Selenium is one of the most widely used automation testing frameworks for web applications, allowing developers and testers to automate interactions with browsers. Whether you’re upgrading from Selenium 3 or starting fresh with Selenium 4, understanding how to install Selenium for Java and JavaScript is critical. In this comprehensive guide, we will walk you through how to install Selenium, set up the necessary dependencies using different build tools like Maven and Gradle, and get started with both Java and JavaScript.
We will cover everything from installation methods to writing and executing basic tests. By the end of this guide, you'll have a fully functional Selenium setup for automating your web application tests.
1. What is Selenium?
Selenium is an open-source web automation tool used to automate web applications for testing purposes. It supports multiple browsers, including Chrome, Firefox, Internet Explorer, and more. Selenium can be used with various programming languages like Java, JavaScript, Python, C#, and Ruby, providing great flexibility for developers and testers.
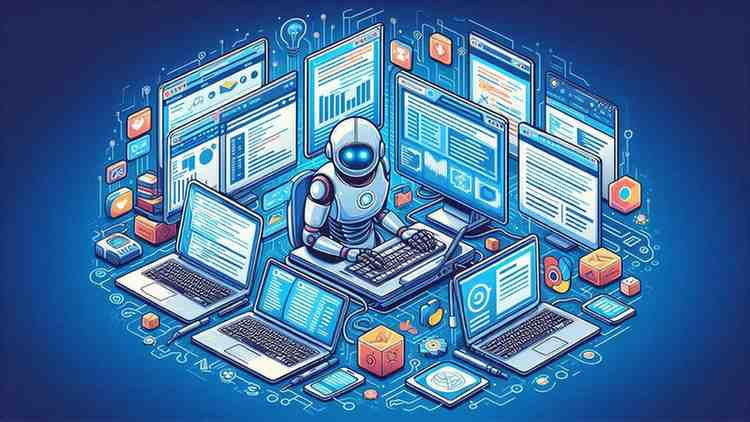
Selenium comprises several components:
Selenium WebDriver: Drives a browser natively, making it the most popular tool for web automation.
Selenium Grid: Enables running tests on multiple machines and browsers in parallel.
Selenium IDE: A Chrome and Firefox extension for record-and-playback testing.
2. Why is Selenium Essential for Web Testing?
Selenium is indispensable for testing web applications because it allows for automated, repeatable, and scalable testing across different browsers and platforms. Some reasons why Selenium is essential include:
Cross-browser compatibility: Test your application across multiple browsers and platforms.
Automation: Reduces manual effort, allowing faster test execution and saving time.
Language flexibility: Use Selenium with multiple programming languages like Java, JavaScript, Python, and C#.
Continuous Integration (CI) support: Integrates seamlessly with CI tools like Jenkins, ensuring rapid testing with every new code deployment.
3. System Prerequisites for Selenium Install
Before you begin installing Selenium, make sure you have the following system prerequisites:
Java Development Kit (JDK): Selenium requires Java to run (for Java bindings). Make sure you have JDK 8 or higher installed. You can check your version using:
bash
java -version
Node.js and npm (for JavaScript bindings): JavaScript-based Selenium installations require Node.js. Ensure you have the latest version of Node.js and npm installed. You can verify their versions with:
bash
node -v
npm -v
Browser drivers: Selenium interacts with browsers via WebDriver implementations like chromedriver for Chrome, geckodriver for Firefox, and so on. These drivers must be installed separately.
4. Selenium Install for Java
Selenium can be installed for Java in different ways: using Maven, Gradle, or manually downloading the necessary libraries.
Using Maven for Selenium Install
Maven is a popular build tool for Java projects that manages project dependencies and automates the build process. Follow these steps to install Selenium using Maven:
Install Maven: Ensure Maven is installed on your system. You can verify the installation by running:
bash
mvn -version
Create a Maven Project: You can create a Maven project manually or by using an IDE like IntelliJ or Eclipse. Once the project is set up, update your pom.xml file with the Selenium dependency:
xml
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
</dependencies>
Download Dependencies: Run the Maven build command to download the required Selenium libraries:
bash
mvn clean install
Using Gradle for Selenium Install
Gradle is another build tool for Java that is increasingly popular due to its flexibility and use of Groovy or Kotlin DSL for configuration. Here’s how to install Selenium using Gradle:
Install Gradle: If Gradle is not already installed, you can download and install it manually. Once installed, verify the installation:
bash
gradle -v
Create a Gradle Project: Create a new Gradle project or configure your existing project by adding the following to your build.gradle file:
groovy
dependencies {
implementation 'org.seleniumhq.selenium:selenium-java:4.0.0'
implementation 'commons-io:commons-io:2.6'
}
Download Dependencies: Run Gradle to download the Selenium libraries:
bash
gradle build
Manual Selenium Install for Java
If you prefer not to use a build tool, you can manually install Selenium by downloading the jar files:
Download Selenium: Visit the official Selenium downloads page and download the Selenium Standalone Server (Java bindings).
Add Selenium Jars to Your Project: In your IDE (e.g., IntelliJ or Eclipse), navigate to your project settings and add the Selenium jar files as external libraries.
Add Browser Drivers: Download the relevant browser drivers (e.g., chromedriver, geckodriver) and set their paths using:
java
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
5. Selenium Install for JavaScript
For JavaScript users, Selenium installation is simplified by npm, the Node.js package manager. Follow these steps to install Selenium for JavaScript:
Using npm for Selenium Install
Install Node.js: Ensure Node.js and npm are installed. If not, download and install them from the official Node.js website.
Initialize npm: Navigate to your project folder and initialize npm by running:
bash
npm init
Install Selenium WebDriver: Use npm to install Selenium WebDriver:
bash
npm install selenium-webdriver
Configure Browser Drivers: Download browser drivers for Chrome, Firefox, or any other browser. Set the driver path in your test file:
javascript
const { Builder } = require('selenium-webdriver');
let driver = new Builder().forBrowser('chrome').build();
Setting Up Browser Drivers for JavaScript
After installing Selenium WebDriver, you'll need to download the appropriate browser drivers:
chromedriver: For Chrome
geckodriver: For Firefox
msedgedriver: For Edge
Make sure to add these drivers to your system path or explicitly set their path in your code. For example:
javascript
const driver = new Builder().forBrowser('chrome').setChromeOptions(options).build();
6. Writing Your First Selenium Test
Once Selenium is installed, you’re ready to write and run your first test.
Java Example
java
package Tests;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class GoogleSearchTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver",
"/path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.google.com");
driver.findElement(By.name("q")).sendKeys("Selenium 4");
driver.findElement(By.name("btnK")).click();
driver.quit();
}
}
JavaScript Example
javascript
const { Builder, By, Key } = require('selenium-webdriver');
async function example() {
let driver = await new Builder().forBrowser('chrome').build();
await driver.get('https://www.google.com');
await driver.findElement(By.name('q')).sendKeys('Selenium 4', Key.RETURN);
await driver.quit();
}
example();
7. Benefits of Upgrading to Selenium 4
Selenium 4 brings several exciting updates that enhance web automation testing. Key features include:
Improved WebDriver APIs: Simplifies interaction with browser windows and tabs.
Relative Locators: Easier identification of elements relative to others.
DevTools Support: Full support for Chrome DevTools Protocol, enabling network and performance monitoring.
8. Common Challenges in Selenium Installation
While installing Selenium is relatively straightforward, you may encounter the following challenges:
Browser compatibility issues: Ensure that you're using the correct WebDriver version for the browser you're testing.
Environment setup problems: Set environment variables correctly for Java or JavaScript, including browser driver paths.
Dependency conflicts: When using Maven or Gradle, make sure to resolve any conflicting dependencies.
9. Best Practices for Selenium Setup
To get the most out of your Selenium setup, follow these best practices:
Use version control for your test dependencies to avoid inconsistencies.
Leverage build tools like Maven or Gradle for better dependency management.
Use explicit waits to handle dynamic content loading instead of fixed delays.
Modularize tests by creating reusable functions for commonly used actions.
Keep browser drivers up to date for compatibility with the latest browser versions.
10. FAQs on Selenium Install
Q1. What programming languages can I use with Selenium?
Selenium supports Java, JavaScript, Python, C#, Ruby, and more.
Q2. How do I install browser drivers for Selenium?
You can download browser drivers like chromedriver or geckodriver from the official Selenium website and configure them in your system path.
Q3. Can I use Selenium for mobile testing?
While Selenium is primarily for web testing, mobile testing can be performed using Appium, which extends Selenium for mobile platforms.
Q4. How do I update Selenium to the latest version?
For Java, update your Maven pom.xml or Gradle build.gradle file to the latest Selenium version. For JavaScript, use npm update selenium-webdriver.
Q5. Can Selenium test desktop applications?Selenium is designed for web testing. For desktop application automation, tools like WinAppDriver or AutoIt are recommended.
11. Conclusion
Installing Selenium for Java or JavaScript is a straightforward process that allows you to automate web testing efficiently. Whether you choose to use Maven, Gradle, or manual installation, having a robust Selenium setup is crucial for maintaining a high standard of web application quality. By upgrading to Selenium 4, you'll benefit from enhanced APIs, improved browser support, and new features that streamline the automation process.
Key Takeaways
Selenium is a powerful tool for automating web browser interactions.
Installing Selenium requires setting up WebDriver for your chosen browser (e.g., Chrome, Firefox).
Build tools like Maven and Gradle simplify Selenium installation for Java projects.
JavaScript projects can use npm to install Selenium WebDriver for automated browser testing.
Selenium 4 introduces enhanced WebDriver APIs, relative locators, and Chrome DevTools support.
Comments