Software development involves many stages, but one critical component is ensuring the code works as intended. This is where unit testing comes in. As one of the first testing methods applied during the development cycle, unit testing checks isolated pieces of code for correctness. Whether you are developing web applications, mobile apps, or large-scale enterprise software, unit testing is an essential practice that can drastically reduce bugs and improve code quality.
In this detailed guide, we’ll cover everything you need to know about unit testing, including its purpose, methods, benefits, and best practices.
What is Unit Testing?
Unit testing is a software testing method where individual components or small units of code are tested to ensure they work as intended. These "units" are the smallest testable parts of an application, such as functions, methods, or procedures. Unit testing is often automated and is typically performed by developers during the early stages of the development cycle to identify bugs before the code is integrated into larger systems.
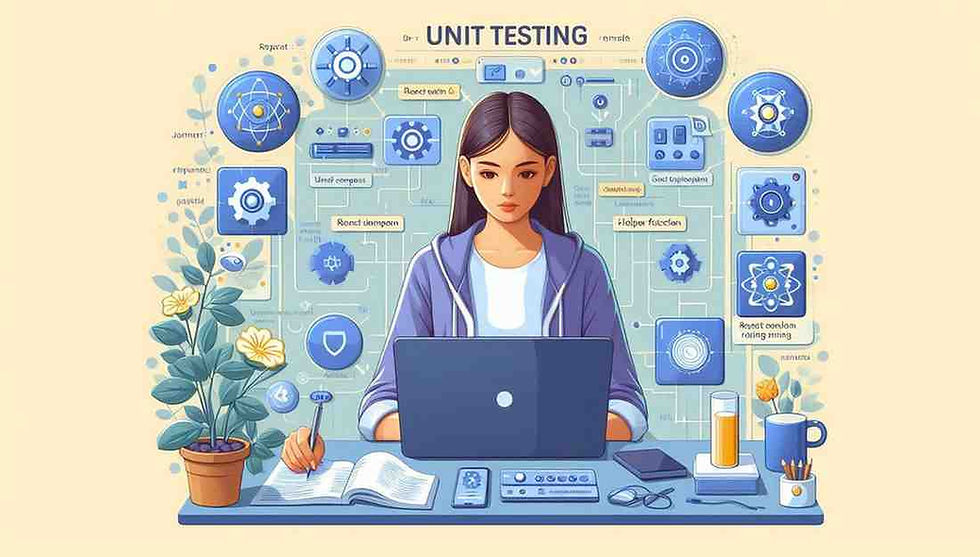
Key Characteristics of Unit Testing:
Focus on Smallest Units: Unit testing isolates individual pieces of code to test them independently.
Performed by Developers: Since unit testing involves knowledge of the code, it’s primarily carried out by developers.
Automated Testing: Unit tests are often written using testing frameworks that allow tests to be automated, ensuring they can be run efficiently throughout development.
Quick Feedback: Unit tests provide immediate feedback on whether a piece of code is functioning correctly.
Why Perform Unit Testing?
Unit testing serves multiple purposes within the software development lifecycle. It allows developers to verify that individual components of a system behave as expected, providing a level of assurance before the code is integrated into larger systems or released to users.
Key Benefits of Unit Testing:
Early Bug Detection: Unit testing helps catch bugs early, reducing the time and cost associated with fixing issues later in the development process.
Code Quality Improvement: By testing individual components, unit testing ensures that each part of the application functions correctly, leading to better overall software quality.
Facilitates Refactoring: With a solid suite of unit tests in place, developers can confidently refactor code knowing that the tests will catch any unintended consequences of their changes.
Reduces Defects in Later Stages: Since unit tests isolate and fix issues in the early stages, there are fewer bugs during integration, system, and acceptance testing.
Documentation: Unit tests act as a form of documentation, providing a clear specification of how the code should behave under different conditions.
What is Application Unit Testing?
Application Unit Testing involves verifying that individual units of an application function as expected. It is typically performed by developers during the software’s development phase. When a new function, method, or class is added to an application, unit testing ensures that it performs its intended role correctly.
Application unit testing offers a focused, isolated approach to verifying code logic without involving external systems such as databases, file systems, or APIs. Developers often use parameterized tests to run the same unit test with different input values, ensuring the robustness of their code.
Unit Testing Techniques
There are several techniques for conducting unit testing, each designed to test different aspects of the software code.
1. Structural Testing (White Box Testing)
Structural testing focuses on the internal structure of the code, examining how it accomplishes its goals rather than what it does. Developers test each line, branch, and path of the code to ensure full coverage.
Example: Testing all execution paths in a login function to ensure each possible outcome (success, failure, error) is covered.
2. Functional Testing
Functional testing evaluates whether a specific function or feature of the software works according to its requirements. It focuses on the output of the function, given a particular input.
Example: Testing whether a function that calculates the sum of two numbers returns the correct result for all possible valid and invalid inputs.
3. Error-Based Testing
This technique involves testing the code based on expected errors or mistakes that could occur during execution. It anticipates common mistakes or faults that developers might make and tests for them.
Examples of Error-Based Techniques:
Historical Test Data: Uses data from previous test runs to determine which test cases are more likely to expose errors.
Mutation Testing: Introduces small changes to the code (mutations) to check if the tests can detect errors.
Fault Seeding: Intentionally introduces known bugs into the code to ensure that the tests can detect them.
Types of Unit Testing
Unit testing can be broken down into different types based on what is being tested and how the tests are performed.
1. Manual Unit Testing
Manual unit testing involves developers writing tests and manually executing them. This can be useful for simple or one-off tests, but it becomes inefficient for larger projects or frequent code changes.
2. Automated Unit Testing
Automated unit testing is more efficient and scalable. Developers use testing frameworks such as JUnit (Java), pytest (Python), or Jest (JavaScript) to automate the execution of test cases. Automated tests can be run repeatedly, ensuring consistent results as the code evolves.
Introduction to Test-Driven Development (TDD)
Test-Driven Development (TDD) is a software development methodology where tests are written before the actual code. The TDD process follows a cycle where a developer writes a test, writes the code to pass that test, and then refines the code. This ensures that code is tested from the very beginning, preventing errors from creeping into the system.
TDD Cycle:
Write a Test: Write a unit test that defines a desired function or improvement.
Write Code: Write the minimal amount of code necessary to pass the test.
Refactor: Refine the code to improve its structure and efficiency without changing its behavior.
Repeat: Continue the process for each feature or bug fix.
The relationship between TDD and unit testing is clear—TDD relies heavily on automated unit tests to ensure code correctness at every step.
Best Practices for Unit Testing
1. Write Tests Early in Development
Unit tests should be written alongside or immediately after writing the code they test. Writing tests early ensures that issues are caught quickly and encourages better design practices.
2. Keep Unit Tests Isolated
Unit tests should focus on small, isolated pieces of code. Avoid testing multiple units or depending on external systems like databases, files, or APIs. Isolation ensures that tests are reliable and run quickly.
3. Avoid Logic in Tests
Tests should focus on expected outcomes rather than implementing complex logic. Including conditional statements (e.g., if, while) in tests makes them harder to understand and more prone to errors.
4. Don’t Let Tests Depend on Each Other
Unit tests should be independent of each other. Test interdependencies can cause tests to behave unpredictably and make debugging difficult. Ensure that each test has its own setup and teardown process.
5. Use Parameterized Tests
Parameterized tests allow developers to run the same test case with multiple sets of data, reducing code duplication and improving test coverage.
Unit Testing Frameworks
Several frameworks help developers automate and manage unit tests across different programming languages. Here are a few popular frameworks:
1. JUnit (Java)
JUnit is one of the most widely used frameworks for testing Java applications. It supports test automation and integrates with development tools like Eclipse and IntelliJ IDEA.
2. pytest (Python)
pytest is a flexible framework for testing Python code. It supports fixtures, parameterized tests, and detailed assertions, making it a popular choice among Python developers.
3. Jest (JavaScript)
Jest is a JavaScript testing framework that works well with modern frameworks like React, Angular, and Vue.js. It provides fast test execution and a great developer experience.
4. NUnit (.NET)
NUnit is a unit-testing framework for .NET applications. Like JUnit, it supports parameterized tests and is widely used in the .NET community.
5. Mocha (Node.js)
Mocha is a flexible JavaScript testing framework running on Node.js. It’s frequently paired with assertion libraries like Chai for behavior-driven testing.
How to Perform Unit Testing with Selenium
While Selenium is typically used for functional and UI testing, it can also be integrated with unit testing frameworks to automate unit tests. Selenium tests can be run using unit testing frameworks like JUnit or NUnit, and executed in real user environments through platforms like BrowserStack.
For instance, with BrowserStack Automate, developers can test their web applications on a wide variety of browsers and devices, ensuring that the code works as intended across different environments.
Common Challenges in Unit Testing
Despite its benefits, unit testing presents some challenges:
Mocking Dependencies: Unit tests require external systems like databases and APIs to be "mocked" or simulated, which can be complex.
Overhead in Writing Tests: Writing comprehensive tests takes time and can increase development effort, especially if test cases are not written early.
Ensuring Test Coverage: Achieving high test coverage can be challenging. It's important to balance thorough testing with practical constraints like time and complexity.
FAQs About Unit Testing
1. What is unit testing?
Unit testing is the process of testing individual units or components of a software application to ensure they function correctly.
2. Why is unit testing important?
Unit testing helps identify bugs early, improves code quality, and facilitates easier code refactoring by providing immediate feedback on the correctness of individual components.
3. What are some common unit testing frameworks?
Popular unit testing frameworks include JUnit (Java), pytest (Python), Jest (JavaScript), and NUnit (.NET).
4. How is unit testing different from integration testing?
Unit testing focuses on testing individual components in isolation, while integration testing checks how different components work together.
5. What is Test-Driven Development (TDD)?
Test-Driven Development (TDD) is a software development approach where tests are written before the actual code. Developers write code that passes the tests and refactor it to improve structure.
6. How often should unit tests be run?
Unit tests should be run frequently, ideally during every code change, to ensure that new bugs are not introduced into the codebase.
7. What are some best practices for unit testing?
Best practices for unit testing include writing tests early in development, avoiding logic in tests, using parameterized tests, and keeping tests independent of each other.
8. How does unit testing help with refactoring?
Unit tests ensure that existing functionality remains intact when developers refactor code, providing a safety net for changes.
Conclusion
Unit testing is an essential part of the software development process, helping developers ensure that individual components of an application function as expected. It improves code quality, facilitates early bug detection, and serves as a safeguard when refactoring code. By incorporating unit testing into your development practices and following best practices, you can significantly reduce the risk of defects in your software.
With the right frameworks and tools, such as JUnit, pytest, and Jest, unit testing can be automated to provide quick and reliable feedback throughout the development process. The key to successful unit testing lies in writing clear, isolated, and maintainable tests from the very beginning of the project.
Key Takeaways
Unit testing isolates and tests individual components of the software, ensuring they function as intended.
It helps detect bugs early in the development cycle, reducing costs and improving code quality.
Popular unit testing frameworks include JUnit, pytest, Jest, NUnit, and Mocha.
Test-Driven Development (TDD) is a methodology that uses unit tests to drive the development process.
Best practices for unit testing include writing tests early, keeping tests isolated, avoiding logic in tests, and using parameterized tests.
Comments