Introduction
In the realm of software development, ensuring that your application can handle the demands of real-world usage is critical. Performance testing, specifically load testing, is key to validating that your system can manage expected user traffic and maintain responsiveness under stress. This is where k6 comes in—a powerful, open-source tool designed for performance and load testing.
In this comprehensive guide, we will dive deep into the world of k6 load tests, exploring how to set up and run load tests, interpret the results, and ultimately ensure that your website or API can perform reliably under pressure. Whether you’re a beginner or an experienced tester, this article will provide you with the knowledge and tools you need to master k6 load testing.
What is Load Testing?
Load testing is a type of performance testing used to evaluate how a system behaves under a specific expected load. The goal is to identify the system's capacity, measure its performance under varying conditions, and detect any bottlenecks or weaknesses. By simulating real-world traffic, load testing helps ensure that an application can handle the number of users, transactions, or data it will encounter in production.
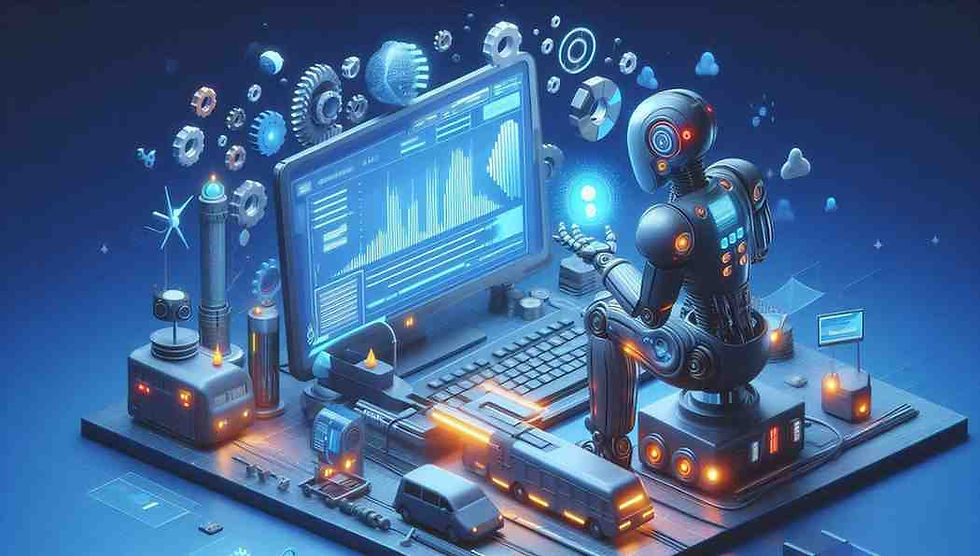
Key metrics in load testing include:
Response Time: How quickly the system responds to user requests.
Throughput: The number of transactions processed by the system in a given time.
Error Rate: The percentage of requests that fail under load.
Scalability: How well the system handles increasing loads.
Introduction to k6
k6 is an open-source load testing tool developed by Grafana Labs. It’s designed to be easy to use, yet powerful enough to handle complex load-testing scenarios. Written in Go and utilizing JavaScript (ES5.1+) for scripting, k6 offers a developer-centric approach to performance testing, making it a favorite among developers and QA engineers alike.
Key Features of k6
Scripting in JavaScript: k6 uses a familiar JavaScript syntax, making it accessible to developers.
High Performance: k6 is designed to handle large-scale load tests with minimal resource consumption.
Extensible and Scalable: It supports custom metrics, and integration with other tools, and can scale from local tests to distributed cloud testing.
Rich Reporting: k6 provides detailed metrics and insights, helping teams quickly identify performance issues.
Why Choose k6 for Load Testing?
k6 stands out in the crowded field of performance testing tools for several reasons:
Ease of Use: With a focus on simplicity, k6 allows users to create and run load tests with minimal setup.
Scriptable Tests: The use of JavaScript for scripting makes it easy to customize tests and add logic, making it ideal for complex scenarios.
Extensive Metrics: k6 provides detailed metrics out-of-the-box, including custom metrics, to give a comprehensive view of system performance.
CI/CD Integration: k6 integrates seamlessly with continuous integration and deployment pipelines, enabling automated performance testing as part of the development workflow.
Open Source and Community-Driven: Being open-source, k6 benefits from a vibrant community that contributes to its ongoing development and improvement.
Setting Up k6: Installation and Configuration
Before running your first k6 load test, you need to install and configure k6 on your machine. Here’s how to get started:
Installing k6
k6 can be installed on various operating systems, including Windows, macOS, and Linux. Below are the installation steps for each platform:
Windows
Download the k6 MSI installer from the official k6 website.
Run the installer and follow the on-screen instructions.
Verify the installation by running the k6 version in your command prompt.
macOS
Open your terminal and run the following command to install k6 using Homebrew:
bash
brew install k6
Verify the installation by running the k6 version in your terminal.
Linux
On Ubuntu or Debian, run the following commands to install k6:
bash
sudo apt update
sudo apt install k6
Verify the installation by running the k6 version in your terminal.
Alternatively, you can use Docker to run k6:
bash
docker pull grafana/k6
docker run -i grafana/k6 run - <script.js
Configuring k6
Once installed, you can customize k6 settings through a configuration file or directly in your test scripts. Common configurations include setting default values for virtual users (VUs), test duration, and custom thresholds.
Creating Your First k6 Load Test Script
Creating a k6 load test script is straightforward, thanks to its use of JavaScript for scripting. Here’s how to write and run a basic load test script:
Sample k6 Load Test Script
javascript
import http from 'k6/http';
import { check, sleep } from 'k6';
export let options = {
vus: 10,
duration: '30s',
};
export default function () {
let res = http.get('https://test-api.example.com');
check(res, {
'status is 200': (r) => r.status === 200,
});
sleep(1);
}
Explanation of the Script
Import Statements: The script begins by importing necessary modules (http, check, and sleep).
Options Object: The options object defines the number of virtual users (vus) and the duration of the test (duration).
Main Function: The default function runs the load test. It sends a GET request to the specified URL and checks if the response status is 200 (OK).
Sleep Function: The sleep(1) function pauses the virtual user for one second before the next iteration.
Running the Script
Save the script as script.js and run it using the following command:
bash
k6 run script.js
This command will execute the script with 10 virtual users over 30 seconds, simulating traffic to your website or API.
Running k6 Load Tests: Key Concepts
To effectively run k6 load tests, it’s essential to understand the key concepts that define the test’s behavior:
Virtual Users (VUs)
Virtual Users (VUs) simulate real users interacting with your application. The number of VUs determines the load on the system. For example, 10 VUs might represent 10 users simultaneously accessing your site.
Duration
The duration of a test defines how long the load test runs. It’s specified in time units (e.g., 30s for 30 seconds or 10m for 10 minutes).
Checks
Checks are assertions that verify the correctness of the response from the server. They ensure that the system behaves as expected under load. For instance, you might check that the status code returned is 200, indicating a successful request.
Thresholds
Thresholds are performance goals that define acceptable performance levels. If the system fails to meet a threshold (e.g., 95% of requests should be faster than 500ms), the test is considered a failure.
javascript
export let options = {
thresholds: {
http_req_duration: ['p(95)<500'], // 95% of requests must complete below 500ms
},
};
Analyzing k6 Load Test Results
After running a k6 load test, interpreting the results is crucial to understanding your system's performance. k6 provides detailed metrics, which can be categorized into HTTP metrics, checks, and custom metrics.
HTTP Metrics
k6 automatically collects several HTTP-related metrics:
http_req_duration: The time taken for an HTTP request to complete.
http_req_connecting: The time taken to establish a TCP connection.
http_req_tls_handshaking: The time taken for the TLS handshake.
http_req_waiting: The time spent waiting for the server to respond (a.k.a. Time to First Byte).
Checks and Thresholds
Checks and thresholds are critical for evaluating the test’s success. The results show whether your application meets the defined performance criteria.
Checks: Passed checks confirm that the system is returning correct responses (e.g., HTTP 200 status codes).
Thresholds: If a threshold is breached (e.g., 95th percentile request duration exceeds 500ms), the test fails.
Custom Metrics
k6 allows you to define custom metrics to track specific aspects of your system, such as the rate of successful logins or the number of items added to a shopping cart.
javascript
import { Counter } from 'k6/metrics';
let successfulLogins = new Counter('successful_logins');
export default function () {
let res = http.post('https://test-api.example.com/login', { username: 'test', password: '12345' });
if (res.status === 200) {
successfulLogins.add(1);
}
}
Advanced k6 Load Testing Scenarios
k6 supports various advanced load testing scenarios beyond basic load testing. These include stress testing, spike testing, soak testing, and breakpoint testing.
Stress Testing
Stress testing pushes the system to its limits to identify its maximum capacity and how it behaves when overloaded.
Spike Testing
Spike testing introduces sudden increases in load to observe how the system handles extreme, short-term spikes in traffic.
Soak Testing
Soak testing involves running a load test over an extended period to detect issues like memory leaks or performance degradation.
Breakpoint Testing
Breakpoint testing gradually increases the load until the system fails, identifying the exact breaking point of the application.
Best Practices for k6 Load Tests
To ensure effective load testing with k6, follow these best practices:
Start with Baseline Tests: Establish a baseline to compare future tests against.
Use Realistic Load: Simulate traffic patterns that mirror real-world usage.
Gradually Increase Load: Avoid overwhelming the system too quickly by gradually increasing the load.
Monitor System Resources: Track CPU, memory, and network usage on your servers during the test.
Analyze Results Thoroughly: Look beyond simple pass/fail results; analyze the data to understand system behavior.
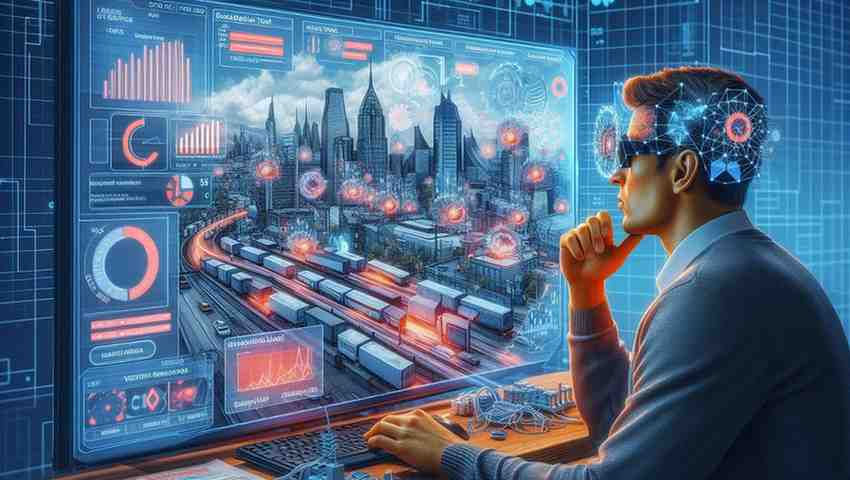
Integrating k6 with CI/CD Pipelines
Integrating k6 into your CI/CD pipeline automates performance testing, ensuring that every code change is tested for performance regressions. This can be achieved using tools like Jenkins, GitLab CI, or GitHub Actions.
Example: GitLab CI Integration
Here’s how you can integrate k6 with GitLab CI:
yaml
stages:
- performance
performance_test:
stage: performance
image: loadimpact/k6
script:
- k6 run script.js
This pipeline stage runs the k6 load test whenever the code is pushed, automatically verifying performance criteria.
Common Challenges and How to Overcome Them
Challenge 1: Handling Large-Scale Load Tests
For large-scale tests, running k6 locally might not be feasible due to resource constraints. Instead, consider using k6 Cloud or distributed testing setups to manage the load across multiple machines.
Challenge 2: Dealing with Network Latency
High network latency can skew results, especially in globally distributed systems. To mitigate this, run tests from geographically relevant locations or use CDN services to cache content closer to users.
Challenge 3: False Positives in Test Results
Sometimes, transient issues (e.g., network hiccups) can cause tests to fail even when the system is stable. Implement retry logic or run tests multiple times to ensure consistent results.
The Future of Load Testing with k6
As systems become more complex and distributed, the need for advanced load testing tools like k6 will continue to grow. Future developments in k6 might include deeper integrations with observability platforms, more sophisticated test scenarios, and enhanced support for microservices and serverless architectures.
With the rise of cloud-native and microservice architectures, load testing must adapt to ensure that these modern systems remain performant and reliable under load. k6 is well-positioned to meet these challenges, making it an essential tool for any performance engineering toolkit.
Conclusion
k6 load tests offer a powerful and flexible way to ensure your applications perform well under various conditions. By simulating real-world traffic and identifying potential bottlenecks, k6 helps you deliver fast, reliable, and scalable software. From setting up simple tests to running complex scenarios, k6 equips you with the tools to thoroughly evaluate your system’s performance and make informed decisions based on real data.
Whether you’re just starting with load testing or looking to enhance your existing processes, k6 provides the capabilities you need to ensure your applications can handle the demands of modern users.
Key Takeaways
k6 is a powerful, open-source load-testing tool designed for simplicity and performance.
Load testing with k6 helps ensure your system is reliable, responsive, and scalable under pressure.
Key concepts in k6 include virtual users, checks, thresholds, and custom metrics.
Advanced scenarios like stress, spike, soak, and breakpoint testing are easily implemented with k6.
Integrating k6 with CI/CD pipelines automates performance testing, catching issues early in development.
Best practices include using realistic loads, gradually increasing traffic, and thorough analysis of test results.
FAQs
Q1: What is k6, and how is it used for load testing?
A1: k6 is an open-source load testing tool that uses JavaScript for scripting and Go for high performance. It is used to simulate traffic, measure performance, and identify bottlenecks in websites and APIs.
Q2: Can k6 be integrated into CI/CD pipelines?
A2: Yes, k6 can be integrated into CI/CD pipelines using tools like Jenkins, GitLab CI, and GitHub Actions, enabling automated performance testing.
Q3: What types of load tests can be performed with k6?
A3: k6 supports various load testing scenarios, including stress testing, spike testing, soak testing, and breakpoint testing.
Q4: How do I analyze the results of a k6 load test?
A4: k6 provides detailed metrics on HTTP performance, checks, and custom metrics. These results help you evaluate if your system meets performance goals.
Q5: What are the best practices for using k6 in load testing?
A5: Best practices include starting with baseline tests, using realistic loads, monitoring system resources, and gradually increasing traffic.
Q6: Is k6 suitable for large-scale load testing?
A6: Yes, k6 can handle large-scale load testing either through local execution or by using k6 Cloud for distributed testing.
Q7: What is the difference between load testing and stress testing in k6?
A7: Load testing evaluates performance under expected conditions, while stress testing pushes the system beyond its limits to find its breaking point.
Q8: Can k6 be used for testing APIs?
A8: Absolutely. k6 is well-suited for API testing, allowing you to simulate multiple users, measure response times, and check for errors.
Comments