As mobile applications continue to dominate the tech landscape, ensuring the quality of Android apps has never been more crucial. With millions of users accessing apps daily on various devices, automated testing has become a key practice in app development and quality assurance. In the world of Android app testing, automation frameworks allow development teams to run comprehensive tests efficiently and effectively, ensuring that applications work seamlessly across different devices and Android versions.
In this in-depth guide, we’ll explore the leading Android automated testing frameworks, explain how they work, provide sample code, and help you choose the right one based on your project requirements. Whether you're testing native, hybrid, or web apps, automating Android app testing is a surefire way to boost productivity, improve app quality, and reduce manual errors.
Introduction to Android Automated Testing
Automated testing is the backbone of quality assurance in the mobile app development process. It allows developers to test their applications on multiple devices and platforms without manually running each test, significantly speeding up the development cycle. For Android apps, automated testing tools are crucial for ensuring that apps perform consistently across a wide range of devices, OS versions, and configurations.
Given the variety of Android devices and the frequent updates in operating systems, it's essential to automate testing on real devices as much as possible. This ensures that any issues with performance, UI, or functionality are identified and corrected early in the development process. In this guide, we focus on the most widely used Android testing frameworks and how they can enhance your automated testing strategy.
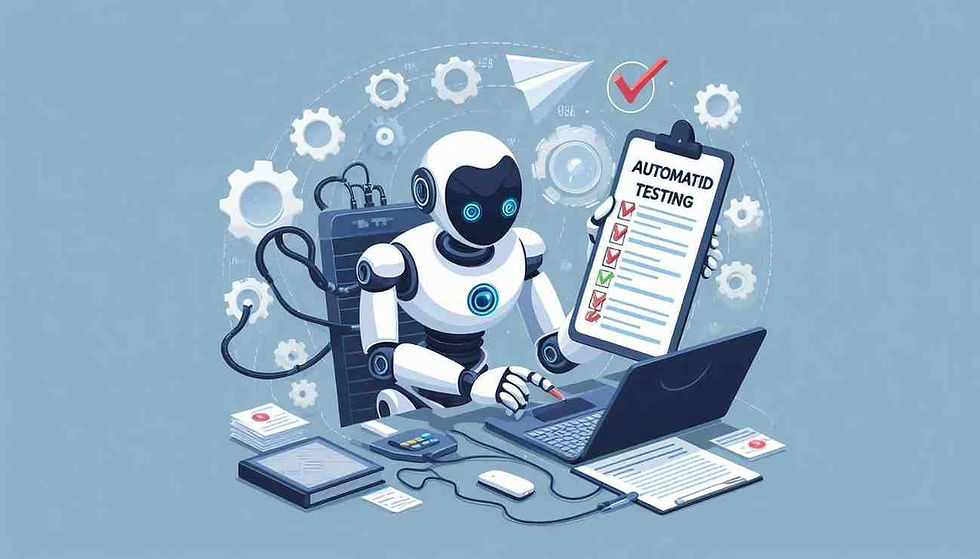
Why Automated Testing is Essential for Android Apps
1. Device Fragmentation
Android operates on a wide range of devices, each with its own screen size, resolution, and hardware configurations. Manually testing on each device is not feasible. Automated testing allows you to cover multiple devices at once.
2. Fast Feedback
Automated tests can run continuously in a CI/CD pipeline, providing immediate feedback to developers after code commits. This reduces the time it takes to find and fix bugs.
3. Repeatability
Automated tests can be run repeatedly without the risk of human error. This makes it easier to identify regressions and ensures consistent results.
4. Reduced Manual Effort
Running repetitive tests manually is time-consuming and prone to errors. Automated tests can handle these tasks more efficiently, freeing up testers to focus on more complex scenarios.
5. Cost Efficiency
While setting up automated tests requires an initial investment, it pays off in the long run by reducing the need for manual testing and shortening release cycles.
Top 5 Android Automated Testing Frameworks
In this section, we’ll break down the top five Android automated testing frameworks, their pros and cons, and provide code examples to illustrate how they work.
1. Appium: The Cross-Platform King
Overview:Appium is one of the most popular open-source tools for automating Android (and iOS) apps. It allows developers to test native, hybrid, and mobile web apps across multiple platforms, including Android and iOS, without needing to modify the app's code.
Key Features:
Cross-platform support: Works with both Android and iOS.
Supports multiple programming languages: Java, Python, Ruby, JavaScript, C#, etc.
Integrates with Selenium: Uses WebDriver, making it familiar to Selenium users.
Appium Code Example:
python
# Wait for hello
sleep(3)
textFields = driver.find_elements_by_tag_name('textField')
assertEqual(textFields[0].get_attribute("value"), "Hello")
# Click sign-in button
driver.find_elements_by_name('Sign in')[0].click()
# Enter username and password
textFields = driver.find_elements_by_tag_name('textField')
textFields[0].send_keys("twitter_username")
textFields[1].send_keys("passw0rd")
# Click the Login button
driver.find_elements_by_tag_name('button')[0].click()
# Exit
driver.quit()
Advantages:
Works with various programming languages.
Ideal for testing across Android and iOS.
Easy integration for those familiar with Selenium.
Drawbacks:
Slower than some other frameworks, especially for large-scale test executions.
Can be complex to set up for beginners.
2. Detox: Fast Execution with React Native Support
Overview:Detox is a JavaScript testing framework designed for React Native applications. It integrates directly into the application and provides fast, reliable test execution.
Key Features:
Built for React Native.
Fast execution: Tests are triggered directly within the app.
Great documentation for easy adoption.
Detox Code Example:
javascript
describe('HelloDetoxTest', () => {
beforeEach(async () => {
await device.reloadReactNative();
});
it('should have welcome screen', async () => {
await expect(element(by.id('welcome'))).toBeVisible();
});
it('should show hello React after tap', async () => {
await element(by.id('hello_react')).tap();
await expect(element(by.text('React!!!'))).toBeVisible();
});
});
Advantages:
Faster execution compared to Appium.
Built-in support for React Native apps.
Simple to pick up for JavaScript developers.
Drawbacks:
Not yet supported on real iOS devices (Android only).
Limited to React Native apps.
3. Espresso: Google’s Reliable UI Testing Tool
Overview:Espresso is a native Android testing framework developed by Google. It's widely used for UI testing and is known for its fast execution and reliable results, as tests run directly on the app's main thread.
Key Features:
Developed and supported by Google.
Fast and reliable UI testing.
Synchronized with the UI thread to avoid flakiness.
Espresso Code Example:
java
public void testEspresso() {
// Check if a view with the text 'Hello' is displayed
onView(withText("Hello")).check(matches(isDisplayed()));
// Perform click on 'Sign in' button
onView(withId(R.id.sign_in)).perform(click());
// Enter username and password
onView(withId(R.id.login_username)).perform(typeText("username"));
onView(withId(R.id.login_password)).perform(typeText("password"));
// Click log in
onView(withId(R.id.login_login)).perform(click());
}
Advantages:
Fast execution with minimal test flakiness.
Works well for Android UI tests.
Synchronizes automatically with the app's main thread.
Drawbacks:
Limited to Android; no cross-platform support.
Not suitable for webviews or hybrid apps.
4. Calabash: Non-Technical User Friendly
Overview:Calabash is an open-source framework for both Android and iOS that allows non-technical users to write and execute automated tests. It uses Cucumber, making it accessible to non-developers by allowing tests to be written in plain language.
Key Features:
Cross-platform support for Android and iOS.
Non-technical user-friendly: Write tests in Cucumber.
Supports natural language commands for ease of use.
Calabash Code Example:
ruby
Feature: Login feature
Scenario: As a valid user, I can log into my app
Given I wait for text "Hello"
Then I press view with id "Sign in"
Then I enter text "username" into "login_username"
Then I enter text "password" into "login_password"
Then I wait for activity "HomeTabActivity"
Advantages:
Suitable for non-technical users.
Simple test scripts using natural language.
Supports both Android and iOS.
Drawbacks:
Slower than frameworks like Espresso or Detox.
Requires more configuration compared to native tools.
5. UI Automator: For Native UI Testing
Overview:UI Automator is a Google-developed framework for testing the UI of Android apps. It allows developers to create automated UI tests that can span multiple apps and perform interactions across processes.
Key Features:
Developed by Google and integrated with Android Studio.
Capable of testing across multiple apps.
Supports JUnit for writing test cases.
UI Automator Code Example:
java
public void testSignInAndTweet() throws Exception {
// Press Home button
getUiDevice().pressHome();
// Launch Twitter app
new UiObject(new UiSelector().text("Twitter")).click();
// Perform login
new UiObject(new UiSelector().text("Sign In")).click();
new UiObject(new UiSelector().className("android.widget.EditText").instance(0)).setText("u
ername");
new UiObject(new UiSelector().className("android.widget.EditText").instance(1)).setText("password");
new UiObject(new UiSelector().className("android.widget.Button").text("Sign In")).click();
}
Advantages:
Supports testing across multiple apps and processes.
Runs on devices with API level 16 or higher.
Provides multiple UI components for easy interaction.
Drawbacks:
Does not support webviews.
Limited to Android apps.
How to Choose the Right Android Automated Testing Tool
Choosing the right automated testing framework depends on several factors, including the type of app you're developing, the platforms you're targeting, and your team’s skillset. Here are a few questions to help guide your decision:
Cross-Platform Needs: Are you testing on both Android and iOS? If so, frameworks like Appium or Calabash are best.
Speed: If you need fast test execution, Espresso and Detox are better options.
Non-Technical Users: If your team includes non-developers, Calabash’s Cucumber-based syntax might be a good fit.
UI Testing: For native UI testing on Android, Google’s own Espresso or UI Automator is ideal.
Best Practices for Android Automated Testing
Test on Real Devices: Use cloud-based testing platforms like BrowserStack or Firebase Test Lab to run tests on real devices, ensuring greater reliability.
Parallel Execution: Run your tests in parallel to reduce test execution time and get faster feedback.
Automate CI/CD: Integrate your tests into a CI/CD pipeline to ensure continuous testing with every code commit.
Mock External Dependencies: Use mock data or services to reduce flakiness and ensure tests run consistently.
Conclusion
Automating Android app testing is essential for ensuring a consistent, high-quality user experience across devices and operating systems. Whether you're developing native, hybrid, or web-based apps, selecting the right testing framework can drastically reduce your development cycle time and improve your app's stability. By understanding the strengths and weaknesses of each framework—whether it’s Appium, Detox, Espresso, Calabash, or UI Automator—you can choose the best tool for your needs and ensure smooth, efficient test automation for your Android projects.
FAQs
1. What is Android automated testing?
Android automated testing involves using specialized tools and frameworks to run tests on Android applications without manual intervention. It helps ensure app functionality across different devices and OS versions.
2. Which framework is best for Android automated testing?
The best framework depends on your project needs. Appium is great for cross-platform testing, while Espresso is excellent for Android UI testing. Calabash is a good choice for non-technical users.
3. What is the difference between Appium and Espresso?
Appium is cross-platform and supports testing both Android and iOS apps. Espresso is a native Android framework designed for fast, reliable UI testing.
4. Can I automate Android testing on real devices?
Yes, you can use cloud-based services like BrowserStack, Firebase Test Lab, or AWS Device Farm to run tests on real devices.
5. Is Calabash still relevant for Android testing?
Yes, Calabash is still relevant, especially for teams that need non-technical members to write and execute automated tests using Cucumber.
6. How do I integrate automated tests into a CI/CD pipeline?
You can integrate automated tests into a CI/CD pipeline using tools like Jenkins, CircleCI, or GitLab CI, which will automatically trigger tests with every code commit.
7. What is the primary advantage of Detox over Appium?
Detox is faster and more efficient for testing React Native applications, as it integrates directly with the app and doesn’t require external orchestration like Appium.
8. Can Espresso be used for testing hybrid apps?
Espresso is primarily designed for native Android apps and does not support testing reviews, making it less suitable for hybrid apps.
Key Takeaways
Automated testing is essential for Android apps to ensure they function well across multiple devices and OS versions.
Appium is best for cross-platform testing, while Espresso offers fast, reliable UI testing for Android.
Detox is an excellent choice for React Native apps due to its fast execution.
Calabash is ideal for non-technical teams needing simple, natural language-based test cases.
Real-device testing on platforms like BrowserStack ensures better test reliability and results.
Comments