance
Inlining is a powerful optimization where the compiler inserts the body of a function directly at the call site, avoiding the overhead of a function call. Go can inline functions that use interfaces, but at the time of writing, it cannot inline generic functions due to the way they are implemented. This can result in slower performance for generic functions in some cases.
7. Best Practices for Using Generics in Golang
To get the most out of generics in Go, it’s essential to follow best practices that maximize their benefits while avoiding potential pitfalls.
When to Use Generics
Reusable Code: Use generics when you need to write reusable code that works across multiple types.
Type Safety: Use generics to enforce type safety and avoid the need for type assertions or conversions.
Data Structures: Generics are particularly well-suited for implementing data structures like stacks, queues, and linked lists that work with any type.
Avoiding Performance Pitfalls
Be Cautious with Interfaces: Avoid passing interfaces as type parameters to generic functions unless necessary, as this can introduce additional overhead.
Benchmark Critical Code: Always benchmark your code when using generics to ensure that performance is not negatively impacted.
Leverage Constraints: Use constraints to guide the compiler in generating optimized code for specific types.
8. Common Use Cases for Generics in Go
Generics open up a wide range of possibilities in Go, allowing developers to write more flexible and efficient code. Here are some common use cases:
Generic Data Structures
Data structures like linked lists, binary trees, and hash maps are excellent candidates for generics. With generics, you can implement these data structures once and use them with any type.
go
package main
type Node[T any] struct {
Value T
Next *Node[T]
}
func NewNode[T any](value T) *Node[T] {
return &Node[T]{Value: value}
}
Generic Algorithms
Algorithms that work with different types, such as sorting, searching, or filtering, can also benefit from generics. By defining these algorithms generically, you can apply them to any data type without code duplication.
go
package main
func Filter[T any](s []T, fn func(T) bool) []T {
var result []T
for _, v := range s {
if fn(v) {
result = append(result, v)
}
}
return result
}
9. Challenges and Limitations of Generics in Golang
While generics bring many benefits, they also come with some challenges and limitations:
Complexity: Generics can make code more complex and harder to read, especially for developers unfamiliar with the concept.
Performance Overhead: The dictionary-based method resolution can introduce runtime overhead, particularly when interfaces are involved.
Limited Inlining: Generic functions are not currently eligible for inlining, which can result in slower performance compared to non-generic code.
10. The Future of Generics in Go
The introduction of generics in Go 1.18 is just the beginning. As the Go community gains more experience with generics, we can expect further improvements and optimizations. The Go team is committed to refining the generics implementation, and future releases may address some of the current limitations, such as inlining and performance optimizations.
11. Conclusion
Generics in Golang are a powerful addition that significantly enhances the language’s ability to write reusable and type-safe code. While there are some performance considerations to be aware of, the benefits of generics—such as reducing code duplication, improving type safety, and enabling more flexible APIs—make them a valuable tool for any Go developer.
By understanding how Go implements generics and following best practices, you can leverage this feature to write more robust and efficient software. Whether you’re building data structures, algorithms, or APIs, generics provide a versatile and elegant solution that will serve you well in many scenarios.
12. Key Takeaways
Generics in Go allow for type-safe, reusable code that works with any data type.
Monomorphisation and Boxing are the two primary strategies for implementing generics, and Go combines elements of both.
Go uses GCshape stenciling and dictionaries for efficient generics implementation.
Performance can vary when using generics; be mindful of potential overhead due to dictionary-based method resolution.
Best practices include benchmarking, avoiding unnecessary use of interfaces in generics, and leveraging type constraints.
13. FAQs
1. What are generics in Golang?
Generics in Golang are a way to write functions, types, and data structures that can work with any data type, providing more flexibility and reducing code duplication.
2. How do generics improve code reusability in Go?
Generics allow developers to write a single, reusable piece of code that can work with multiple types, eliminating the need for redundant implementations.
3. Are there any performance drawbacks to using generics in Go?
Yes, the dictionary-based method resolution used in Go’s generics implementation can introduce some runtime overhead, especially when interfaces are involved.
4. Can generic functions be inlined in Go?
As of Go 1.18, generic functions cannot be inlined, which can result in slower performance compared to non-generic functions.
5. When should I use generics in my Go code?
Use generics when you need to write reusable, type-safe code that works across multiple data types, such as in data structures or algorithms.
6. How do Go generics differ from generics in other languages like C++ or Java?
Go’s generics implementation is unique, combining elements of monomorphisation and boxing, with a focus on maintaining the simplicity and efficiency of the language.
Comments