In the world of programming, languages are broadly categorized into two types: statically typed and dynamically typed. A dynamically typed language offers developers the flexibility to change the data type of a variable at runtime. This flexibility is one of the key reasons why languages like JavaScript, Python, Ruby, and Lua are popular, especially for rapid development and prototyping. However, this flexibility comes with its own set of challenges, particularly when it comes to efficiently managing and storing data types in memory.
In this comprehensive guide, we’ll dive deep into the mechanisms that allow dynamic languages to handle data types efficiently. We’ll explore concepts such as virtual machines (VMs), tagged unions, NaN boxing, and pointer tagging. By the end of this article, you will have a thorough understanding of how dynamic languages operate under the hood and how they manage to balance flexibility with performance.
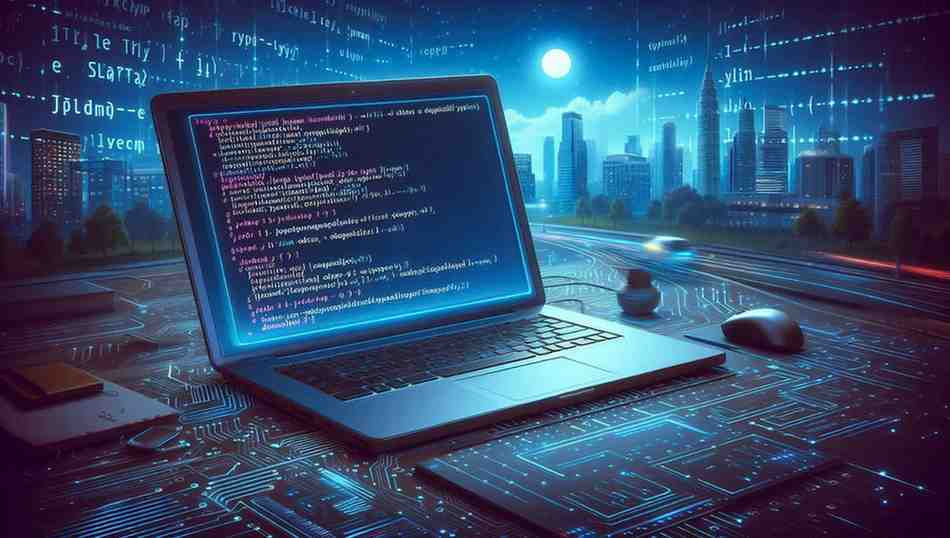
What Does It Mean for a Language to Be Dynamic?
A dynamic language is one in which variables do not have a fixed data type at compile-time. Instead, the data type of a variable can change during runtime. This characteristic allows developers to write more flexible and concise code but also introduces the challenge of managing different data types efficiently.
Dynamic Typing vs. Static Typing
Dynamic Typing: The type of a variable is determined at runtime. This allows for more flexible code but can lead to runtime errors if types are misused.
Static Typing: The type of a variable is determined at compile-time. This often results in more optimized code but requires more verbose type declarations.
Dynamic languages often provide strong type guarantees at runtime, ensuring that operations on variables are type-safe. However, this runtime flexibility requires the underlying language implementation to be efficient in handling these dynamic types.
How Dynamic Languages Operate at Runtime
Most dynamic languages rely on an intermediate representation called "bytecode" to operate at runtime. Bytecode is a low-level, platform-independent representation of the code that a virtual machine (VM) interprets. VMs are responsible for executing bytecode and managing the runtime environment, including data types.
Stack-Based vs. Register-Based Virtual Machines
There are two primary types of VMs used by dynamic languages:
Stack-Based VM: Uses a stack to store and manage data. Instructions in the bytecode push and pop values from the stack as needed.
Register-Based VM: Uses a set of registers (which can be thought of as named slots in memory) to store and manage data. This type of VM is generally more efficient as it reduces the number of memory operations.
The choice between a stack-based or register-based VM affects how data types are handled and optimized in memory.
The Core Challenge: Representing Dynamic Values
In a dynamically typed language, variables can hold any type of data at any time. This presents a challenge for the language's runtime system: how do you design a data structure that can efficiently store different types of data without wasting memory?
Fixed Set of Data Types
Most dynamic languages have a fixed set of data types. For example, JavaScript has data types such as number, string, boolean, object, symbol, and undefined. A variable can hold any one of these types at any time, and the runtime must be able to handle this flexibility efficiently.
Bloated Structs: A Simple but Inefficient Approach
One approach to handling dynamic data types is to use a struct that contains a field for each possible data type. For instance, in a C-like implementation, you might define a struct like this:
c
typedef struct Value_t {
double number;
bool boolean;
void* object; // For heap-allocated types like strings, arrays, etc.
} Value;
While this approach is straightforward, it is also inefficient because the struct always allocates memory for all possible types, even though only one type is used at any given time. This leads to unnecessary memory consumption, especially for large data structures.
Tagged Unions: A More Efficient Representation
A more efficient approach is to use a tagged union. A tagged union is a data structure that stores only the data type currently in use and a tag to indicate the type. This allows the struct to dynamically change its memory usage based on the type of data it holds.
Implementing a Tagged Union
Here’s how you might implement a tagged union in C:
c
typedef enum { T_NUM, T_BOOL, T_OBJECT } Type;
typedef struct {
Type type;
union {
double number;
bool boolean;
void* object;
} data;
} Value;
With this structure, the Value can hold a number, a boolean, or an object, but never more than one at a time. The type field indicates which type is currently stored in the union.
Example: Adding Values with a Tagged Union
Let’s consider a function that adds two Value structs together, handling both numbers and strings:
c
Value add_values(const Value* a, const Value* b) {
Value result;
if (a->type == T_NUM && b->type == T_NUM) {
result.type = T_NUM;
result.data.number = a->data.number + b->data.number;
} else if (a->type == T_OBJECT && b->type == T_OBJECT) {
result.type = T_OBJECT;
result.data.object = concat_strings(a->data.object, b->data.object);
}
return result;
}
In this implementation, the function checks the type of each Value and performs the appropriate operation based on the type. This method is both flexible and memory-efficient.
NaN Boxing: An Advanced Optimization Technique
For those who demand even more efficiency, NaN boxing is a technique used by some interpreters to further optimize memory usage. NaN boxing exploits the fact that the IEEE-754 standard for floating-point numbers reserves certain bit patterns to represent special values like NaN (Not a Number).
How NaN Boxing Works
In IEEE-754, a double-precision floating-point number is 64 bits long, with specific bits reserved for the sign, exponent, and mantissa. If all the exponent bits are set to 1, the value is considered NaN. By leveraging the fact that NaN values have unused bits, we can store additional information, such as pointers or type tags, within these bits.
Here’s a basic example:
c
typedef union {
uint64_t bits;
double number;
} BoxedValue;
With NaN boxing, you can distinguish between different types based on the specific bit patterns in the BoxedValue:
Numbers: Use standard floating-point representation.
Objects: Use NaN with a specific bit pattern to indicate a pointer to a heap-allocated object.
Singletons (e.g., null, true, false): Use other specific bit patterns within NaN to
represent these values.
Pointer Tagging: A Different Approach
Another technique used by some interpreters is pointer tagging. This method takes advantage of the fact that pointers on most modern systems are aligned to 8-byte boundaries, meaning the last three bits of a pointer are always zero. These bits can be used to store type information.
Implementing Pointer Tagging
Here’s an example of how pointer tagging might be implemented:
c
define TAG_OBJECT 0b000
define TAG_TRUE 0b001
define TAG_FALSE 0b010
define TAG_NULL 0b011
define TAG_NUM 0b100
typedef void* Value;
define MASK_TAG 0x7
define GET_TAG(val) ((uintptr_t)(val) & MASK_TAG)
define SET_TAG(val, tag) ((Value)((uintptr_t)(val) | tag))
In this system, a Value can either be a pointer to an object or a special value like null, true, or false, with the tag bits indicating the type. This method is particularly efficient because it allows the interpreter to quickly determine the type of a value without needing additional memory.
Efficiency Considerations in Dynamic Languages
The techniques described above are crucial for making dynamic languages efficient. Without these optimizations, the flexibility of dynamic typing would come at the cost of performance and memory usage.
Trade-offs
Memory Efficiency vs. Flexibility: More sophisticated techniques like NaN boxing and pointer tagging save memory but can be more complex to implement and may be architecture-dependent.
Execution Speed: Techniques that reduce memory usage also tend to improve execution speed by reducing cache misses and improving data locality.
Real-World Applications
These techniques are not just theoretical—they are used by some of the most popular dynamic language interpreters today:
Lua: Uses a tagged union for its TValue representation.
CPython: Uses a similar approach with its PyObject structure.
JavaScript Engines (V8, SpiderMonkey): Use NaN boxing and pointer tagging to optimize performance.
Conclusion
Understanding how dynamic languages efficiently handle data types is crucial for anyone working with or implementing interpreters for these languages. From simple tagged unions to advanced techniques like NaN boxing and pointer tagging, these methods allow dynamic languages to provide the flexibility developers love without sacrificing performance.
By mastering these concepts, you can gain deeper insights into how your favorite dynamic languages work and even apply these techniques to your own projects.
Key Takeaways
Dynamic Languages: Offer flexibility by allowing variable types to change at runtime but require efficient handling of data types to maintain performance.
Tagged Unions: Provide a memory-efficient way to store different types in a single structure.
NaN Boxing: Optimizes memory usage by leveraging unused bits in floating-point numbers.
Pointer Tagging: Uses spare bits in pointers to store type information, further optimizing memory usage.
Real-World Implementations: Techniques like tagged unions and NaN boxing are used in interpreters for Lua, Python, and JavaScript.
Frequently Asked Questions (FAQs)
1. What is a dynamically typed language?
A dynamically typed language is one where the type of a variable is determined at runtime, allowing more flexibility but requiring efficient type management.
2. How do dynamic languages handle data types efficiently?
Dynamic languages use techniques like tagged unions, NaN boxing, and pointer tagging to efficiently store and manage data types in memory.
3. What is NaN boxing?
NaN boxing is a technique that uses the unused bits in a floating-point NaN value to store additional data, such as pointers or type tags.
4. What is a tagged union?
A tagged union is a data structure that stores only the data type currently in use, along with a tag to indicate the type, optimizing memory usage.
5. How does pointer tagging work?
Pointer tagging takes advantage of the alignment of pointers to store type information in the unused bits of the pointer value.
6. Why are these techniques important in dynamic languages?
These techniques help maintain the performance and efficiency of dynamic languages, which would otherwise suffer from the overhead of managing multiple data types at runtime.
7. Are these techniques used in real-world programming languages?
Yes, interpreters for languages like Lua, Python, and JavaScript use these techniques to optimize memory usage and execution speed.
8. What are the trade-offs of using these techniques?
The trade-offs include increased implementation complexity and potential architecture dependency, but the benefits in terms of memory efficiency and speed often outweigh these downsides.
Comments