Introduction
The Shopify API Python library allows developers to create custom applications that connect directly to Shopify stores, automating tasks, processing data, and enhancing the online shopping experience for both businesses and customers. Whether you’re building public or private Shopify apps, this library provides a powerful toolkit for creating customized integrations that support Shopify’s robust e-commerce infrastructure.
In this guide, we'll dive into the essentials of the Shopify API Python library, covering everything from setup and installation to advanced usage scenarios like GraphQL queries, billing, pagination, and more.
What is Shopify API Python?
The Shopify API Python library provides a Python-based wrapper for interacting with the Shopify Admin API. This API grants developers access to manage store data, handle orders, update inventory, create custom customer journeys, and more. By using the Shopify API Python library, developers can simplify the complex processes required to integrate with Shopify, such as authentication, data retrieval, and transaction management.
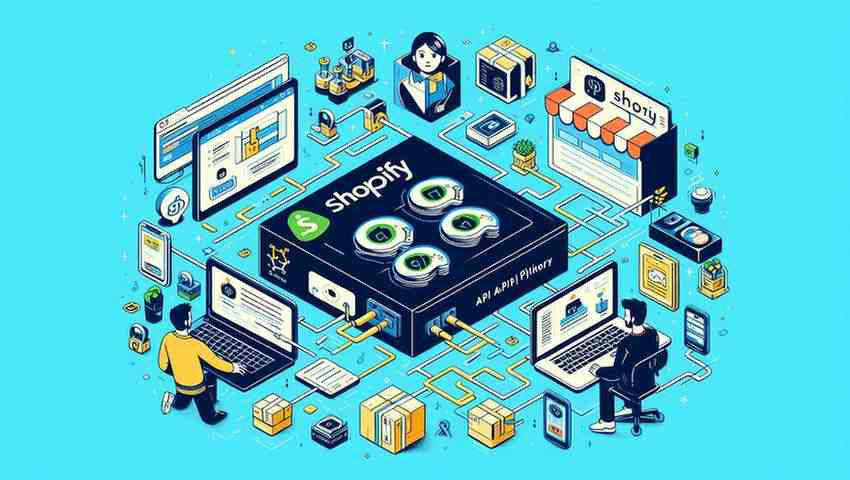
Why Use the Shopify API with Python?
Python is a widely popular programming language, known for its readability, versatility, and extensive library support. By combining Python with Shopify’s API, developers can create scalable, robust applications that interface smoothly with Shopify stores. Some benefits include:
Automation: Automate repetitive store tasks, saving time and resources.
Customization: Create unique, tailored experiences on the Shopify platform.
Data-Driven Decisions: Pull detailed reports to make informed, data-driven decisions.
Seamless API Integration: Python’s compatibility with Shopify's REST and GraphQL APIs simplifies integration.
Setting Up Shopify API Python
To get started with Shopify API Python, make sure you’re signed up as a partner on the Shopify Partners Dashboard. This allows you to create, manage, and monitor your Shopify apps, whether they’re public, custom, or private applications.
1. Installing the Shopify API Python Library
First, ensure you have Python installed (version 3.6+ recommended). Use the following command to install or update the library:
bash
pip install --upgrade ShopifyAPI
2. Requirements
To access the Shopify API, you'll need:
Shopify Partner Account: Register on the Shopify Partners Dashboard.
API Keys: API credentials are necessary to authorize your application.
3. Getting Started with the Shopify API Python Library
The library supports a wide range of functionalities, which we’ll discuss in detail. But to begin, here’s a quick outline of how to set up the basic structure for your Shopify app.
python
import shopify
# Initialize session with your API key, password, and store URL
shop_url = "https://your-store-name.myshopify.com"
api_version = "2021-01"
api_key = "your_api_key"
password = "your_api_password"
session = shopify.Session(shop_url, api_version, api_key, password)
shopify.ShopifyResource.activate_session(session)
# Example of retrieving shop information
shop = shopify.Shop.current()
print(shop)
Types of Shopify Apps
When working with the Shopify API, you can build Public Apps, Custom Apps, and Private Apps, each of which has its specific use cases:
1. Public and Custom Apps
Public Apps: These are available on the Shopify App Store and can be installed by multiple Shopify merchants.
Custom Apps: These apps are designed for specific merchants and are created for custom store requirements.
2. Private Apps
Private apps are built for a single store and can be useful for businesses needing unique integrations that are not shared with other Shopify users.
Establishing Shopify API Sessions
To ensure secure interactions, the Shopify API Python library offers two types of sessions:
1. Full Session
A full session requires an API key and password, offering a persistent connection with full permissions. This is useful for tasks that require continuous background operations.
python
session = shopify.Session("your_store.myshopify.com", api_version, api_key, password)
shopify.ShopifyResource.activate_session(session)
2. Temporary Session
Temporary sessions are useful for short-lived tasks and can be initialized without API keys.
python
with shopify.Session.temp("your_store.myshopify.com", api_version, api_key, password):
shop = shopify.Shop.current()
print(shop)
Working with Billing in Shopify API Python
Shopify requires billing for many of its services. The Python library enables developers to handle billing requirements smoothly:
One-Time Charges: Useful for apps that require a one-time setup fee.
Recurring Charges: For apps that charge users periodically.
Usage-Based Charges: Charges are applied based on usage metrics, like API requests or data storage.
Example code to create a recurring charge:
python
recurring_charge = shopify.RecurringApplicationCharge({
"name": "Recurring Plan",
"price": 10.0,
"return_url": "https://yourapp.com/confirm_billing",
"test": True,
})
recurring_charge.save()
print(recurring_charge.confirmation_url)
Advanced Usage of Shopify API Python
Beyond basic setup and data retrieval, the Shopify API Python library provides more advanced functionalities, including:
1. Using GraphQL
GraphQL is Shopify’s preferred API for more efficient and flexible data queries. It reduces the need for multiple REST calls and enhances the app’s performance.
python
query = """
{
products(first: 5) {
edges {
node {
id
title
}
}
}
}
"""
response = shopify.GraphQL().execute(query)
print(response)
2. Prefix Options
The library includes prefix options that help in organizing API calls based on your application’s unique needs. For example, you can retrieve resources under a specific category.
3. Console Access
You can access Shopify’s resources directly from the console to test small API calls or retrieve data instantly.
Pagination with Relative Cursor
For large datasets, relative cursor pagination enables faster data retrieval by limiting API response sizes. Cursor pagination is essential for efficiently managing extensive data sets like order histories or inventory records.
python
orders = shopify.Order.find(limit=50, page_info="cursor_value")
for order in orders:
print(order)
Using Development Version of Shopify API Python
You may want to try out the latest updates before the official release. Follow these steps to clone, build, and install the development version from the GitHub repository:
Clone the Repository:
bash
git clone https://github.com/Shopify/shopify_python_api.git
cd shopify_python_api
Install the Development Version:
bash
pip install -e .
Run Tests:
bash
python setup.py test
Relative Cursor Pagination for Large Datasets
Relative cursor pagination simplifies retrieving large data sets, allowing you to page through collections. This method is particularly useful when dealing with extensive lists of orders, products, or customer data.
python
orders = shopify.Order.find(limit=50, page_info="cursor_value")
for order in orders:
print(order)
Pre-commit Configuration
Using pre-commit hooks allows you to set coding standards and ensure consistency across your codebase. Run the following command to set up pre-commit locally (optional):
bash
pre-commit install
Limitations of Shopify API Python
While the Shopify API Python library is robust, it has some limitations:
Rate Limiting: Shopify imposes rate limits on API requests, requiring developers to plan for throttling in heavy-usage applications.
Private App Restrictions: Private apps have limited API permissions compared to public and custom apps.
Additional Resources and Sample Apps
Shopify offers sample apps built using this library on the Shopify GitHub page. These resources can be a starting point to understand how the library functions in real-world applications.
Conclusion
The Shopify API Python library enables you to create powerful, scalable Shopify applications that enhance e-commerce functionality. Whether developing custom automation or public apps, this library is indispensable for Python developers aiming to streamline Shopify interactions. By understanding the library’s setup, session management, billing, and advanced usage features, you can fully leverage Shopify's capabilities to support various e-commerce workflows.
Key Takeaways
Versatile Python Library: Enables powerful customizations and integrations for Shopify stores.
Public, Private, and Custom App Support: Flexibility in creating different types of Shopify applications.
Advanced API Functionality: Supports GraphQL, relative pagination, and billing options.
Development-Friendly: Extensive community support and sample apps simplify development.
Pre-commit Support: Ensures code quality through pre-commit hook integration.
Frequently Asked Questions (FAQs)
1. What is the Shopify API Python library?
The Shopify API Python library is a Python wrapper for the Shopify Admin API, enabling developers to build applications that interact with Shopify stores.
2. How can I install the Shopify API Python library?
Install the library using pip: pip install --upgrade ShopifyAPI.
3. Can I use this library for private Shopify apps?
Yes, this library supports private, public, and custom Shopify apps.
4. What is GraphQL, and does Shopify API Python support it?
GraphQL is a data query language, and yes, the Shopify API Python library supports it, offering more efficient data handling.
5. How do I handle rate limits in Shopify API Python?
The library automatically manages Shopify's rate limits, but for high-usage applications, consider implementing additional logic for throttling requests.
6. What is cursor pagination, and why use it?
Cursor pagination allows efficient navigation of large data sets, essential for handling large collections of Shopify orders or products.
7. Does the library support recurring billing?
Yes, the Shopify API Python library supports creating recurring charges for apps.
8. Can I use pre-commit hooks with this library?
Yes, pre-commit hooks can be configured to maintain coding standards across your project.
Comments