Introduction to Java Dictionaries
In the world of Java programming, managing data efficiently is paramount. Java dictionaries offer a robust solution for storing and managing key-value pairs, making them an indispensable tool for developers. Whether you're creating a simple phonebook application or handling complex data structures, understanding Java dictionaries can significantly enhance your coding skills and project outcomes.
Dictionaries in Java provide a way to associate unique keys with specific values, allowing for quick and efficient data retrieval. This guide explores the various ways to implement dictionaries in Java, focusing on the java.util.Dictionary class and its modern alternatives, such as HashMap and Hashtable.
Understanding the Dictionary Data Structure
Definition and Purpose
A dictionary, also known as a map or associative array, is a data structure that stores data in key-value pairs. Each key is unique and is used to retrieve its corresponding value. This structure is particularly useful for situations where data needs to be accessed quickly and efficiently based on a specific identifier.
Real-World Applications
Dictionaries are widely used in various applications, including:
Telephone Directories: Mapping names (keys) to phone numbers (values).
Configuration Settings: Storing settings where each configuration parameter (key) has a corresponding value.
Language Translation: Mapping words in one language (keys) to their translations in another language (values).
Java Dictionary Class: An Overview
History and Evolution
The java.util.Dictionary class is one of the original components of the Java Collections Framework, introduced in Java 1.0. It provides a way to store and manage key-value pairs, but it has largely been replaced by the Map interface and its implementations, such as HashMap and Hashtable.
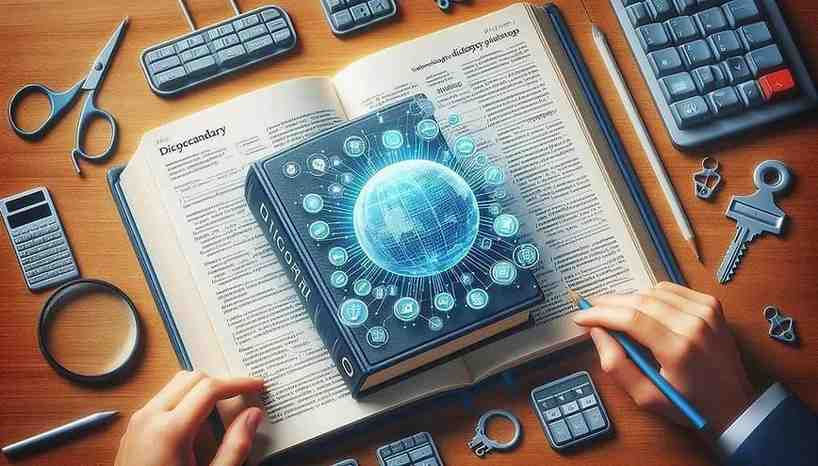
Key Features and Methods
The java.util.Dictionary class includes several essential methods:
get(Object key): Retrieves the value associated with the specified key.
put(Object key, Object value): Adds or updates a key-value pair.
remove(Object key): Removes the key-value pair associated with the specified key.
size(): Returns the number of key-value pairs in the dictionary.
isEmpty(): Checks if the dictionary is empty.
keys(): Returns an enumeration of the keys.
elements(): Returns an enumeration of the values.
Creating a Dictionary in Java
Using java.util.Dictionary
The java.util.Dictionary class is abstract, so you cannot instantiate it directly. Instead, you can use its concrete subclass, Hashtable, to create a dictionary.
java:
import java.util.Dictionary;
import java.util.Hashtable;
public class DictionaryExample {
public static void main(String[] args) {
Dictionary<String, Integer> dictionary = new Hashtable<>();
dictionary.put("Alice", 25);
dictionary.put("Bob", 30);
dictionary.put("Charlie", 35);
System.out.println("Bob's age: " + dictionary.get("Bob")); // 30
}
}
Implementing java.util.Hashtable
Hashtable extends Dictionary and implements the Map interface. It stores key-value pairs in a hash table, ensuring that each key is unique and mapped to one value.
java:
import java.util.Hashtable;
public class HashtableExample {
public static void main(String[] args) {
Hashtable<String, String> phoneBook = new Hashtable<>();
phoneBook.put("John Doe", "555-1234");
phoneBook.put("Jane Smith", "555-5678");
System.out.println("Jane's number: " + phoneBook.get("Jane Smith"));
}
}
HashMap as a Java Dictionary
Advantages Over Hashtable
HashMap is a more modern and flexible implementation of a dictionary in Java. Unlike Hashtable, HashMap allows null keys and values and is not synchronized, which makes it more efficient in environments where thread safety is not a concern.
java:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
Map<String, String> phoneBook = new HashMap<>();
phoneBook.put("John Doe", "555-1234");
phoneBook.put("Jane Smith", "555-5678");
System.out.println("John's number: " + phoneBook.get("John Doe"));
}
}
Code Examples and Usage
Using HashMap provides flexibility and better performance in non-thread-safe contexts. Here's how you can create and manipulate a HashMap:
java:
import java.util.HashMap;
import java.util.Map;
public class DictionaryDemo {
public static void main(String[] args) {
Map<String, Integer> ageMap = new HashMap<>();
ageMap.put("Alice", 25);
ageMap.put("Bob", 30);
ageMap.put("Charlie", 35);
System.out.println("Alice's age: " + ageMap.get("Alice"));
System.out.println("Is the map empty? " + ageMap.isEmpty());
System.out.println("Number of entries: " + ageMap.size());
}
}
Java Dictionary Class vs. HashMap
Key Differences
Thread Safety: Hashtable is synchronized and thread-safe, while HashMap is not.
Null Handling: HashMap allows null keys and values, Hashtable does not.
Performance: HashMap generally offers better performance due to the lack of synchronization overhead.
Use Cases and Best Practices
Use Hashtable: When you need a thread-safe implementation and null values are not required.
Use HashMap: When you need better performance and can manage thread safety independently.
Implementing a Telephone Book Using a Dictionary
Step-by-Step Guide
Creating a telephone book using a dictionary involves storing names as keys and phone numbers as values. You can use either Hashtable or HashMap for this purpose. Here's an example using HashMap:
java:
import java.util.HashMap;
import java.util.Map;
public class TelephoneBook {
public static void main(String[] args) {
Map<String, String> phoneBook = new HashMap<>();
phoneBook.put("John Doe", "555-1234");
phoneBook.put("Jane Smith", "555-5678");
phoneBook.put("Sam Brown", "555-9876");
System.out.println("Jane's number: " + phoneBook.get("Jane Smith"));
System.out.println("All entries: " + phoneBook);
}
}
Example Code and Output
The above code demonstrates how to create and use a simple telephone book. The output will be:
mathematica:
Jane's number: 555-5678
All entries: {John Doe=555-1234, Jane Smith=555-5678, Sam Brown=555-9876}
Handling Null Values in Java Dictionaries
Hashtable vs. HashMap
Hashtable does not allow null keys or values, whereas HashMap does. This distinction is crucial when deciding which implementation to use, especially in applications where null handling is important.
Best Practices for Null Handling
Use HashMap: When you need to handle null values for keys or values.
Avoid Hashtable: If null handling is a requirement in your application.
Performance Considerations for Java Dictionaries
Synchronization in Hashtable
The synchronization in Hashtable ensures thread safety but can lead to performance bottlenecks in multi-threaded applications.
Efficiency of HashMap
HashMap is generally more efficient due to its lack of synchronization overhead. It is the preferred choice for single-threaded environments or when thread safety can be managed externally.
Common Use Cases for Java Dictionaries
Data Storage and Retrieval
Dictionaries are commonly used for scenarios where data needs to be stored and retrieved efficiently based on unique keys. Examples include:
Configuration Settings: Storing application settings where each setting has a unique key.
Caching: Storing frequently accessed data to reduce database or computation overhead.
Real-World Examples
Language Translation: Mapping words or phrases from one language to another.
User Data Storage: Associating user IDs with user profiles or settings in an application.
Key Takeaways
Versatility: Java dictionaries, including Hashtable and HashMap, provide versatile solutions for key-value data management.
Performance: HashMap offers better performance and flexibility compared to Hashtable, especially in non-thread-safe environments.
Legacy Support: Hashtable provides legacy support but is generally considered obsolete in favor of more modern Map implementations.
Use Cases: Dictionaries are ideal for scenarios requiring efficient data retrieval based on unique keys.
Best Practices: Choose HashMap for flexibility and performance, and Hashtable for thread-safe, legacy applications.
Null Handling: Use HashMap if your application needs to handle null keys or values.
Conclusion: Mastering Java Dictionaries
Java dictionaries provide a powerful and efficient way to manage key-value pairs, making them essential for a wide range of applications. While the Dictionary class itself is considered obsolete, understanding its legacy role and modern alternatives like HashMap and Hashtable can greatly enhance your ability to work with Java data structures.
By mastering Java dictionaries, you can efficiently handle data storage and retrieval in your applications, ensuring that your code is both effective and maintainable. Whether you're dealing with configuration settings, caching, or complex data mappings, the knowledge of Java dictionaries will serve you well in your programming endeavors.
FAQs About Java Dictionaries
What is the difference between Hashtable and HashMap?
Hashtable is synchronized and does not allow null keys or values, making it thread-safe. HashMap, on the other hand, is not synchronized, allows null keys and values, and generally offers better performance.
Why is the Dictionary class considered obsolete?
The Dictionary class is considered obsolete because it was part of the original Java Collections Framework and does not implement the Map interface, limiting its integration with modern collections.
Can I use a Dictionary to store null values?
Hashtable does not allow null values for keys or values, while HashMap permits both.
When should I use a HashMap over a Hashtable?
Use a HashMap when you need a more flexible and performant solution without the need for built-in synchronization. Opt for Hashtable if you require thread safety and are working with legacy code.
How do I create a dictionary in Java?
You can create a dictionary using the Hashtable class for thread-safe, legacy support, or HashMap for modern applications. Use java.util.Dictionary only for understanding legacy code as it is abstract and obsolete.
What are the common applications of Java dictionaries?
Java dictionaries are commonly used for storing configuration settings, caching, language translation, and user data storage.
Are Java dictionaries thread-safe?
Hashtable is thread-safe due to its synchronized methods, while HashMap is not thread-safe by default. External synchronization is required if HashMap is used in a multi-threaded environment.
What is the primary benefit of using a dictionary data structure?
The primary benefit of using a dictionary data structure is the efficient storage and retrieval of data based on unique keys, making it ideal for applications requiring quick data access.
Comments