Guide to Keyword-Driven Framework for Selenium
- Gunashree RS
- Oct 8, 2024
- 7 min read
In the world of software testing, automation is key to improving efficiency and reducing errors. However, managing and maintaining test scripts can become complex and time-consuming as the number of test cases grows. Enter the Keyword Driven Framework, a popular approach in automation testing that allows for better organization, maintenance, and ease of use. This framework simplifies test creation by separating test design from code implementation, making it easier for both technical and non-technical testers to contribute to test automation.
In this comprehensive guide, we'll explore what a keyword-driven framework is, its benefits, how to implement it in Selenium WebDriver, and why it is a preferred choice for many QA teams.
What is a Keyword Driven Framework?
A keyword-driven framework is a type of test automation framework that uses keywords to represent actions to be performed during testing. These keywords act as an abstraction layer between the test cases and the underlying automation code, allowing testers to define test cases using high-level keywords instead of writing complex code. The idea is to separate the test logic (what needs to be tested) from the test implementation (how it is tested).
In simple terms, testers define keywords like "click," "input," "open browser," and "navigate," which are mapped to actual test scripts or functions in the framework. This allows non-technical stakeholders to understand and even create test cases without needing to know the automation code behind them.
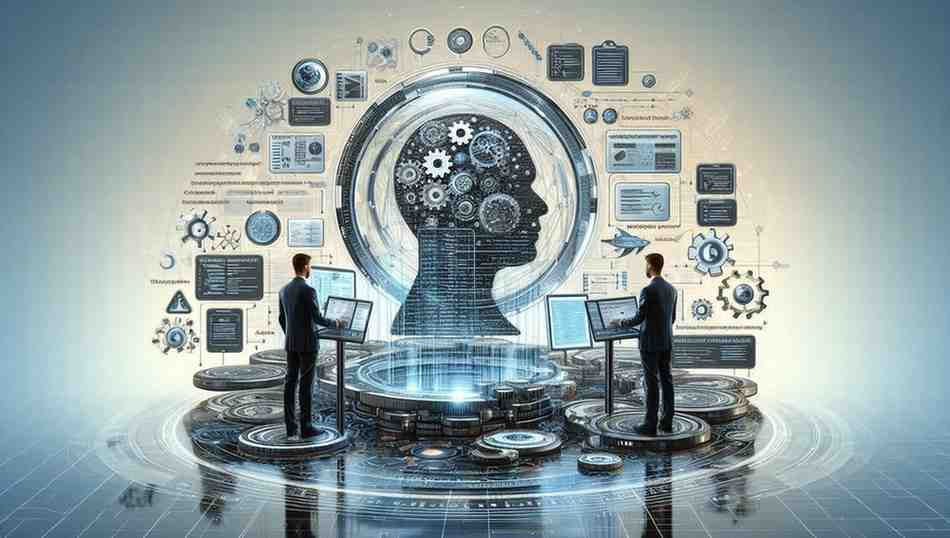
Key Features of a Keyword-Driven Framework:
Abstraction: Test cases are created using keywords rather than complex code.
Reusability: Keywords can be reused across different test cases, promoting efficiency.
Modular Approach: Test steps are separated into keywords, making test cases easier to manage and modify.
Data-Driven: It can be combined with data-driven testing, allowing different inputs to be tested with the same set of keywords.
Non-technical Contribution: Non-technical team members can contribute to test case creation by using predefined keywords.
Why Use a Keyword-driven Framework in Selenium?
When working with a test automation tool like Selenium WebDriver, managing large test suites can quickly become overwhelming. A Keyword-driven Driven Framework helps to address this challenge by introducing a structured way of managing test scripts and data. Here are some reasons why teams use this framework:
1. Ease of Maintenance
Because test scripts are broken into reusable keywords, making changes to tests becomes easier. You can update the keyword logic without having to rewrite the entire test case.
2. Separation of Test Logic and Code
Testers and business users can create or modify test cases without knowing how to code. The automation team only needs to implement the keywords once, after which the keywords can be used to build multiple test cases.
3. Reusable Components
Keywords can be reused across multiple test cases, which reduces the duplication of code. For example, a keyword like "Login" can be reused in various tests without having to rewrite the logic every time.
4. Scalability
As the application grows, maintaining a large number of test cases becomes easier when using a keyword-driven framework. You can add new keywords or modify existing ones to accommodate new functionality without affecting other test cases.
5. Enhanced Collaboration
The separation between the test logic and the code enables better collaboration between technical and non-technical team members. Business analysts, manual testers, or product managers can help define tests without needing to understand the underlying code.
Components of a Keyword-Driven Framework
A well-structured keyword-driven framework consists of several key components that work together to make the framework efficient, reusable, and maintainable. Below are the main components:
1. Keyword Library
This is a collection of reusable functions or methods that are mapped to specific keywords. For instance, a "Click" keyword would map to a method that performs a click action using Selenium WebDriver.
2. Test Data
Test data is typically stored in external files, like Excel sheets or CSV files. Each test case may require specific inputs, such as usernames, passwords, URLs, etc., which are retrieved from these files.
3. Object Repository
An Object Repository stores the locators for all web elements that need to be interacted with during the test. This could be XPaths, CSS selectors, or any other types of locators that Selenium uses to find elements on a web page.
4. Test Scripts
These scripts contain the logic to read the keywords and execute the corresponding actions. The test script acts as the driver, processing the test case step by step.
5. Excel/CSV File (Keyword Mapping)
The external file (typically an Excel sheet) holds the sequence of keywords that define the actions to be taken in a test case. Each row in the file represents a step in the test case, with columns for the keyword, test data, and any necessary parameters.
6. Driver Script
The driver script reads the test steps from the Excel file, executes the corresponding keywords, and interacts with the application under test. It serves as the backbone of the framework, ensuring everything runs smoothly.
Implementing a Keyword Driven Framework in Selenium WebDriver
Now that we understand the components, let’s take a closer look at how to implement a Keyword Driven Framework in Selenium WebDriver. We'll walk through an example scenario that automates logging into a web application using the framework.
Step-by-Step Guide
Step 1: Set Up Your Project in Selenium
Start by setting up your Selenium WebDriver environment. You will need to configure Selenium with a build tool like Maven and add the necessary dependencies for Selenium WebDriver and Apache POI (to handle Excel files).
Example Maven pom.xml Configuration:
xml
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>5.3.1</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.11</version>
</dependency>
</dependencies>
Step 2: Create Keywords for Common Actions
Next, define your keywords as methods in a separate class. These methods will represent common actions like "Open Browser," "Click," "Enter Text," etc.
Example:
java
public class Action_Keyword {
public static WebDriver driver;
public void openBrowser() {
System.setProperty("webdriver.chrome.driver", "path_to_driver");
driver = new ChromeDriver();
driver.manage().window().maximize();
}
public void navigate() {
driver.get(Constants.URL);
}
public void clickSignIn() {
driver.findElement(By.id("signin")).click();
}
public void enterUsername() {
driver.findElement(By.id("username")).sendKeys(Constants.username);
}
public void enterPassword() {
driver.findElement(By.id("password")).sendKeys(Constants.password);
}
public void clickLogin() {
driver.findElement(By.id("login-btn")).click();
}
public void closeBrowser() {
driver.quit();
}
}
Step 3: Create the Excel Sheet
In the Excel sheet, define your test steps using keywords and the corresponding input data. For example:
Step No | Action Keyword | Locator | Test Data |
1 | Open Browser | ||
2 | Navigate | ||
3 | Click | signin | |
4 | Input | username | test_user |
5 | Input | password | test_password |
6 | Click | login-btn | |
7 | Close Browser |
Step 4: Implement the Driver Script
The driver script will read the keywords from the Excel sheet and execute the corresponding methods.
Example:
java
public class ExecutionTest {
public static void main(String[] args) throws Exception {
// Create an instance of ReadExcelSheet and Action_Keyword
ReadExcelSheet rs = new ReadExcelSheet();
Action_Keyword action = new Action_Keyword();
// Open browser
action.openBrowser();
// Read the Excel file and execute actions based on the keywords
for (int i = 0; i < rs.getRowCount(); i++) {
String keyword = rs.getCellData(i, 1);
if (keyword.equals("Open Browser")) {
action.openBrowser();
} else if (keyword.equals("Navigate")) {
action.navigate();
} else if (keyword.equals("Click SignIn")) {
action.clickSignIn();
} else if (keyword.equals("Input Username")) {
action.enterUsername();
} else if (keyword.equals("Input Password")) {
action.enterPassword();
} else if (keyword.equals("Click Login")) {
action.clickLogin();
} else if (keyword.equals("Close Browser")) {
action.closeBrowser();
}
}
}
}
Advantages of Keyword Driven Framework in Selenium
1. High Reusability
The framework allows you to reuse the same keywords across multiple test cases. This reduces redundancy and the effort required to maintain test scripts.
2. Platform Independence
Test scripts created using a keyword-driven framework can be easily executed on different platforms, including Windows, Linux, or macOS.
3. Improved Collaboration
Non-technical stakeholders can contribute to test creation by working with keywords, making it easier to collaborate across teams.
4. Easy Maintenance
If changes are made to the application, only the relevant keyword needs to be updated, rather than all test cases.
5. Separation of Concerns
Test logic is separated from test implementation, making the framework cleaner and easier to understand.
Disadvantages of Keyword-Driven Framework
1. Initial Setup Time
The framework requires extensive initial planning and setup, especially when creating the keyword library and structuring the framework.
2. Complexity for Large Applications
For very large applications, managing a large set of keywords and maintaining the Excel sheets can become cumbersome.
3. Expertise Required
Setting up and maintaining the framework still requires technical expertise, especially when defining complex keywords.
FAQs
1. What is a Keyword Driven Framework?
A keyword-driven framework is a test automation framework that separates test logic from code by using keywords to represent actions. Testers define keywords in an external file, which are mapped to corresponding automation functions.
2. How does a keyword-driven framework work in Selenium?
In Selenium, a keyword-driven framework reads test steps (keywords) from an external file (like Excel) and executes them using predefined methods in the automation code.
3. What are the benefits of using a keyword-driven framework?
The primary benefits include reusability, ease of maintenance, platform independence, and enhanced collaboration between technical and non-technical teams.
4. What tools are used in a keyword-driven framework?
Common tools include Selenium WebDriver for automation, Apache POI for reading Excel files, and test frameworks like TestNG or JUnit.
5. How is Keyword Driven Testing different from Data Driven Testing?
Keyword Driven Testing focuses on using keywords to define test steps, while Data Driven Testing is concerned with running the same tests with different sets of input data.
6. Can non-technical users create tests in a keyword-driven framework?
Yes, one of the key advantages of this framework is that non-technical users can define test cases using predefined keywords without needing to write code.
Conclusion
The Keyword Driven Framework is an efficient and scalable approach to test automation that simplifies test case creation and management. By abstracting the test logic from the code, this framework makes it easier for teams to collaborate, maintain test scripts, and ensure higher-quality software. While it requires more setup time initially, the long-term benefits—such as easier maintenance, reusability, and scalability—make it a popular choice among QA teams using Selenium WebDriver.
Key Takeaways:
A keyword-driven framework separates test logic from implementation by using predefined keywords.
It allows for easy collaboration between technical and non-technical stakeholders.
The framework improves test script reusability and reduces maintenance efforts.
Initial setup can be time-consuming, but it pays off in the long run.
Selenium WebDriver and Apache POI are commonly used tools in this framework.
Sources:
Comments