Guide to GoTest: Testing Go Applications Effectively
- Gunashree RS
- Oct 23, 2024
- 7 min read
In the world of modern software development, rigorous and efficient testing has become a cornerstone of reliable, maintainable code. As Go (Golang) continues to gain popularity, its built-in testing framework—GoTest—has proven to be a powerful tool for ensuring the quality of Go applications. Whether you are building a microservice, command-line tool, or a full-scale web application, writing automated tests is essential for delivering high-quality software.
In this comprehensive guide, we will walk through the GoTest tool, including its features, how to use it, and best practices for writing tests in Go. We’ll also explore strategies for testing command-line interfaces (CLIs) and dive into advanced testing tools like testscript for more complex Go-based projects.
What Is GoTest?
GoTest is Go’s built-in testing tool, designed to make writing and running tests in Go applications simple and straightforward. It provides developers with everything needed to test their code, check for errors, and ensure their software behaves as expected.
At its core, GoTest runs the tests you write within your Go codebase. It follows a convention where test files have the _test.go suffix and include functions that begin with Test, Benchmark, or Example. These tests can be run using the go test command.
GoTest supports the following test types:
Unit Tests: To verify individual functions or methods.
Integration Tests: To test how different components work together.
Benchmark Tests: To measure the performance of your code.
Example Tests: Embedded examples in your code that serve as both documentation and testable code snippets.
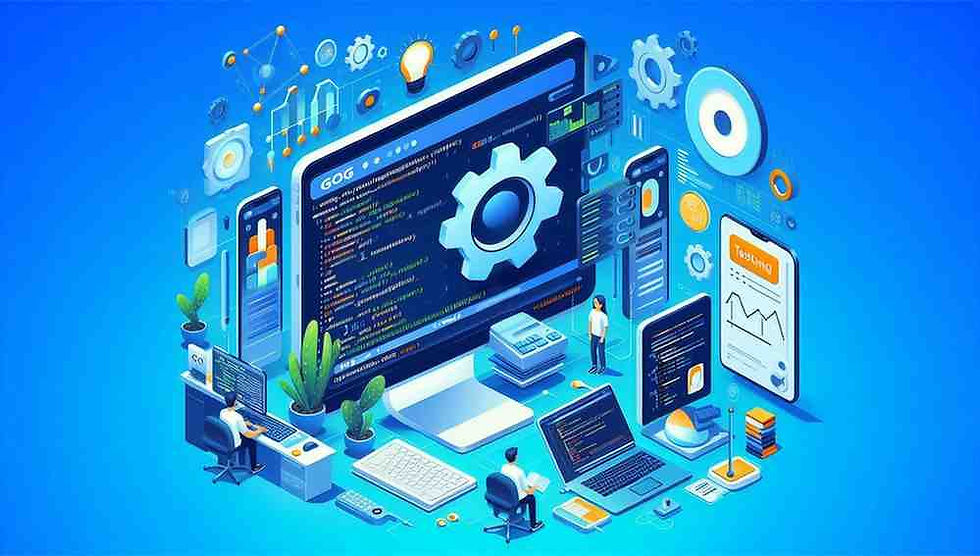
Why You Should Use GoTest
The simplicity and flexibility of GoTest make it a valuable tool for Go developers. Here are some reasons why you should incorporate it into your workflow:
1. Built-in and Easy to Use
GoTest is built into the Go language itself, so there’s no need to install additional tools. Running go test immediately starts executing your test cases.
2. Efficient Feedback Loop
Testing as part of your continuous integration (CI) process ensures you can quickly identify problems and fix them before they grow into bigger issues. GoTest integrates seamlessly with most CI/CD pipelines.
3. Cross-Platform Testing
Since Go compiles to multiple platforms, GoTest lets you write tests once and run them across different environments, ensuring your application is robust across platforms.
4. Supports Parallel Testing
GoTest has native support for parallel testing, meaning you can run multiple test cases simultaneously, saving time on execution, particularly when dealing with large codebases.
How to Get Started with GoTest
Getting started with GoTest is incredibly simple. Here's a basic example:
go
package math
import "testing"
// A simple function to test
func Add(a, b int) int {
return a + b
}
// Unit test for the Add function
func TestAdd(t *testing.T) {
result := Add(2, 3)
if result != 5 {
t.Errorf("Add(2, 3) = %d; want 5", result)
}
}
Save the test file with the name math_test.go. Then, you can run your tests using:
bash
go test ./...
Writing Unit Tests with GoTest
Unit tests focus on verifying the functionality of individual pieces of code, usually at the function or method level. The structure of a unit test in Go looks like this:
Arrange: Set up the environment, inputs, or dependencies required by the function under test.
Act: Execute the function you want to test.
Assert: Compare the output or behavior of the function with the expected result.
Cleanup: Tear down the environment (optional).
Here’s an example of a unit test for a function that reverses a string:
go
func TestReverseString(t *testing.T) {
input := "hello"
expected := "olleh"
result := ReverseString(input)
if result != expected {
t.Errorf("expected %s but got %s", expected, result)
}
}
Running Tests with GoTest
Running tests in Go is simple. By default, the go test command scans your package for _test.go files and execute any functions that start with Test.
bash
go test
If you want more detailed output, use the -v flag:
bash
go test -v
You can also target specific tests by using the -run flag followed by the name of the test you want to run:
bash
go test -run TestReverseString
Advanced Features of GoTest
While GoTest is easy to use, it offers several advanced features that can significantly enhance your testing workflow.
1. Benchmarking
GoTest allows you to measure the performance of your code by writing benchmark tests. Benchmark functions begin with Benchmark and use the b *testing.B parameter.
go
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(2, 3)
}
}
Run benchmarks using:
bash
go test -bench=.
2. Parallel Testing
GoTest supports running tests in parallel to speed up test execution. This is particularly useful for large test suites. To mark a test for parallel execution, use the t.Parallel() method.
go
func TestSomething(t *testing.T) {
t.Parallel()
// test logic
}
3. Example Tests
Go supports example tests, which are code examples that live alongside your functions. These examples can also be run as part of your test suite, ensuring your documentation stays up to date.
go
func ExampleAdd() {
fmt.Println(Add(2, 3))
// Output: 5
}
4. Test Coverage
GoTest provides a way to measure code coverage. You can see which parts of your code are being tested using the -cover flag.
bash
go test -cover
For more detailed coverage reports, use the -coverprofile flag:
bash
go test -coverprofile=coverage.out
go tool cover -html=coverage.out
This will generate an HTML report showing which lines of code are covered by your tests.
Testing Command-Line Interfaces (CLI) in Go
Many CLI tools are written in Go, such as Docker, kubectl, and Terraform. Testing CLI tools can be more challenging than testing standard libraries because of the need to simulate command-line inputs, handle different environments, and capture output streams.
Here’s a general approach to testing CLI tools in Go using GoTest:
1. Simulating Command-Line Input
To simulate input in your tests, you can override os.Args, which is the slice that Go uses to handle command-line arguments.
go
func TestCLI(t *testing.T) {
os.Args = []string{"cmd", "arg1", "arg2"}
main() // Calls the CLI entry point
}
2. Capturing STDOUT and STDERR
To verify output, you’ll want to capture and compare the standard output (STDOUT) and standard error (STDERR) streams. You can redirect these streams to custom buffers:
go
func captureOutput(f func()) string {
var buf bytes.Buffer
stdOut := os.Stdout
os.Stdout = &buf
defer func() { os.Stdout = stdOut }()
f() // Execute the function
return buf.String()
}
3. Testing CLI Tools with testscript
For more complex CLI testing scenarios, testscript is a fantastic library based on Go's internal script_test.go framework. It allows you to write shell-like scripts that test the behavior of your CLI tools by creating temporary files, executing commands, and checking outputs.
Here’s an example script for testing a CLI tool called mytool:
go
func TestScript(t *testing.T) {
testscript.Run(t, testscript.Params{
Dir: "testdata",
})
}
The test script (testdata/mytest.txt) might look like this:
bash
exec mytool
stdout 'expected output'
exec mytool -flag
stderr 'error message'
The testscript library automatically handles file setup, command execution, and cleanup.
Best Practices for Writing Go Tests
When writing Go tests, follow these best practices to ensure the quality and maintainability of your test suite:
1. Keep Tests Isolated
Each test should be independent of others, so they can run in parallel without interference. Use temporary files and mock dependencies where possible.
2. Name Tests Clearly
Choose clear, descriptive names for your test functions, such as TestAddFunction or TestHandleError. This makes it easier to locate and debug failing tests.
3. Write Tests for Edge Cases
Ensure your tests cover both happy paths and edge cases. Testing boundary conditions and unusual inputs will catch bugs that may not appear during normal operation.
4. Use Table-Driven Tests
For functions that need to be tested with multiple inputs, use table-driven tests. This approach keeps your tests DRY (Don't Repeat Yourself) and easy to maintain.
go
func TestMathOperations(t *testing.T) {
cases := []struct {
inputA, inputB int
expected int
}{
{1, 2, 3},
{0, 0, 0},
{-1, -1, -2},
}
for _, c := range cases {
result := Add(c.inputA, c.inputB)
if result != c.expected {
t.Errorf("Add(%d, %d) = %d; want %d", c.inputA, c.inputB, result, c.expected)
}
}
}
5. Test Performance with Benchmarks
Use benchmarks to test the performance of critical functions. If a function is slow or inefficient, a benchmark will reveal the performance cost, helping you optimize your code.
Conclusion
GoTest is an essential tool for Go developers, offering everything you need to write, run, and maintain high-quality tests. Whether you’re testing individual functions, measuring performance, or ensuring your CLI behaves as expected, GoTest has you covered. By following best practices and leveraging Go’s advanced testing features, you can build reliable, bug-free applications.
Key Takeaways
GoTest is Go’s built-in tool for writing and running tests.
It supports unit tests, integration tests, benchmarks, and example tests.
Use go test to run tests, with options like -v for verbose output or -run to target specific tests.
Parallel and benchmark testing are powerful features for improving performance.
Test your CLI tools by simulating command-line inputs and capturing outputs.
Libraries like testscript can simplify complex CLI testing scenarios.
FAQs
1. How do I run tests in Go?
To run tests in Go, use the go test command. It automatically runs all tests in files ending with _test.go.
2. What is a table-driven test in Go?
A table-driven test is a way to test multiple input scenarios by iterating over a list of test cases in a loop, reducing redundancy in your test code.
3. How do I measure test coverage in Go?
Use the -cover flag with go test to see test coverage. For detailed reports, use -coverprofile to generate a coverage report.
4. Can I run Go tests in parallel?
Yes, you can run tests in parallel by using the t.Parallel() method inside your test function.
5. How do I write benchmark tests in Go?
Benchmark tests in Go are written using functions that start with Benchmark and use the b *testing.B parameter. Run them with go test -bench=.
6. What is the best way to test CLI tools in Go?
For testing CLI tools, simulate command-line inputs using os.Args, capture output streams and consider using libraries like testscript for more complex scenarios.
7. How do I check if my Go code is optimized for performance?
You can write benchmark tests using Go’s testing package and run them to measure and optimize the performance of critical code paths.
8. How do I test my Go code across different platforms?
GoTest is cross-platform, meaning you can write tests once and run them on different operating systems by compiling your code for each target platform.
Comments