Introduction
In the world of modern web development, download test is an essential aspect of automated testing. Whether your web application allows users to download reports, images, documents, or any other type of file, it's important to ensure that the download functionality works smoothly and that the files are correctly saved and accessible.
Cypress, a popular end-to-end testing framework, offers robust tools to handle such tests. This framework allows developers to automate tests for file downloads, making it easy to validate that files are downloaded correctly and have the expected content or structure. With Cypress, you can simulate real-world user behavior, ensuring that the file download functionality is fully tested and reliable across different scenarios.
In this comprehensive guide, we'll walk you through everything you need to know about conducting download tests in Cypress, including how to set up your testing environment, how to automate file downloads, and how to verify the integrity of downloaded files. We’ll cover the installation of necessary tools, running tests on different file types, and addressing common challenges.
By the end of this guide, you will be able to confidently implement automated download tests within your Cypress framework to ensure the seamless performance of your application’s file download features.
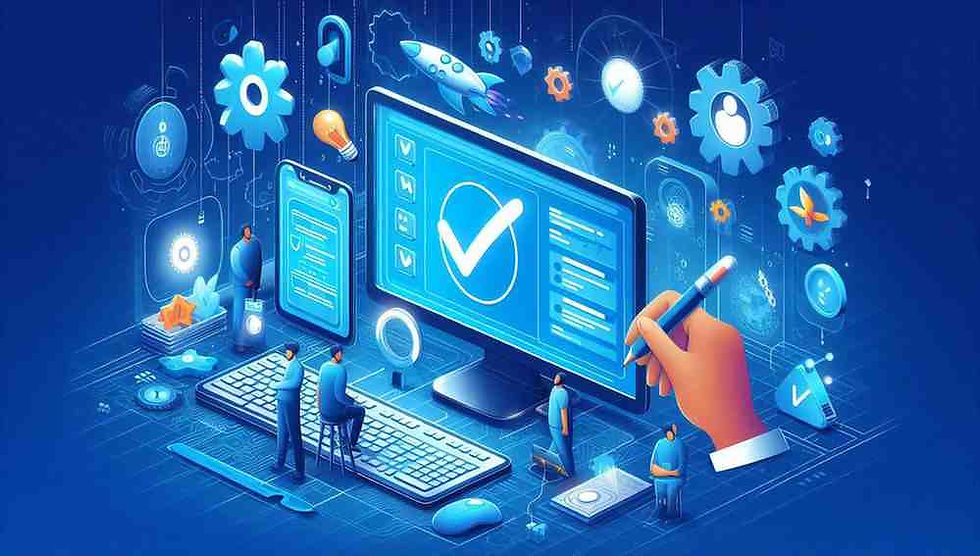
Why is Downloads Testing Important?
Downloads testing is crucial for several reasons:
1. Ensuring User Satisfaction
When users interact with your application, they expect file downloads to be seamless and error-free. Faulty downloads can frustrate users and diminish their experience with your product. Download testing ensures that files are correctly downloaded and accessible, maintaining a high-quality user experience.
2. Maintaining Data Integrity
It's essential to verify that the files users download contain the correct and complete data. By automating download tests, you can ensure that data is preserved accurately, avoiding situations where corrupted or incomplete files are delivered.
3. Preventing Broken Features
File downloads are often core features of many web applications, especially in cases like document generation (e.g., invoices, reports) or media files (e.g., images, videos). Automated testing allows you to quickly identify broken download links, incorrect file formats, or missing files before they affect end users.
4. Security Compliance
In industries dealing with sensitive data, such as healthcare, finance, or legal services, it is important to verify that downloaded files adhere to security and privacy standards. Automating these tests ensures that sensitive information is securely and correctly transferred to the end user.
Setting Up Cypress for Downloads Testing
To begin automating the downloads test in Cypress, you need to set up your testing environment with the appropriate tools and plugins. One of the most commonly used tools for download testing in Cypress is the cypress-downloadfile NPM package.
1. Install Cypress and cypress-download file
To begin, you will need to install Cypress and the cypress-downloadfile plugin. If you haven't already installed Cypress in your project, you can do so by running the following command in your terminal:
bash
npm install cypress --save-dev
After installing Cypress, install the cypress-download file package using the following command:
bash
npm i --save-dev cypress-downloadfile
This package allows you to download files during your Cypress tests and save them to specific directories.
2. Configure BrowserStack and Cypress for Downloads Testing
If you’re running Cypress tests on BrowserStack, the configuration for download testing becomes slightly different. You need to update your browserstack.json file to include the necessary dependencies for the cypress-downloadfile plugin.
Here’s how you can set it up:
json
"run_settings": {
...
"npm_dependencies": {
"cypress-downloadfile": "^1.2.1"
},
...
}
This setup ensures that the plugin is installed and available when running your Cypress tests on BrowserStack.
3. Add Plugin and Command Support
To use the cypress-downloadfile plugin, you need to configure your Cypress project by updating two files: commands.js and index.js.
Update commands.js:
In the cypress/support/commands.js file, add the following line:
javascript
require('cypress-downloadfile/lib/downloadFileCommand');
This will ensure that the download file command is available in your Cypress test scripts.
Update index.js:
Next, in the cypress/plugins/index.js file, add the following code:
javascript
const {downloadFile} = require('cypress-downloadfile/lib/addPlugin');
module.exports = (on, config) => {
on('task', {downloadFile});
}
This will set up the download plugin to work with Cypress.
Writing Cypress Tests for File Downloads
Now that your environment is set up, it’s time to write tests that simulate file downloads and verify their success. Let's go through the process of creating Cypress tests for downloading and validating different types of files.
1. Downloading Files in Cypress
To download a file from a URL, use the cy.downloadFile command in your test script. Here’s an example:
javascript
context('Downloads Test', () => {
it('downloads a file and saves it in the specified directory', () => {
cy.downloadFile('https://sample-videos.com/doc/Sample-doc-file-100kb.doc', 'Downloads', 'sample-doc-file.doc')
});
});
In this example, Cypress downloads a file from a URL and saves it to the Downloads directory with the specified name sample-doc-file.doc.
You can also specify custom directories for your downloads, giving you flexibility in organizing your downloaded files.
2. Verifying Downloaded Files
After downloading the file, it’s important to verify that the file was successfully downloaded and contains the expected content. Cypress provides two main methods to achieve this:
cy.readFile: Use this command to verify the contents of text-based files like .doc, .csv, .txt, etc.
cy.verifyDownload: Use this command to verify the existence of files like .zip, .png, .jpg, and more.
Example Using cy.readFile:
javascript
describe('File Download and Verification Test', () => {
it('should download a document and verify its contents', () => {
cy.downloadFile('https://sample-videos.com/doc/Sample-doc-file
100kb.doc', 'Downloads', 'sample-doc-file.doc');
cy.readFile("./Downloads/sample-doc-file.doc").should('contain', 'Sample Document');
});
});
In this test, we download a .doc file and then use cy.readFile to read the file and verify that it contains the text "Sample Document."
3. Verifying Non-Text Files
For files that are not easily readable, such as images or zip files, you can use cy.verifyDownload to check that the file exists in the specified directory after the download.
javascript
context('Image Download Verification', () => {
it('should download an image and verify its existence', () => {
cy.downloadFile('https://sample-videos.com/img/Sample-png-image1mb.png', 'Downloads', 'sample-image.png');
cy.verifyDownload('sample-image.png');
});
});
Here, we download an image file and verify that it has been successfully saved to the Downloads directory.
Handling Common File Download Issues in Cypress
While conducting download tests, you may run into a few common challenges, such as file corruption, slow network responses, or even incorrect file types being downloaded. Here are some best practices to help you address these issues:
1. Timeout Issues
Downloading large files or files from slow servers may result in timeout errors. You can adjust the default timeout settings in Cypress by using the timeout option in your test configuration.
javascript
it('downloads a large file with extended timeout', () => {
cy.downloadFile('https://example.com/large-file.zip', 'Downloads', 'large-file.zip', { timeout: 120000 });
});
2. File Integrity Validation
To ensure that the file was downloaded without corruption, you can compare the hash of the downloaded file against an expected hash value. This ensures that the file has not been tampered with or corrupted during download.
3. Multiple File Downloads
If your app allows downloading multiple files, you can extend your Cypress test to download and verify multiple files in a single test run.
javascript
context('Multiple Files Download Test', () => {
it('should download multiple files and verify their contents', () => {
cy.downloadFile('https://example.com/sample1.doc', 'Downloads', 'file1.doc');
cy.downloadFile('https://example.com/sample2.pdf', 'Downloads', 'file2.pdf');
cy.readFile("./Downloads/file1.doc").should('contain', 'Document 1 Content');
cy.readFile("./Downloads/file2.pdf").should('contain', 'Document 2 Content');
});
});
Conclusion
Download testing in Cypress is a powerful way to automate the validation of your web application’s file download functionality. By integrating tools like cypress-downloadfile into your test environment, you can easily automate the process of downloading files, verifying their content, and ensuring that everything works as expected across different file types.
In this guide, we've covered the steps to set up your testing environment, write scripts for downloading files, and verify the integrity of those files. Whether you're testing .doc, .pdf, .csv, images, or even compressed files, Cypress offers a flexible solution for all your download testing needs.
By incorporating automated download tests into your workflow, you can ensure that your application’s file download features are reliable and ready for production, helping you deliver a better experience to your users.
Key Takeaways
Download testing ensures that the file download features in your web applications are functioning correctly.
Use cy. download file in Cypress to download files automatically during tests.
cy.readFile allows you to verify the contents of text-based files like .doc or .pdf, while cy.verifyDownload helps check the existence of non-text files.
Cypress can be easily integrated with BrowserStack for cross-browser testing of file downloads.
Best practices, such as adjusting timeouts and validating file integrity, help overcome common issues in download testing.
FAQs on Downloads Test in Cypress
1. What is download testing in Cypress?
Download testing in Cypress involves automating the process of downloading files from web applications and verifying their integrity to ensure that they are saved correctly and contain the expected data.
2. How do I verify file downloads in Cypress?
You can use the cy.readFile command to verify the contents of text-based files and cy.verifyDownload to check that non-text files have been downloaded successfully.
3. Can I test downloads on different file types in Cypress?
Yes, Cypress supports testing downloads for a wide variety of file types, including .doc, .pdf, .png, .jpg, .zip, and more.
4. How do I handle large file downloads in Cypress?
You can extend the default timeout setting in Cypress to accommodate large file downloads by passing a timeout option in your test script.
5. Can I test downloads on BrowserStack with Cypress?
Yes, by configuring your BrowserStack environment and using the cypress-downloadfile plugin, you can test file downloads as part of your Cypress automation on BrowserStack.
6. How do I organize downloaded files during tests?
You can specify custom directories for downloaded files in your Cypress test scripts, allowing you to organize and manage files easily during testing.
7. What are the common issues faced in download testing?
Common issues include timeout errors for large files, file corruption, slow network responses, and incorrect file types being downloaded.
8. How do I ensure the downloaded file is not corrupted?
You can verify the integrity of the downloaded file by comparing its hash value with an expected hash, ensuring the file has not been tampered with or corrupted during the download.
Comentários