Java RESTful Services: Guide for Modern API Development
- Gunashree RS
- 5 hours ago
- 13 min read
Introduction to Java RESTful Services
In today's interconnected digital landscape, RESTful services have become the backbone of modern web applications. Java, with its robust ecosystem and enterprise capabilities, remains one of the most popular programming languages for implementing RESTful services. Whether you're building a microservices architecture, mobile application backends, or integrating disparate systems, understanding Java RESTful services is essential for any backend developer.
REST (Representational State Transfer) is an architectural style that defines a set of constraints for creating web services. It was introduced by Roy Fielding in his doctoral dissertation in 2000 and has since become the industry standard for API development. RESTful services are stateless, scalable, and provide a uniform interface, making them ideal for the diverse and distributed nature of modern applications.
Java offers several frameworks and specifications that simplify the development of RESTful services. From the standard JAX-RS (Java API for RESTful Web Services) specification to powerful frameworks like Spring Boot, the Java ecosystem provides comprehensive tools for building, testing, and deploying REST APIs.
In this guide, we'll explore the fundamentals of RESTful services in Java, examine the popular implementation approaches, and provide practical examples to help you master this essential technology. Whether you're new to REST or looking to enhance your existing knowledge, this article will serve as your comprehensive resource for Java RESTful services.
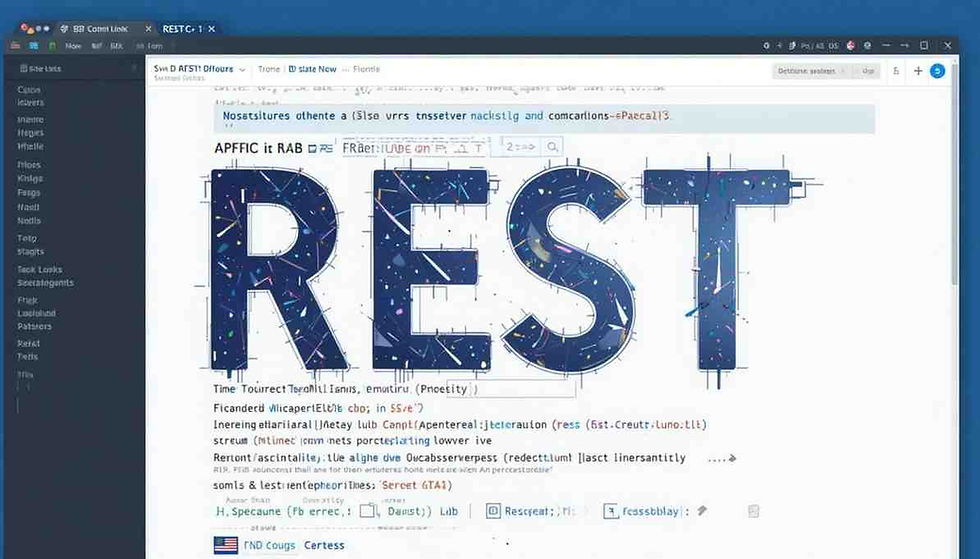
Understanding REST Architecture Principles
Before diving into Java implementations, it's crucial to understand the core principles that define RESTful architecture. These principles govern how RESTful services should be designed and implemented, regardless of the programming language used.
Key Constraints of REST Architecture
REST is defined by six architectural constraints that guide the development of RESTful services:
Client-Server Architecture: Separates user interface concerns from data storage concerns, improving portability of the user interface and scalability of the server components.
Statelessness: Each request from the client to server must contain all the information needed to understand and complete the request. The server doesn't store any client context between requests.
Cacheability: Responses must explicitly indicate whether they can be cached, improving network efficiency and performance.
Uniform Interface: Simplifies and decouples the architecture, allowing each part to evolve independently. The uniform interface includes:
Resource identification in requests
Resource manipulation through representations
Self-descriptive messages
Hypermedia as the engine of application state (HATEOAS)
Layered System: Constrains component behavior so that each component cannot "see" beyond the immediate layer they are interacting with.
Code on Demand (optional): Allows client functionality to be extended by downloading and executing code in the form of applets or scripts.
Resources and Representations
In REST, everything is considered a resource, which can be any entity or concept that needs to be exposed to the outside world. Resources are identified by URIs (Uniform Resource Identifiers), and clients interact with these resources through their representations.
A representation is the current or intended state of a resource, typically in formats like JSON or XML. When a client requests a resource, the server sends a representation of that resource's state, not the resource itself.
HTTP Methods in RESTful Services
RESTful services rely heavily on HTTP methods to indicate the desired action on a resource:
HTTP Method | CRUD Operation | Description |
GET | Read | Retrieves a resource or a collection of resources |
POST | Create | Creates a new resource |
PUT | Update | Updates an existing resource completely |
PATCH | Update | Updates an existing resource partially |
DELETE | Delete | Removes a resource |
HEAD | - | Similar to GET but returns only headers, no body |
OPTIONS | - | Returns supported HTTP methods for a resource |
REST Maturity Model (Richardson Maturity Model)
The Richardson Maturity Model helps in understanding the levels of REST API implementation:
Level 0: Uses HTTP as a transport system for remote interactions but doesn't utilize other web mechanisms.
Level 1: Introduces resources as core concepts.
Level 2: Implements HTTP verbs properly.
Level 3: Implements HATEOAS (Hypermedia as the Engine of Application State).
Most modern RESTful services aim to reach at least Level 2, with Level 3 being the goal for truly RESTful APIs.
JAX-RS: Java's Standard for RESTful Services
JAX-RS (Java API for RESTful Web Services) is the Java EE standard specification for implementing RESTful services. It provides annotations and interfaces that simplify the development of RESTful web services in Java.
Core JAX-RS Annotations
JAX-RS uses annotations to map Java classes and methods to REST resources and operations:
@Path: Specifies the relative path for a resource class or method.
@GET, @POST, @PUT, @DELETE, @HEAD: HTTP method designators for resource methods.
@Produces: Defines the MIME types that a resource method can produce.
@Consumes: Defines the MIME types that a resource method can consume.
@PathParam: Extracts values from the request URI path.
@QueryParam: Extracts values from the query component of the request URI.
@HeaderParam: Extracts values from HTTP headers.
@FormParam: Extracts values from form parameters in the request entity body.
@Context: Injects instances of supported resource contexts.
Simple JAX-RS Example
Here's a basic example of a JAX-RS resource class:
java
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import java.util.ArrayList;
import java.util.List;
@Path("/books")
public class BookResource {
private static List<Book> books = new ArrayList<>();
static {
books.add(new Book(1, "Effective Java", "Joshua Bloch"));
books.add(new Book(2, "Clean Code", "Robert C. Martin"));
}
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<Book> getBooks() {
return books;
}
@GET
@Path("/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Book getBook(@PathParam("id") int id) {
Return books.stream()
.filter(b -> b.getId() == id)
.findFirst()
.orElseThrow(() -> new NotFoundException("Book not found"));
}
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Book addBook(Book book) {
books.add(book);
return book;
}
@PUT
@Path("/{id}")
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Book updateBook(@PathParam("id") int id, Book updatedBook) {
Book book = getBook(id);
book.setTitle(updatedBook.getTitle());
book.setAuthor(updatedBook.getAuthor());
return book;
}
@DELETE
@Path("/{id}")
public void deleteBook(@PathParam("id") int id) {
Book book = getBook(id);
books.remove(book);
}
}
JAX-RS Implementations
Several frameworks implement the JAX-RS specification:
Jersey: The reference implementation of JAX-RS, developed by Oracle.
RESTEasy: JBoss's implementation of JAX-RS.
Apache CXF: A web services framework that includes a JAX-RS implementation.
Restlet: One of the earliest REST frameworks for Java that also supports JAX-RS.
Spring Framework for RESTful Services
While JAX-RS is the Java EE standard, the Spring Framework offers its approach to RESTful service development that's widely used in the industry. Spring provides a rich set of features for building RESTful services, especially through its Spring MVC and Spring Boot projects.
Spring MVC for REST
Spring MVC (Model-View-Controller) is a powerful web framework that can be easily configured to build RESTful services. It uses annotations to map HTTP requests to controller methods:
@RestController: Combines @Controller and @ResponseBody, indicating that the return values should be bound to the web response body.
@RequestMapping: Maps HTTP requests to handler methods.
@GetMapping, @PostMapping, @PutMapping, @DeleteMapping, @PatchMapping: Specialized variants of @RequestMapping for specific HTTP methods.
@RequestBody: Binds the HTTP request body to a method parameter.
@ResponseBody: Indicates that the return value should be bound to the web response body.
@PathVariable: Binds a method parameter to a URI template variable.
@RequestParam: Binds a method parameter to a web request parameter.
Spring Boot for Simplified REST Development
Spring Boot simplifies the development of RESTful services by providing auto-configuration and a standalone environment. It eliminates much of the boilerplate configuration required in traditional Spring applications.
Here's a simple Spring Boot REST controller example:
java
import org.springframework.web.bind.annotation.*;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
@RestController
@RequestMapping("/api/books")
public class BookController {
private final List<Book> books = new ArrayList<>();
private final AtomicInteger idGenerator = new AtomicInteger(1);
public BookController() {
books.add(new Book(idGenerator.getAndIncrement(), "Effective Java", "Joshua Bloch"));
books.add(new Book(idGenerator.getAndIncrement(), "Clean Code", "Robert C. Martin"));
}
@GetMapping
public List<Book> getAllBooks() {
return books;
}
@GetMapping("/{id}")
public Book getBookById(@PathVariable int id) {
return books.stream() .filter(book -> book.getId() == id)
.findFirst()
.orElseThrow(() -> new BookNotFoundException("Book not found with id: " + id));
}
@PostMapping
public Book createBook(@RequestBody Book book) {
book.setId(idGenerator.getAndIncrement());
books.add(book);
return book;
}
@PutMapping("/{id}")
public Book updateBook(@PathVariable int id, @RequestBody Book updatedBook) {
Book book = getBookById(id);
book.setTitle(updatedBook.getTitle());
book.setAuthor(updatedBook.getAuthor());
return book;
}
@DeleteMapping("/{id}")
public void deleteBook(@PathVariable int id) {
Book book = getBookById(id);
books.remove(book);
}
}
Spring Data REST
Spring Data REST is another powerful tool in the Spring ecosystem that automatically exposes Spring Data repositories as RESTful services with minimal coding. It creates a complete hypermedia-driven REST API based on your domain model and repository definitions.
java
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.rest.core.annotation.RepositoryRestResource;
@RepositoryRestResource(path = "books")public interface BookRepository extends JpaRepository<Book, Long> {
// Spring Data REST automatically creates CRUD endpoints
// No additional code needed!
}
Best Practices for Java RESTful Services
Implementing RESTful services in Java goes beyond just understanding the frameworks. Adhering to best practices ensures your APIs are robust, maintainable, and user-friendly.
Versioning Your API
API versioning is crucial for maintaining backward compatibility while allowing for evolution:
URI Path Versioning: /api/v1/books, /api/v2/books
Query Parameter Versioning: /api/books?version=1
Header Versioning: Using custom headers like X-API-Version: 1
Accept Header Versioning: Using the Accept header like Accept: application/vnd.company.app-v1+json
Proper HTTP Status Codes
Using appropriate HTTP status codes improves API clarity:
2xx (Success)
200 OK: Standard response for successful requests
201 Created: Resource successfully created
204 No Content: Request succeeded, but no content to return
4xx (Client Error)
400 Bad Request: Malformed request syntax
401 Unauthorized: Authentication required
403 Forbidden: Server understood but refuses to authorize
404 Not Found: Resource not found
405 Method Not Allowed: Method not allowed for the resource
409 Conflict: Request conflicts with the current state of the server
5xx (Server Error)
500 Internal Server Error: Generic server error
503 Service Unavailable: Server temporarily unavailable
Error Handling
Consistent error handling provides clear feedback to API consumers:
java
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
ErrorResponse error = new ErrorResponse(
HttpStatus.NOT_FOUND.value(),
ex.getMessage(),
System.currentTimeMillis()
);
return new ResponseEntity<>(error, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleGeneralException(Exception ex) {
ErrorResponse error = new ErrorResponse(
HttpStatus.INTERNAL_SERVER_ERROR.value(),
"An unexpected error occurred",
System.currentTimeMillis()
);
return new ResponseEntity<>(error, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Pagination, Filtering, and Sorting
For collections, implement mechanisms to manage large datasets:
java
@GetMapping("/books")
public Page<Book> getBooks(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size,
@RequestParam(defaultValue = "id") String sortBy,
@RequestParam(required = false) String title) {
Pageable pageable = PageRequest.of(page, size, Sort.by(sortBy));
if (title != null) {
return bookRepository.findByTitleContaining(title, pageable);
}
return bookRepository.findAll(pageable);
}
Security Considerations
Securing RESTful services is paramount:
Authentication: Implement JWT, OAuth 2.0, or API keys.
Authorization: Use role-based or attribute-based access control.
HTTPS: Always use HTTPS in production.
Input Validation: Validate all client inputs to prevent injection attacks.
Rate Limiting: Protect against DoS attacks by limiting request frequency.
Testing Java RESTful Services
Comprehensive testing ensures your RESTful services function correctly and reliably:
Unit Testing Controllers
java
@WebMvcTest(BookController.class)
public class BookControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private BookService bookService;
@Test
public void testGetBookById() throws Exception {
Book book = new Book(1, "Test Book", "Test Author");
when(bookService.findById(1)).thenReturn(book);
mockMvc.perform(get("/api/books/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.id").value(1))
.andExpect(jsonPath("$.title").value("Test Book"))
.andExpect(jsonPath("$.author").value("Test Author"));
}
}
Integration Testing
java
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class BookIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testCreateBook() {
Book newBook = new Book(null, "Integration Test Book", "Test Author"); ResponseEntity<Book> response = restTemplate.postForEntity("/api/books", newBook, Book.class);
assertEquals(HttpStatus.OK, response.getStatusCode());
assertNotNull(response.getBody().getId());
assertEquals("Integration Test Book", response.getBody().getTitle());
}
}
Performance Optimization for Java RESTful Services
Optimizing performance is critical for scalable RESTful services:
Caching Strategies
Implement caching to reduce load and improve response times:
java
@GetMapping("/{id}")
@Cacheable(value = "books", key = "#id")
public Book getBookById(@PathVariable int id) {
// This result will be cached
return bookRepository.findById(id).orElseThrow(() -> new BookNotFoundException(id));
}
@CacheEvict(value = "books", key = "#id")
@DeleteMapping("/{id}")
public void deleteBook(@PathVariable int id) {
// This will also clear the cache
bookRepository.deleteById(id);
}
Connection Pooling
Configure connection pooling for database access:
properties
# application.properties
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.idle-timeout=600000
spring.datasource.hikari.connection-timeout=30000
Asynchronous Processing
Use asynchronous processing for non-blocking operations:
java
@GetMapping("/async-books")
public CompletableFuture<List<Book>> getBookAsync() {
return CompletableFuture.supplyAsync(() -> {
// Potentially time-consuming operation
return bookService.findAllBooks();
});
}
Conclusion
Java RESTful services represent a powerful approach to building modern, scalable, and interoperable web applications. Whether you choose the standard JAX-RS approach or leverage the powerful features of Spring, Java provides a robust ecosystem for developing RESTful APIs.
The key to successful RESTful service implementation lies in understanding the core REST principles, following best practices, and selecting the right tools for your specific requirements. By adhering to REST constraints and utilizing the strengths of Java frameworks, you can create APIs that are maintainable, performant, and aligned with industry standards.
As the software industry continues to evolve towards microservices and distributed architectures, RESTful services in Java will remain a cornerstone technology for enterprise applications. By mastering these concepts and techniques, you'll be well-equipped to design and implement effective APIs that stand the test of time.
Key Takeaways
RESTful services follow architectural constraints, including client-server architecture, statelessness, cacheability, uniform interface, layered system, and code on demand (optional).
Java offers multiple frameworks for implementing RESTful services, with JAX-RS as the standard specification and Spring providing powerful alternatives.
HTTP methods (GET, POST, PUT, DELETE) form the backbone of REST API operations, mapping to CRUD operations.
Spring Boot simplifies RESTful service development with auto-configuration and a standalone environment.
Best practices include proper API versioning, appropriate HTTP status codes, consistent error handling, and implementing pagination.
Security considerations should include authentication, authorization, HTTPS, input validation, and rate limiting.
Comprehensive testing, both unit and integration, ensures RESTful services function correctly and reliably.
Performance optimization techniques include caching, connection pooling, and asynchronous processing.
The Richardson Maturity Model helps evaluate the RESTfulness of your API implementation.
Proper documentation is essential for API adoption and developer productivity.
Frequently Asked Questions (FAQ)
What is the difference between REST and SOAP web services?
REST is an architectural style that uses standard HTTP methods and typically exchanges data in lightweight formats like JSON. SOAP is a protocol that defines strict standards for message structure, often using XML, and can work over various transport protocols. REST services are generally simpler, more lightweight, and easier to consume, while SOAP offers more built-in standards for security and reliability.
Is JAX-RS or Spring better for RESTful services in Java?
Neither is universally "better" - they serve different needs. JAX-RS is the Java EE standard and provides a focused API specifically for RESTful services. Spring offers a more comprehensive ecosystem with additional features beyond REST. Spring Boot's auto-configuration makes development faster, while JAX-RS may be preferred in Java EE environments or when a more standardized approach is required.
How do I handle authentication in RESTful services?
Common authentication methods include JWT (JSON Web Tokens), OAuth 2.0, API keys, and Basic Authentication. For Spring-based applications, Spring Security provides comprehensive support for these mechanisms. The choice depends on your security requirements, with OAuth 2.0 being preferred for third-party API access and JWT for stateless authentication.
What is HATEOAS, and should I implement it?
HATEOAS (Hypermedia as the Engine of Application State) is a constraint where a client interacts with a REST application entirely through hypermedia provided dynamically by the server. It improves API discoverability and self-documentation but adds complexity. Whether to implement it depends on your API consumers' needs - it's beneficial for public APIs or when client applications are developed independently from the server.
How can I document my Java RESTful services?
Popular tools include Swagger/OpenAPI, SpringDoc, Spring REST Docs, and RAML. Swagger/OpenAPI is widely used and can generate interactive documentation from annotations in your code. Spring REST Docs takes a test-driven approach to documentation. The choice depends on your workflow preferences and how tightly you want documentation coupled with code.
How should I version my RESTful APIs?
Common strategies include URI path versioning (/api/v1/resource), query parameter versioning (/api/resource?version=1), custom header versioning (X-API-Version: 1), or content negotiation using Accept headers. URI path versioning is the most straightforward and widely adopted approach, though content negotiation is more RESTfully pure.
What's the best way to handle errors in RESTful services?
Use appropriate HTTP status codes combined with consistent error response bodies. Implement global exception handlers (like @ControllerAdvice in Spring) to centralize error handling. Error responses should include a status code, error message, timestamp, and potentially a unique error reference for troubleshooting.
How can I secure my Java RESTful services against common vulnerabilities?
Implement HTTPS, input validation, output encoding, CSRF protection, and proper authentication/authorization. Use security headers, implement rate limiting, and keep dependencies updated. For Spring applications, leverage Spring Security's features like CSRF protection, secure headers, and method-level security annotations. Regular security audits and penetration testing are also recommended.
Sources and Further Reading
Richardson, Leonard, and Sam Ruby. "RESTful Web Services." O'Reilly Media, 2007. https://www.oreilly.com/library/view/restful-web-services/9780596529260/
Oracle. "The Java EE Tutorial: Building RESTful Web Services with JAX-RS." https://docs.oracle.com/javaee/7/tutorial/jaxrs.htm
Spring Framework. "Building REST services with Spring." https://spring.io/guides/tutorials/rest/
Baeldung. "REST with Spring Tutorial." https://www.baeldung.com/rest-with-spring-series
Mozilla Developer Network. "HTTP response status codes." https://developer.mozilla.org/en-US/docs/Web/HTTP/Status
OWASP. "REST Security Cheat Sheet." https://cheatsheetseries.owasp.org/cheatsheets/REST_Security_Cheat_Sheet.html
Richardson, Leonard. "Richardson Maturity Model." https://martinfowler.com/articles/richardsonMaturityModel.html
Swagger. "OpenAPI Specification." https://swagger.io/specification/
Spring. "Spring Data REST Reference Guide." https://docs.spring.io/spring-data/rest/docs/current/reference/html/
Comments