In the modern, digital-first world, APIs (Application Programming Interfaces) are the glue that connects various applications, enabling them to communicate with each other. They allow businesses to integrate services, share data, and automate workflows. However, as APIs have become more prevalent, so has the importance of API security. At the center of this security framework lie API credentials—the keys that enable clients to access and interact with an API securely.
Whether you’re developing a new API or integrating an existing one, understanding how API credentials work is essential for ensuring the security of your applications. In this guide, we’ll take an in-depth look at what API credentials are, their types, how they work, and best practices for managing them. We’ll also explore their crucial role in authentication and securing APIs against unauthorized access.
What Are API Credentials?
API credentials are a set of identifiers used to authenticate and authorize clients to access an API. When an application or user tries to communicate with an API, it must provide valid credentials to identify itself. These credentials tell the API server, "I am who I claim to be" and "I have permission to access these resources."
Credentials play a critical role in ensuring that only authorized clients can access the API and its data. Without this authentication mechanism, sensitive information and backend services could be exposed to malicious actors, leading to security breaches.
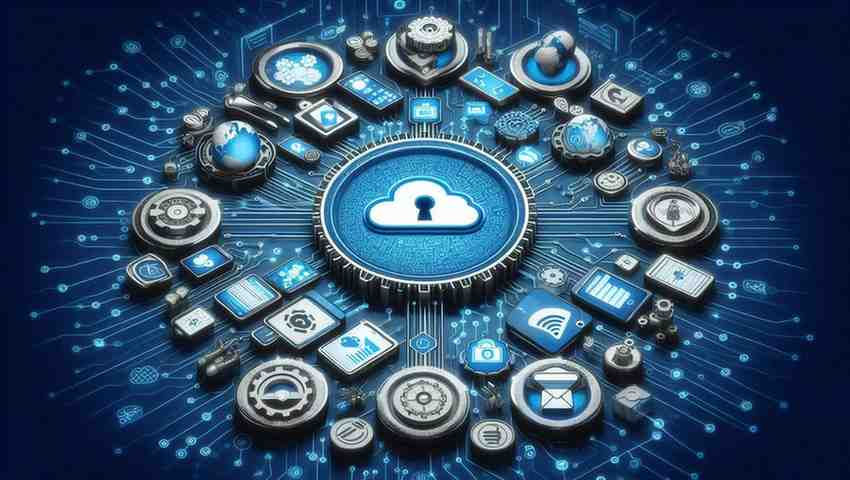
Common Types of API Credentials
Several types of API credentials are commonly used for authentication, including:
API Keys
OAuth Tokens
JWT (JSON Web Tokens)
Client ID and Client Secret
Basic Authentication (Username and Password)
Each of these credential types serves the purpose of identifying and verifying the client, though they differ in their implementation and security capabilities.
How API Credentials Work
API credentials enable clients to identify themselves to an API server, serving as the basis for the authentication workflow. Here’s how the process typically works:
Client Sends Request with Credentials:The client (such as a mobile app or web service) makes a request to the API server, including API credentials in the header, query parameters, or body of the request.
API Server Validates Credentials:The server checks the submitted credentials against its stored records to ensure they are valid. This might involve checking an API key, verifying a token’s signature, or cross-referencing credentials in a user database.
Permissions and Authorization:After validation, the server checks whether the client has the necessary permissions to perform the requested operation. For example, certain credentials may grant read-only access, while others permit full CRUD operations.
Processing the Request:If the credentials are valid and the client is authorized, the API server processes the request and returns the appropriate response (data, confirmation, etc.).
Handling Invalid Credentials:If the API credentials are invalid or the client lacks the necessary permissions, the API server rejects the request with an error, typically returning an HTTP status code like 401 Unauthorized or 403 Forbidden.
Types of API Credentials
1. API Keys
API keys are one of the most straightforward types of credentials used to authenticate clients. An API key is a unique string that identifies the client and provides access to the API. While simple and easy to implement, API keys alone may not offer sufficient security because they are typically sent as plain text, making them vulnerable to interception if not transmitted securely over HTTPS.
Advantages:
Simple to generate and use.
Can be restricted by an IP address or API endpoint.
Disadvantages:
Vulnerable to unauthorized use if intercepted.
Not as secure as token-based authentication systems.
2. OAuth Tokens
OAuth (Open Authorization) is a more advanced and secure authentication framework that issues tokens rather than sharing credentials (like API keys). OAuth allows users to grant access to applications without exposing their username and password. There are two types of tokens in OAuth: Access Tokens and Refresh Tokens.
Access Tokens: Short-lived tokens used to authenticate requests.
Refresh Tokens: Used to generate new access tokens when the original token expires.
OAuth is ideal for applications that require third-party access to a user’s resources, such as logging in with Google or Facebook.
Advantages:
Provides enhanced security with token expiration and refresh.
Allows fine-grained permissions (scope-based access control).
Disadvantages:
More complex to implement.
Requires secure token storage and management.
3. JWT (JSON Web Tokens)
JWT (JSON Web Tokens) are a popular type of token used in API authentication and authorization. JWTs are self-contained tokens that carry encoded information about the client, such as the user’s identity and roles. They consist of three parts: a header, a payload, and a signature. The server can validate the token without needing to store it, which makes JWTs highly scalable.
Advantages:
Stateless (doesn’t require server-side session storage).
Compact and efficient for transmission.
Allows secure transmission of user information between parties.
Disadvantages:
If not properly secured, the payload can be exposed.
Tokens cannot be revoked unless additional mechanisms are used.
4. Client ID and Client Secret
The Client ID and Client Secret pair are often used in OAuth-based authentication workflows. The Client ID is a public identifier for an application, while the Client Secret is a confidential value used to authenticate the application when requesting access tokens.
This method is often used when the client (application) and API server trust each other, such as in server-to-server communications.
Advantages:
Secure for backend or server-to-server API interactions.
Prevents unauthorized access from external parties.
Disadvantages:
Not suitable for front-end applications (where the secret could be exposed).
Requires proper secret storage.
5. Basic Authentication (Username and Password)
Basic Authentication involves sending a username and password in the request header. While this method is simple, it is not recommended for modern APIs because credentials are transmitted in an easily decoded format. Basic Authentication should only be used over HTTPS to ensure the credentials are encrypted during transmission.
Advantages:
Simple to implement.
Supported by most systems out of the box.
Disadvantages:
Not secure if used without encryption.
Does not support fine-grained permissions.
API Credentials and API Security
Ensuring the security of API credentials is essential to protecting sensitive data and preventing unauthorized access. Credentials are often the first target of attackers, and if compromised, they can grant access to critical resources.
Best Practices for Securing API Credentials
Use HTTPS for All API RequestsAlways transmit API credentials over a secure HTTPS connection to prevent interception by malicious actors. APIs using HTTP expose credentials to man-in-the-middle (MitM) attacks.
Rotate API Credentials RegularlyRegularly rotating API keys, tokens, or other credentials can reduce the risk of unauthorized access. If a key is exposed, it will only be valid for a limited time.
Limit Scope and PermissionsGrant the least amount of access necessary when issuing credentials. If a client only needs read access, don’t issue credentials that allow for create or delete operations.
Use IP WhitelistingRestrict the usage of API credentials to certain IP addresses or ranges. This ensures that even if credentials are compromised, they can only be used from trusted locations.
Monitor and Audit API UsageMonitor API usage to detect anomalies, such as excessive requests or attempts from unauthorized IP addresses. Auditing usage can help identify compromised credentials.
Encrypt Sensitive InformationEnsure that sensitive API credentials, such as Client Secrets or JWTs, are securely stored and encrypted. This is particularly important for backend systems where credentials are stored long-term.
How to Implement API Credentials in Modern APIs
When implementing API credentials, you need to decide on the appropriate type of credentials based on your use case. Here’s a high-level overview of how to set up and use various credentials in a typical API setup:
1. Generating API Keys
Most API providers have built-in tools to generate API keys. These keys can then be shared with developers or applications that need to access the API.
In a development environment, you might issue test API keys that only provide limited access.
For production, enforce IP whitelisting and other restrictions on API keys.
2. OAuth Workflow
For applications that require access to a user’s resources, implement OAuth 2.0. The workflow involves:
Redirecting the user to an authorization page.
The user grants access, and the API provider issues an access token.
The client uses this access token to interact with the API on the user’s behalf.
3. Using JWTs
To implement JWT-based authentication:
When a user logs in, generate a JWT with encoded information about the user.
The client includes the JWT in the Authorization header for subsequent requests.
The API server validates the JWT and processes the request.
4. Basic Authentication in Legacy Systems
If you’re working with a legacy system that still uses Basic Authentication, ensure that you transmit credentials over HTTPS and consider upgrading to a more secure method, such as OAuth or JWTs.
Conclusion
API credentials are fundamental to ensuring secure and efficient API interactions. Whether you’re using simple API keys or more complex OAuth tokens, it’s important to understand how these credentials work and implement best practices for securing them. As APIs continue to grow in importance, securing API credentials will remain a critical component of safeguarding your application’s data and functionality.
By understanding the different types of credentials and how to manage them properly, you can create a more secure API ecosystem that mitigates the risks of unauthorized access and data breaches.
Key Takeaways
API credentials are essential for authenticating and authorizing API access.
Common types include API keys, OAuth tokens, JWTs, Client ID/Secret, and Basic Authentication.
Security best practices include using HTTPS, rotating credentials, and monitoring API usage.
Credentials control who can access specific resources and actions in an API.
Choose the appropriate credential type based on security needs and use case.
FAQs
1. What are API credentials?
API credentials are identifiers used by clients to authenticate and authorize themselves to access an API. Common types include API keys, OAuth tokens, and JWTs.
2. How do API credentials work?
API credentials are included in API requests to identify the client. The API server validates the credentials and checks whether the client has permission to perform the requested action.
3. What is the difference between an API key and an OAuth token?
API keys are simple strings used for authentication, while OAuth tokens are more secure, allowing for fine-grained permissions and expiration.
4. Can API credentials be compromised?
Yes, if not properly secured, API credentials can be intercepted or exposed, leading to unauthorized access. Using HTTPS, rotating credentials, and restricting their scope can help mitigate this risk.
5. What is the best way to store API credentials?
API credentials should be stored securely using encryption, especially when stored in backend systems. They should not be exposed to client-side code or version control.
6. Why are JWTs popular for API authentication?
JWTs are stateless and can carry encoded user information, making them efficient for secure API authentication without needing server-side session storage.
Comments