API headers play a pivotal role in how client-server communication occurs, defining how requests are processed and responses are formatted. In today’s interconnected digital world, API headers serve as crucial components that provide metadata for every request sent between systems. Understanding how to effectively use API headers is vital for any developer working with APIs, as headers ensure smooth data exchanges, proper authentication, caching management, and much more.
Whether you're new to APIs or looking to enhance your integration skills, this guide covers all aspects of API headers. From understanding what they are, how they work, and their technical importance, to practical examples and best practices for building robust and secure API requests, we’ve got it all covered.
What Are API Headers?
API headers are key-value pairs included in API requests and responses that carry metadata, offering additional information about the request or the response itself. Headers provide context and instructions that help define how data is transferred between systems. For example, headers can indicate the format of the data being sent, validate whether the requester has access, or control how data is cached.
Headers are part of the HTTP protocol, which governs how information is sent between the client (requester) and the server (responder). Whether you’re making a GET request to retrieve data or sending a POST request to submit data, headers are essential for smooth operation.
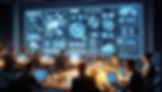
Why Are API Headers Important?
While the body of an API request carries the actual data, the headers provide important context that guides the server on how to handle the data. Without properly configured headers, requests may fail, data may not be returned in the correct format, or authentication may be rejected.
Here’s why API headers are crucial:
Authorization: API headers help manage security and authorization by containing credentials like API keys or OAuth tokens.
Content Negotiation: They ensure that the server responds in a format that the client expects (e.g., JSON or XML).
Performance Optimization: Headers manage caching and reduce the need for repeated requests to the server.
Error Handling: HTTP status codes returned in headers inform the client if the request was successful or if errors occurred.
Headers are a fundamental part of API communication, ensuring that the interaction between the client and server is smooth, efficient, and secure.
Key Components of API Headers
API headers can be classified into several broad categories depending on the role they play in the communication process. Understanding these categories is important for constructing and interpreting API calls correctly.
1. Body and Response Information
One of the primary uses of headers is to control the format of the request or response body. This ensures that both the client and server agree on how data is exchanged.
Accept: This header tells the server which formats are acceptable in the response. Common options include application/json or application/xml. For example, if your application expects a JSON response, you would use:
bash
Accept: application/json
Content-Type: This header indicates the type of data being sent in the request body, such as application/json, application/xml, or text/html. It’s crucial for servers to know how to interpret the incoming data:
bash
Content-Type: application/json
Accept-Charset: This header specifies the character encoding that the client can handle. This is important when dealing with text-based data:
makefile
Accept-Charset: utf-8
For responses, headers like Status are essential for determining the outcome of the request. Status codes like 200 OK signal a successful response, while 404 Not Found indicates that the requested resource is not available.
2. Authorization and Security
Security is one of the most important functions of API headers. They often carry credentials that allow the client to authenticate with the server.
Authorization: This header carries authentication credentials, such as API keys or OAuth tokens, that prove the client has permission to access certain resources:
makefile
Authorization: Bearer YOUR_API_KEY
WWW-Authenticate: This header is included in server responses when the client needs to provide credentials before gaining access to the resource.
Authorization headers ensure secure communication by validating the identity of the client before allowing access to sensitive data or actions.
3. Response Caching
To optimize performance and reduce server load, caching headers are used. Caching allows clients to reuse a previous response without contacting the server again, reducing the number of requests made.
Cache-Control: This header dictates how responses should be cached. It includes directives like public, private, no-cache, and max-age. For instance, you can specify that a response should not be cached:
yaml
Cache-Control: no-cache
Expires: This header specifies the time after which the response is considered stale and must be refreshed from the server:
yaml
Expires: Wed, 01 Oct 2023 00:00:00 GMT
Caching headers can improve the efficiency of an application by reducing redundant data requests and speeding up response times.
4. Cookies for Session Management
Cookies are small pieces of data sent by the server to the client, which the client stores and sends back with future requests. This is especially useful in web applications where session management is required.
Set-Cookie: This header is used by the server to send a cookie to the client. The cookie is then stored by the client and included in future requests to maintain the state:
yaml
Set-Cookie: sessionId=abc123; Expires=Wed, 01 Oct 2023 00:00:00 GMT
Cookie: This header is included in requests from the client, containing cookies previously sent by the server. This allows the server to identify the client and maintain session consistency:
makefile
Cookie: sessionId=abc123
Cookies are essential for managing user sessions, tracking interactions, and providing personalized experiences in web applications.
Real-World Examples of API Headers
Let’s take a look at a couple of real-world examples to better understand how API headers are used in practice.
Example 1: A GET Request with Authorization and Content-Type
Imagine you're sending a GET request to a weather API to retrieve the current weather for a specific city. You need to include an API key for authorization and specify that you expect the response in JSON format.
Request:
vbnet
GET /current-weather?city=London HTTP/1.1
Host: api.weather.com
Authorization: Bearer YOUR_API_KEY
Accept: application/json
Response:
css
HTTP/1.1 200 OK
Content-Type: application/json
{
"city": "London",
"temperature": "15°C",
"condition": "Cloudy"
}
In this example, the Authorization header ensures that only authenticated users can access the API, and the Accept header specifies that the response should be in JSON format.
Example 2: A POST Request with Custom Headers
Now let’s say you are sending a POST request to an API that manages a database of users. You need to send the data in JSON format, specify the content type, and prevent the response from being cached.
Request:
makefile
POST /users HTTP/1.1
Host: api.example.com
Authorization: Bearer YOUR_API_KEY
Content-Type: application/json
Cache-Control: no-store
{
"name": "John Doe",
"email": "john.doe@example.com"
}
Response:
css
HTTP/1.1 201 Created
Content-Type: application/json
Cache-Control: no-store
{
"id": "12345",
"name": "John Doe",
"email": "john.doe@example.com"
}
Here, the Content-Type header indicates that the data being sent is in JSON format, and the Cache-Control header ensures that the response is not stored.
How to Use API Headers in Practice
Working with API headers is easy once you understand their function. Here's a step-by-step guide on how to effectively use headers when making API calls.
1. Check the API Documentation
API documentation provides all the necessary details about what headers are required for each endpoint. Always refer to the documentation to understand what headers you need to include and in what format.
2. Include Authorization Credentials
Most APIs require some form of authentication. Ensure that the Authorization header is included and that you have the correct credentials, such as an API key or OAuth token.
3. Specify the Expected Response Format
To ensure that you receive data in the format you can process, include headers like Accept and Content-Type. If you want the response in JSON, specify:
bash
Accept: application/json
Content-Type: application/json
4. Manage Caching Behavior
Use caching headers like Cache-Control to control how your application handles data caching. For example, if you want to prevent caching of sensitive information:
yaml
Cache-Control: no-store
5. Monitor HTTP Status Codes
Always check the status code in the response headers to confirm if your request was successful. A 200 OK status indicates success, while a 404 Not Found or 401 Unauthorized indicates an issue that needs attention.
Best Practices for API Headers
To ensure the efficient and secure use of API headers, follow these best practices:
Keep It Secure: Always use HTTPS to encrypt API traffic. Include sensitive information like API keys in the headers, not in the URL.
Be Clear About Content Type: Explicitly specify the content type in both requests and responses to avoid format mismatches.
Use Cache-Control Wisely: Control caching to optimize performance while ensuring that outdated data is not used.
Minimize Header Size: Large headers can slow down requests and responses. Only include necessary metadata.
Log and Monitor: Track header data for debugging and to ensure proper authentication and caching behavior.
Conclusion
API headers are an essential aspect of web development, ensuring secure, efficient, and organized communication between client and server applications. From specifying content formats and managing authorization to handling caching and session data, headers provide crucial metadata that makes API interactions possible.
Understanding how to effectively use and implement API headers can significantly enhance the performance and security of your API integrations. By following best practices and leveraging headers correctly, you can ensure smooth data exchanges and reliable application performance.
Key Takeaways
API headers are used to provide metadata for requests and responses, guiding server-client communication.
They play crucial roles in authorization, content negotiation, caching, and session management.
Headers like Accept, Authorization, and Cache-Control are vital for controlling data formats, security, and performance.
Proper use of HTTP status codes in headers helps developers handle errors and track the success of API calls.
Always follow API documentation for header requirements and best practices for optimal integration.
Frequently Asked Questions (FAQs)
1. What is the purpose of the Content-Type header in API calls?
The Content-Type header specifies the format of the data being sent in the request body, such as application/json or application/xml. It helps the server process the data correctly.
2. What does the Authorization header do in an API request?
The Authorization header is used to send credentials (like API keys or OAuth tokens) that authenticate the client and prove that it has permission to access the requested resource.
3. How does caching work with API headers?
Caching is controlled by headers like Cache-Control and Expires, which instruct the server and client whether to store a response and for how long. This can improve performance by reducing repeated requests.
4. What are cookies in API headers used for?
Cookies in API headers (via Set-Cookie and Cookie headers) are used to store small pieces of data that help manage user sessions, personalize experiences, or track user interactions.
5. Can I include multiple headers in an API request?
Yes, API requests often include multiple headers, each serving a different function, such as specifying content type, handling authentication, and controlling caching.
6. How do I secure API headers?
Ensure that sensitive data like API keys are sent via headers and always use HTTPS to encrypt API traffic. Rotate credentials regularly and monitor requests for suspicious activity.
7. What is the role of status codes in API headers?
Status codes (like 200 OK, 404 Not Found, or 500 Internal Server Error) indicate the success or failure of an API request and help the client understand how to handle the response.
8. How can I view the headers of an API request or response?
You can view headers using API testing tools like Postman or directly from the developer tools in web browsers under the "Network" tab.