Introduction
In the realm of software development, testing is a crucial phase that ensures the quality and functionality of applications. MS Test Manager is an essential tool for managing and executing tests, particularly when integrated with powerful frameworks like Selenium WebDriver and MSTest. This guide provides an in-depth exploration of MS Test Manager, covering everything from setup to advanced usage, ensuring you can maximize its potential for visual testing.
What is MS Test Manager?
Understanding MS Test Manager
MS Test Manager is a testing tool provided by Microsoft that integrates with Visual Studio. It is designed to help developers and testers manage their testing efforts, execute automated tests, and analyze test results efficiently.
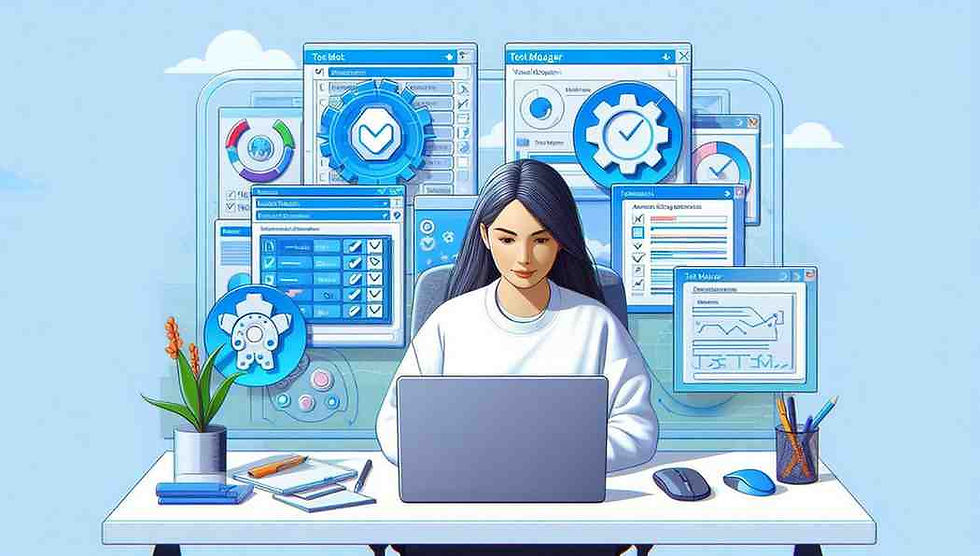
Key Features
Test Management: Organize and manage test cases, plans, and suites.
Automated Testing: Execute automated tests using MSTest, Selenium, and other frameworks.
Result Analysis: Analyze test results to identify issues and ensure quality.
Integration: Seamless integration with Visual Studio and other development tools.
Preparing Your Environment for MS Test Manager
Prerequisites
Before you start using MS Test Manager, ensure you have the following:
Visual Studio: The primary IDE for .NET development, which includes MS Test Manager.
.NET SDK: The software development kit for building and running C# projects.
Google Chrome and ChromeDriver: For running Selenium WebDriver tests.
Applitools Account: For visual testing and leveraging the Ultrafast Grid and Execution Cloud.
Installing ChromeDriver
Ensure ChromeDriver is installed and accessible via the system PATH. You can verify the installation by running chromedriver -v in your terminal.
Getting Started with MS Test Manager
Setting Up a Test Project
To create a new test project in Visual Studio:
Open Visual Studio: Launch the IDE and create a new project.
Select Project Template: Choose "Unit Test Project" for MSTest.
Configure Project: Name your project and configure settings as needed.
Integrating Selenium WebDriver and Applitools
To integrate Selenium WebDriver and Applitools into your project:
Install NuGet Packages: Add the necessary packages for Selenium WebDriver and Applitools Eyes SDK.
Configure Applitools: Set up your Applitools API key and configure visual testing options.
Example Project Setup
You can use the example project provided at Applitools GitHub. Clone the repository and follow the setup instructions.
Writing and Running Tests in MS Test Manager
Creating a Test Case
In Visual Studio, create a new test class and write your test methods. Use attributes like [TestClass] and [TestMethod] to define your test structure.
Sample Test Case
Here is a sample test case for the ACME Bank demo app:
csharp
using Applitools.Selenium;
using Applitools.VisualGrid;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using OpenQA.Selenium;
using OpenQA.Selenium.Chrome;
using OpenQA.Selenium.Remote;
using System.Drawing;
namespace Applitools.Example.Tests
{
[TestClass]
public class AcmeBankTest
{
public const bool UseUltrafastGrid = true;
public const bool UseExecutionCloud = false;
public TestContext TestContext { get; set; }
private static string? ApplitoolsApiKey;
private static bool Headless;
private static BatchInfo Batch;
private static Configuration Config;
private static EyesRunner Runner;
private WebDriver Driver;
private Eyes Eyes;
[AssemblyInitialize]
public static void SetUpConfigAndRunner(TestContext context)
{
ApplitoolsApiKey = Environment.GetEnvironmentVariable("APPLITOOLS_API_KEY");
Headless = Environment.GetEnvironmentVariable("HEADLESS")?.ToLower() == "true";
if (UseUltrafastGrid)
{
Runner = new VisualGridRunner(new RunnerOptions().TestConcurrency(5));
}
else
{
Runner = new ClassicRunner();
}
Batch = new BatchInfo($"Example: Selenium C# MSTest with {(UseUltrafastGrid ? "Ultrafast Grid" : "Classic Runner")}");
Config = new Configuration().SetApiKey(ApplitoolsApiKey).SetBatch(Batch);
if (UseUltrafastGrid)
{
Config.AddBrowser(800, 600, BrowserType.CHROME);
Config.AddBrowser(1600, 1200, BrowserType.FIREFOX);
Config.AddBrowser(1024, 768, BrowserType.SAFARI);
Config.AddDeviceEmulation(DeviceName.Pixel_2, ScreenOrientation.Portrait);
Config.AddDeviceEmulation(DeviceName.Nexus_10, ScreenOrientation.Landscape);
}
}
[TestInitialize]
public void OpenBrowserAndEyes()
{
ChromeOptions options = new ChromeOptions();
if (Headless) options.AddArgument("headless");
if (UseExecutionCloud)
{
Driver = new RemoteWebDriver(new Uri(Eyes.GetExecutionCloudUrl()), options);
}
else
{
Driver = new ChromeDriver(options);
}
Driver.Manage().Timeouts().ImplicitWait = TimeSpan.FromSeconds(10);
Eyes = new Eyes(Runner).SetConfiguration(Config);
Eyes.SaveNewTests = true;
Eyes.Open(Driver, "ACME Bank Web App", TestContext.TestName, new Size(1200, 600));
}
[TestMethod]
public void LogIntoBankAccount()
{
Driver.Navigate().GoToUrl("https://demo.applitools.com");
Eyes.Check(Target.Window().Fully().WithName("Login page"));
Driver.FindElement(By.Id("username")).SendKeys("applibot");
Driver.FindElement(By.Id("password")).SendKeys("I<3VisualTests");
Driver.FindElement(By.Id("log-in")).Click();
Eyes.Check(Target.Window().Fully().WithName("Main page").Layout());
}
[TestCleanup]
public void CleanUpTest()
{
Eyes.Close();
Driver.Quit();
}
[AssemblyCleanup]
public static void PrintResults()
{
TestResultsSummary allTestResults = Runner.GetAllTestResults();
Console.WriteLine(allTestResults);
}
}
}
Running Tests
To run tests, use the Test Explorer in Visual Studio or the dotnet CLI. Ensure your Applitools API key is set as an environment variable.
Advanced Techniques with MS Test Manager
Using Applitools Ultrafast Grid
The Ultrafast Grid allows you to run visual tests across multiple browsers and devices simultaneously, significantly reducing test execution time.
Handling Dynamic Content
Configure match levels to handle dynamic content effectively. Use layout match levels for pages with varying content but consistent structure.
Continuous Integration
Integrate MS Test Manager with your CI/CD pipeline to automate testing and ensure continuous quality checks.
Best Practices for Using MS Test Manager
Regularly Update Baselines
Keep your baseline images updated to reflect the latest application design, reducing false positives.
Modular Test Design
Organize tests into logical units to enhance readability and maintainability. Use test classes and methods appropriately.
Parameterize Tests
Use parameterization to run tests with different inputs, increasing test coverage and flexibility.
Monitor Test Performance
Regularly review test execution times and optimize tests to improve performance.
Conclusion
MS Test Manager, when combined with Selenium WebDriver and Applitools, provides a powerful framework for comprehensive visual testing. By following best practices and leveraging advanced features, you can ensure high-quality, visually consistent applications.
Key Takeaways
MS Test Manager integrates seamlessly with Visual Studio for efficient test management.
Proper setup and configuration are crucial for successful visual testing.
Applitools enhances visual testing with features like the Ultrafast Grid and Execution Cloud.
Regularly update baselines and use appropriate match levels for dynamic content.
Integrate with CI/CD pipelines for continuous testing and quality assurance.
FAQs
What is MS Test Manager?
MS Test Manager is a test management tool provided by Microsoft that integrates with Visual Studio, allowing developers to organize, execute, and analyze tests efficiently.
How do I set up MS Test Manager for visual testing?
Set up MS Test Manager by integrating it with Selenium WebDriver and Applitools. Configure your project in Visual Studio, install necessary packages, and set environment variables like the Applitools API key.
What are the benefits of using Applitools with MS Test Manager?
Applitools provides advanced visual testing capabilities, including the Ultrafast Grid for cross-browser testing and Execution Cloud for managing WebDriver sessions, enhancing the efficiency and accuracy of visual tests.
How do I handle dynamic content in visual tests?
Use the layout match level in Applitools to focus on the structural consistency of pages with dynamic content, ignoring irrelevant differences in text and graphics.
Can I integrate MS Test Manager with CI/CD pipelines?
Yes, integrating MS Test Manager with CI/CD pipelines automates testing and ensures continuous quality checks, enhancing the development workflow.
What are best practices for using MS Test Manager?
Best practices include regularly updating baselines, modular test design, parameterizing tests, and monitoring test performance for optimization.
How do I run tests using the dotnet CLI?
To run tests using the dotnet CLI, navigate to your project directory and use commands like dotnet build and dotnet test.
What is the Ultrafast Grid in Applitools?
The Ultrafast Grid in Applitools allows you to run visual tests across multiple browsers and devices simultaneously, reducing test execution time and increasing coverage.
Commentaires