iFrames, or inline frames, play an essential role in embedding external content into a web page. Whether it's embedding a video, form, advertisement, or any interactive third-party content, iFrames makes it easy to load these elements without affecting the host page's structure. In this comprehensive guide, we’ll explore iFrame examples, best practices, security considerations, and even some advanced use cases to enhance your web development projects.
What is an iFrame?
An iFrame (inline frame) is an HTML element that allows you to embed another HTML page within your current web page. The embedded page can come from the same domain or a different domain altogether, which can lead to cross-domain access issues (discussed later in this guide). iFrames are useful for embedding videos, ads, forms, widgets, or interactive content like maps and charts.
Here is the basic syntax of an iFrame:
html
<iframe src="https://example.com" width="600" height="400"></iframe>
This line of code will embed content from https://example.com into your web page, displaying it in a box 600 pixels wide and 400 pixels tall.
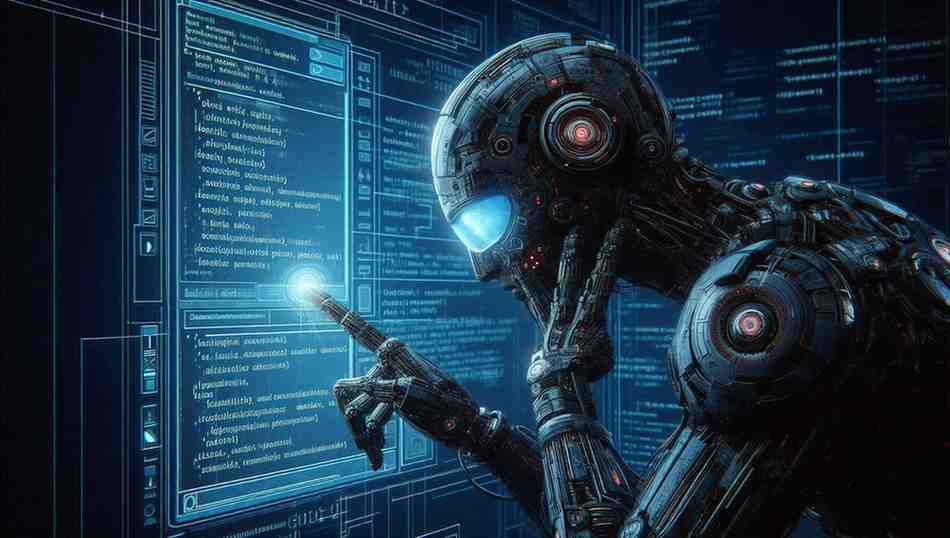
Basic iFrame Examples
Let's start with some basic iFrame examples to illustrate its use in web development.
1. Embedding a YouTube Video
One of the most common uses of iFrames is embedding a YouTube video. Here's how you can embed a video into your website using an iFrame:
html
<iframe width="560" height="315" src="https://www.youtube.com/embed/VIDEO_ID" frameborder="0" allowfullscreen></iframe>
Replace VIDEO_ID with the unique identifier of the YouTube video, and the video will appear embedded on your page.
2. Embedding a Google Map
You can also use iFrames to embed Google Maps for directions or location services:
html
<iframe src="https://www.google.com/maps/embed?pb=!1m18!1m12!1m3!1d3153.0909497266476!2d144.9643933156933!3d-37.80751897975179!2m3!1f0!2f0!3f0!3m2!1i1024!2i768!4f13.1!3m3!1m2!1s0x6ad642af0f11fd9d%3A0x5045675218ce6e0!2sMelbourne%20VIC%2C%20Australia!5e0!3m2!1sen!2sus!4v1600663512292!5m2!1sen!2sus" width="600" height="450" frameborder="0" style="border:0;" allowfullscreen="" aria-hidden="false" tabindex="0"></iframe>
This iFrame code will display a map centered on Melbourne, Australia. You can easily modify the latitude and longitude parameters to adjust the map's focus.
3. Embedding a Contact Form
iFrames are also handy for embedding forms from third-party services like Google Forms:
html
<iframe src="https://docs.google.com/forms/d/e/YOUR_FORM_ID/viewform?embedded=true" width="640" height="800" frameborder="0" marginheight="0" marginwidth="0">Loading…</iframe>
By using this code, you can embed a Google Form directly into your webpage, making it easy for visitors to submit their responses without leaving your site.
Advanced iFrame Examples
While embedding a video or a form is straightforward, working with iFrames becomes more complex when dealing with advanced use cases such as third-party APIs, security protocols, and cross-domain issues.
1. Embedding iFrames from a Different Domain
An iFrame can load content from another domain, which is known as a cross-domain iFrame. However, due to security restrictions, this can cause issues. Browsers often block certain interactions between the parent page and the iFrame to prevent malicious activity, known as Cross-Origin Resource Sharing (CORS) issues.
Here’s an example of an iFrame that loads an external webpage:
html
<iframe src="https://www.external-domain.com" width="800" height="600"></iframe>
2. Responsive iFrames
In modern web design, it's crucial to make your iFrames responsive so they adapt to different screen sizes. One way to do this is by using CSS to make the iFrame adjust dynamically to the parent container’s size.
css
.responsive-iframe {
width: 100%;
height: auto;
}
html
<iframe class="responsive-iframe" src="https://www.example.com"></iframe>
3. Handling iFrame Load Delays
If you’re embedding third-party content that loads slowly, you can manage the loading process using JavaScript or lazy-loading techniques. Here’s an example of how you can detect if the iFrame has fully loaded:
html
<iframe id="myIframe" src="https://example.com"></iframe>
<script>
document.getElementById('myIframe').onload = function() {
console.log('iFrame has loaded');
};
</script>
This script will log a message to the console when the iFrame fully loads.
iFrame Security Considerations
While iFrames offer many benefits, they can also pose serious security risks if not used correctly. Here are some ways to mitigate those risks:
1. X-Frame-Options Header
To protect your site from clickjacking attacks, you can add the X-Frame-Options header to your HTTP response. This header tells the browser whether the page is allowed to be embedded in an iFrame.
DENY: The page cannot be displayed in an iFrame.
SAMEORIGIN: The page can be embedded only if the request is from the same domain.
apache
Header always append X-Frame-Options SAMEORIGIN
2. Content Security Policy (CSP)
Another security measure is implementing a Content Security Policy (CSP) that controls which domains are allowed to be included in iFrames.
http
Content-Security-Policy: frame-ancestors 'self' https://trusted-site.com
This restricts the iFrame embedding to trusted domains only, preventing unauthorized websites from embedding your content.
SEO Implications of Using iFrames
While iFrames are useful, they can have negative implications for SEO. Search engine crawlers, like Google's, often have difficulty indexing content within iFrames because it is technically separate from the parent page. This means that critical content should not be placed within an iFrame if you want it to be indexed.
1. Crawling Issues
Search engines may not be able to access or index the content inside iFrames, especially when it's from another domain. For example, if your iFrame loads content from an external domain that is disallowed by the site’s robots.txt, Google won’t index that content.
2. Solutions
Direct Embedding: If possible, avoid using iFrames for critical content like blogs, products, or landing pages that you want search engines to crawl.
Transcripts or NoScript: Provide a fallback for the iFrame by including a transcript or description in the HTML to make the content more SEO-friendly.
Example:
html
<noscript>Your browser does not support iFrames, but you can still visit the page by clicking <a href="https://example.com">here</a>.</noscript>
Handling iFrames with JavaScript
When working with iFrames, you often need to interact with the content inside the frame, such as detecting button clicks or retrieving form data. However, this can be tricky due to cross-domain security restrictions.
1. Accessing iFrame Content
To manipulate the content inside an iFrame from JavaScript, you need to ensure the iFrame is from the same domain. Otherwise, the browser will block access to avoid security risks.
Here’s an example of accessing iFrame content with JavaScript:
html
<iframe id="myIframe" src="https://same-domain.com"></iframe>
<script>
const iframe = document.getElementById('myIframe');
const iframeDocument = iframe.contentDocument ||
iframe.contentWindow.document;
console.log(iframeDocument.body.innerHTML);
</script>
2. Cross-Domain Workarounds
If you must interact with content from a different domain, you can set up postMessage communication between the parent page and the iFrame. This technique allows secure, cross-origin communication.
html
<iframe id="myIframe" src="https://external-domain.com"></iframe>
<script>
window.addEventListener('message', function(event) {
if (event.origin === 'https://external-domain.com') {
console.log('Message received:', event.data);
}
});
</script>
In this setup, the external domain can send data back to the parent page using postMessage.
Conclusion
iFrames provides a flexible and straightforward way to embed external content into your web pages. From basic examples like embedding YouTube videos or Google Maps to more advanced use cases involving cross-domain communication and security measures, iFrames are a powerful tool when used correctly. However, they also come with challenges, such as SEO implications and potential security vulnerabilities. Understanding these complexities ensures that you can implement iFrames effectively while mitigating risks.
Key Takeaways
iFrames are used to embed external content into a webpage.
Common iFrame uses include embedding videos, maps, and forms.
Cross-domain iFrames can lead to security and accessibility issues.
iFrames can pose SEO challenges due to content being difficult for crawlers to index.
Security best practices include setting X-Frame-Options and using a CSP.
You can interact with iFrame content using JavaScript if the iFrame is from the same domain.
For cross-domain iFrames, use the postMessage API for communication.
Always ensure that third-party iFrame content is safe and reputable.
FAQs About iFrames
1. Can I use iFrames for SEO-critical content?
No, iFrames can negatively affect SEO because search engines might not index the content. Direct embedding is preferable.
2. Are iFrames still relevant today?
Yes, iFrames are widely used for embedding third-party content like videos, forms, and advertisements.
3. How do I make iFrames responsive?
Use CSS with percentage-based widths and height adjustments to make iFrames responsive across devices.
4. What is the X-Frame-Options header?
This HTTP header prevents your webpage from being embedded in iFrames, protecting it from clickjacking attacks.
5. How do I solve cross-domain issues with iFrames?
You can use the postMessage API to safely communicate between the parent page and the cross-domain iFrame.
6. Are there security risks with iFrames?
Yes, iFrames can be a security risk if the embedded content comes from untrusted sources. Always vet the content and implement security policies like X-Frame-Options.
7. Can I spy on network calls made by an iFrame?
Yes, using testing tools like Cypress, you can monitor and even stub network calls made from within iFrames.
8. How do I embed interactive content in an iFrame?
You can embed interactive content like forms, maps, or games by loading the respective URLs into the iFrame's src attribute.
Comments