JSONPath Escape: Mastering Advanced Filtering Techniques
- Gunashree RS
- 19 minutes ago
- 9 min read
Introduction to JSONPath and Escape Characters
In the world of modern application development and data handling, JSON (JavaScript Object Notation) has become the standard format for data interchange. As applications grow increasingly complex, the need to efficiently navigate and extract specific information from JSON data structures becomes critically important. This is where JSONPath comes in—a powerful query language specifically designed for JSON data.
JSONPath, inspired by XML's XPath, provides a way to navigate through JSON structures using expressions. However, when working with JSONPath, you'll inevitably encounter situations where you need to use special characters or reserved symbols.
This is where the concept of "JSONPath escape" becomes essential.
In this comprehensive guide, we'll dive deep into JSONPath escape techniques, exploring how to properly handle special characters, navigate complex JSON structures, and implement advanced filtering effectively. Whether you're a developer working with Kubernetes' kubectl commands, building data processing pipelines, or simply looking to enhance your JSON manipulation skills, understanding JSONPath escape will significantly improve your efficiency and code quality.
Understanding JSONPath Fundamentals
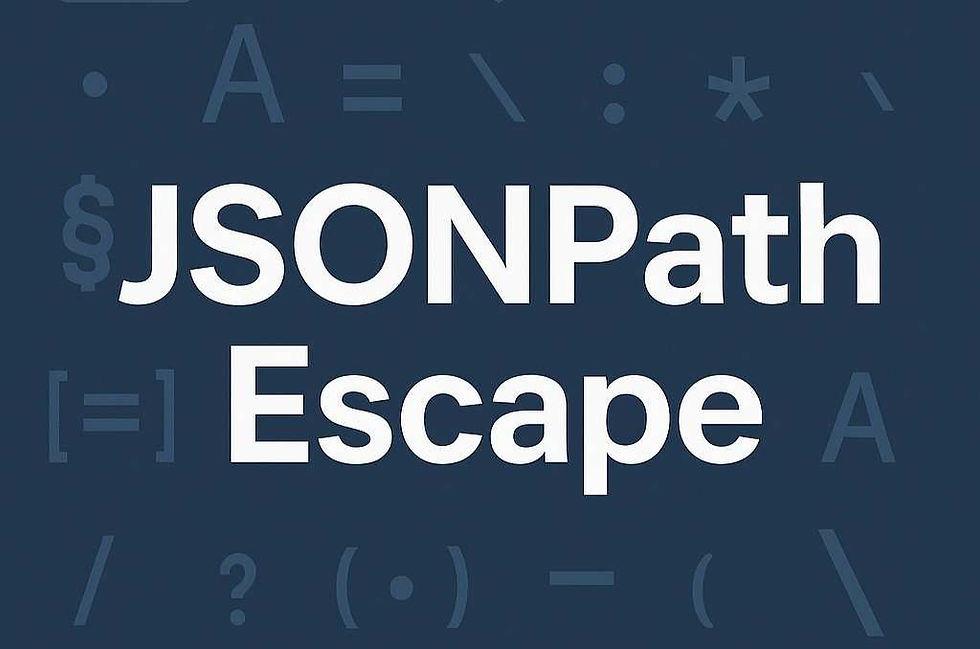
Before we can effectively use JSONPath escape techniques, we need to establish a solid understanding of JSONPath itself. JSONPath is a query language that allows you to select and extract data from a JSON document.
Basic JSONPath Syntax
JSONPath expressions use a path-like syntax to navigate through the elements of a JSON structure:
$ - Represents the root object/element
. - Child operator (selects a specific child element)
[] - Subscript operator (used for array access or filtering)
* - Wildcard (selects all elements regardless of their names)
.. - Recursive descent (searches for all matching descendants)
@ - Current element being processed (used in filters)
For example, given this JSON:
json
{
"store": {
"book": [
{
"title": "The Lord of the Rings",
"price": 22.99
},
{
"title": "Dune",
"price": 17.99
}
]
}
}
The JSONPath $.store.book[*].title would return:
"The Lord of the Rings"
"Dune"
Common JSONPath Use Cases
JSONPath has become essential in numerous scenarios, including:
Data extraction - Pulling specific values from complex JSON responses
Data transformation - Reshaping JSON data for different system requirements
Filtering - Selecting only elements that match certain criteria
Kubernetes management - Extracting specific information from kubectl output
API testing - Validating specific elements within API responses
Configuration management - Accessing nested configuration values programmatically
Understanding when and why you might need to escape characters in JSONPath queries becomes clearer when you grasp these common use cases.
When and Why to Use JSONPath Escape
JSONPath escape techniques become necessary when your expressions contain special characters or characters that have a specific meaning in JSONPath syntax. Escaping these characters ensures they're interpreted as literal values rather than as part of the JSONPath syntax.
Scenarios Requiring JSONPath Escaping
Several common scenarios require proper escaping:
Special characters in property names - When JSON properties contain spaces, dots, special symbols, or reserved JSONPath characters
Filtering on values with special characters - When filtering for strings containing quotes, backslashes, or other special characters
Working with dynamic property names - When property names are variables or come from user input
Nested filtering expressions - When complex filtering requires special syntax handling
Kubernetes resource management - When querying resources with special characters in names or annotations
Special Characters That Need Escaping
The following characters typically need special handling in JSONPath expressions:
Character | Meaning in JSONPath | How to Handle |
. | Child operator | Bracket notation or escape |
$ | Root element | Escape when used as a literal |
@ | Current element | Escape when used as a literal |
* | Wildcard | Escape when used as a literal |
[ and ] | Array or filter | Escape when used as a literal |
' and " | Quote strings | Escape or alternate quotes |
\ | Escape character | Double escape |
Spaces | Can break expressions | Bracket notation or escape |
Let's examine a practical example. If you have a JSON object with a property named "special.property", you can't simply use:
This would be interpreted as looking for "property" within "special". Instead, you'd need to use bracket notation with quotes:
$['special.property']
JSONPath Escape Techniques in Different Contexts
The specific techniques for escaping JSONPath expressions may vary depending on the context and tools you're using.
Escaping in Kubernetes kubectl Commands
When using JSONPath with Kubernetes' kubectl command, you need to handle multiple layers of interpretation:
Shell interpretation - Your shell may interpret certain characters before they reach kubectl
kubectl JSONPath interpretation - The JSONPath expression needs proper escaping for kubectl
JSON structure handling - The actual JSON data structure might contain special characters
For example, to get container images from all pods:
bash
kubectl get pods -o jsonpath='{.items[*].spec.containers[*].image}'
But what if you need to filter pods with names containing dots? You might need:
bash
kubectl get pods -o jsonpath='{.items[?(@.metadata.name=="app.backend")].status.phase}'
Notice the double quotes inside single quotes to handle the dot in the name.
Escaping in Programming Languages
Different programming languages have their own JSONPath libraries, each with slightly different escaping requirements:
JavaScript Example
javascript
const jsonpath = require('jsonpath');
const result = jsonpath.query(data, "$['property.with.dots']");
Python Example
python
import jsonpath_ng.ext as jsonpath
expression = jsonpath.parse("$['property.with.dots']")
result = [match.value for match in expression.find(data)]
Java Example
java
import com.jayway.jsonpath.JsonPath;
String result = JsonPath.read(json, "$['property.with.dots']");
Online Tools for JSONPath Escaping
Several online tools can help with proper JSONPath escaping:
JSONPath Evaluators - Allow you to test expressions against your data
JSON Escape Tools - Help prepare strings for inclusion in JSONPath expressions
Kubernetes JSONPath Testers - Specifically designed for kubectl JSONPath testing
These tools can be invaluable when working with complex expressions or troubleshooting JSONPath queries that aren't returning expected results.
Advanced JSONPath Filtering with Escaped Characters
One of the most powerful features of JSONPath is its filtering capability. Filtering becomes particularly complex when dealing with special characters that need escaping.
Filter Expression Syntax
In JSONPath, filter expressions use the following syntax:
[?(<expression>)]
Where <expression> is a Boolean expression that determines which elements to include.
Common Filter Operations
JSONPath supports various comparison operations:
== - Equal to
!= - Not equal to
>, >=, <, <= - Greater than, greater than or equal, less than, less than or equal
=~ - Regular expression match (in some implementations)
Escaping in Filter Expressions
When your filter criteria involve special characters, proper escaping becomes crucial:
Example 1: Filtering with Dot in Property Names
$[?(@['user.name'] == 'John')]
This finds elements where the property "user.name" equals "John".
Example 2: Escaping Quotes in Filter Values
$[?(@.message == 'Don\'t worry')]
or
$[?(@.message == "Don't worry")]
Both find elements where "message" equals "Don't worry".
Example 3: Complex Nested Filtering
$[?(@.metadata.annotations['kubernetes.io/description'] == 'Database pod')]
This finds Kubernetes resources with a specific annotation value.
Best Practices for JSONPath Escaping
To make your JSONPath expressions more reliable and maintainable, follow these best practices:
1. Prefer Bracket Notation for Special Characters
When dealing with property names containing special characters, bracket notation is clearer than trying to escape dots:
// Good
$['user.name']
// Less clear
$.user\.name // Note: This syntax isn't even supported in all implementations
2. Use Single Quotes Outside, Double Quotes Inside (or vice versa)
This approach avoids most escaping issues:
$[?(@.property == 'value with "quotes"')]
$[?(@.property == "value with 'quotes'")]
3. Test Expressions Incrementally
Build complex expressions step by step, testing each part:
Start with the basic path ($.store.book)
Add array access ($.store.book[*])
Add filtering ($.store.book[?(@.price > 10)])
Add complex conditions with escaped characters
4. Document Complex Expressions
When working with complex JSONPath expressions, especially those requiring escaping, add comments explaining the logic:
bash
# Find pods with annotations containing special characters
kubectl get pods -o jsonpath='{.items[?(@.metadata.annotations["special/annotation"] == "value")].metadata.name}'
5. Consider Alternative Approaches for Very Complex Queries
Sometimes, it's cleaner to:
Extract all data and filter programmatically
Use multiple simpler queries instead of one complex query
Use tools like jq that might have more intuitive syntax for complex cases
Common JSONPath Escape Pitfalls and Solutions
Even experienced developers encounter issues with JSONPath escaping. Here are some common problems and their solutions:
1. Multiple Levels of Escaping
Problem: When using JSONPath in command-line tools or scripts, you may need to escape characters for both the shell and JSONPath.
Solution: Use single quotes around the entire expression when possible, and double quotes around string values inside:
bash
kubectl get pods -o jsonpath='{.items[?(@.metadata.name=="example.com")].status.phase}'
2. Inconsistent Implementation Support
Problem: Different JSONPath implementations support different features and escaping mechanisms.
Solution:
Test your expressions with your specific implementation
Stick to widely supported features when possible
Document any implementation-specific quirks
3. Regular Expression Escaping
Problem: When using regex in JSONPath filters (if supported), you need extra escaping:
Solution: Be extra careful with backslashes in regex patterns, often requiring double or even triple escaping:
$[?(@.code =~ /^E\d+$/)] # May need to be written as:
$[?(@.code =~ /^E\\d+$/)]
4. Nested Quotes
Problem: Handling strings with both single and double quotes can be tricky.
Solution: Use backslash escaping consistently:
$[?(@.message == "He said: \"Don't worry\"")]
5. Reserved Words and Operators
Problem: Some words or symbols might have special meaning in JSONPath.
Solution: Use bracket notation and quotes:
$['and'].value # If 'and' is a property name that could be confused with an operator
Conclusion
JSONPath escape techniques are essential for effectively working with complex JSON data structures, especially when property names or values contain special characters. By understanding the fundamental principles of JSONPath, recognizing when escaping is necessary, and applying the appropriate techniques for your specific context, you can write more robust and precise data extraction expressions.
Whether you're working with Kubernetes resources, API responses, or any other JSON data, proper JSONPath escaping ensures your queries work as expected. Remember to test your expressions incrementally, leverage appropriate tools, and follow best practices to make your JSONPath expressions both powerful and maintainable.
As JSON continues to dominate as a data interchange format, mastering JSONPath and its escaping techniques will remain a valuable skill for developers, data engineers, and DevOps professionals. The time invested in understanding these concepts will pay dividends in more efficient data handling and fewer unexpected behaviors in your applications.
Key Takeaways
JSONPath is a powerful query language for extracting and filtering data from JSON structures.
JSONPath escape techniques are necessary when working with property names or values containing special characters.
Use bracket notation (['property.name']) instead of dot notation (.property.name) when properties contain special characters
In filter expressions, use alternating quote styles (single outside, double inside, or vice versa) to minimize escaping.
Different contexts (Kubernetes kubectl, programming languages, etc.) may require different escaping approaches.
Test complex expressions incrementally to isolate and resolve escaping issues.
Online tools can help validate JSONPath expressions and proper character escaping.
Documentation is crucial for complex JSONPath expressions, especially those with escaped characters.
Sometimes, simpler approaches or multiple simple queries are preferable to heavily escaped complex expressions.
Regular expression filtering requires special attention to escaping rules
FAQ About JSONPath Escape
What is the difference between JSONPath and XPath?
While both are query languages for navigating structured data, JSONPath is specifically designed for JSON, while XPath is for XML. JSONPath uses dot notation ($.store.book) and bracket notation ($['store']['book']), while XPath uses slash notation (/store/book). JSONPath was inspired by XPath but has a simpler syntax tailored to JSON's structure.
How do I handle spaces in property names in JSONPath?
Use bracket notation with quotes around the property name. For example, if your JSON has a property named "user name", use $['user name'] instead of $.user name (which would be invalid). This bracket notation approach works for any property names with special characters, not just spaces.
Can I use regular expressions in JSONPath filters?
Some JSONPath implementations support regular expressions using the ~ = operator, but support varies. For example: $[?(@.name =~ /^test.*/)]. When using regex, be aware that you may need additional escaping for special regex characters, especially backslashes.
How do I escape quotes within string values in JSONPath filters?
You have two options: use different quote types (single outside, double inside or vice versa), or escape with backslashes. For example: $[?(@.message == "Don't worry")] or $[?(@.message == 'Don't worry')]. The first approach is generally cleaner when possible.
Why is my kubectl JSONPath query not working?
Common issues include: shell interpretation of special characters (fix by using single quotes around the entire expression), incorrect JSONPath syntax, or trying to access properties that don't exist. Try building your query incrementally and test with a JSONPath evaluator. For kubectl specifically, remember that the expression must be enclosed in curly braces: -o jsonpath='{.items[*].metadata.name}'.
How do I access properties with numeric names in JSONPath?
Use bracket notation with quotes: $['123'] or $["123"]. This works for any property name but is specifically needed for numeric names since they can't be accessed with dot notation.
Can I combine multiple filter conditions in JSONPath?
Yes, you can use logical operators like && (AND) and || (OR) in filter expressions: $[?(@.price > 10 && @.category == 'fiction')]. When these expressions contain special characters, ensure proper escaping for each part of the condition.
How do I handle deeply nested properties with special characters?
Use bracket notation consistently at each level where special characters appear: $['user.info']['contact.details']['phone.number']. You can mix dot notation and bracket notation as needed: $.user['contact.details'].phone.
Sources for Further Reading
Kubernetes Documentation: JSONPath Support
FreeFormatter: JSON Escape / Unescape Tool
LambdaTest: JSON Escape Online Tool
JSONPath Online Evaluator: JSONPath Expression Tester
GitHub - JSONPath Plus: A Robust JSONPath Implementation
Medium Article: Mastering JSONPath for Kubernetes Resource Management
Newtonsoft JSON Documentation: JSONPath - Selective JSON Parsing
StackOverflow Question: JSONPath Escaping Special Characters
Jayway JsonPath GitHub: JsonPath Implementation for Java
Baeldung Tutorial: Guide to JSONPath with Java
Comments