Postman Automation: Guide to Effortless API Testing
- Gunashree RS
- 2 days ago
- 8 min read
In today's interconnected digital ecosystem, APIs (Application Programming Interfaces) serve as the critical connectors between different software systems. As applications grow more complex and distributed, ensuring these connectors function flawlessly becomes paramount. Enter Postman automation—a powerful approach to API testing that transforms manual, time-consuming verification processes into streamlined, repeatable test workflows.
Postman has evolved from a simple API client to a comprehensive platform that enables developers and testers to automate complex API workflows with minimal effort. Whether you're a developer looking to validate your APIs during development or a QA engineer responsible for ensuring API reliability, mastering Postman automation can significantly enhance your productivity and the quality of your applications.
Understanding Postman Automation Fundamentals
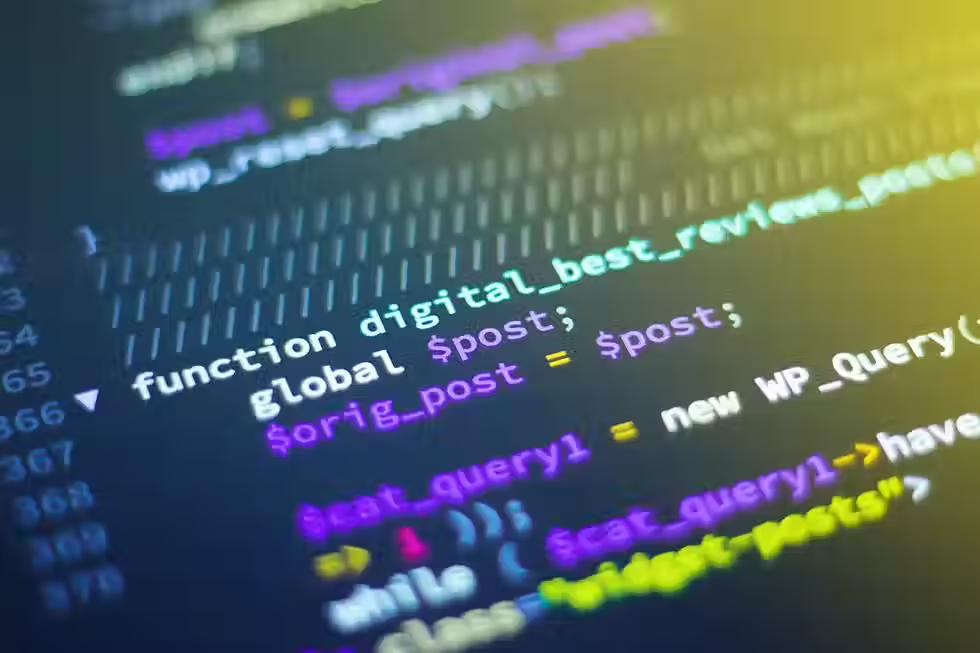
Postman automation centers around creating programmatic tests that validate API functionality, performance, and reliability. At its core, this automation capability allows you to:
Write test scripts using JavaScript to verify expected responses
Create collections of related API requests
Define environments for different testing scenarios
Run collections automatically through various triggers
Generate reports to track API health and performance
The true power of Postman automation lies in its ability to transform one-off manual API calls into comprehensive test suites that can be executed repeatedly across different environments with consistent results.
Key Components of Postman Automation
To effectively implement Postman automation, understanding its primary components is essential:
1. Collections
Collections serve as containers for organizing related API requests. They can be structured to:
Group endpoints by functionality
Represent user flows through an application
Test specific features or requirements
Create end-to-end testing scenarios
Collections form the foundation of automation in Postman, as they define what requests will be executed during test runs.
2. Test Scripts
Test scripts are JavaScript code snippets that execute after an API request completes. These scripts can:
Validate response status codes
Check the response body content
Verify response headers
Confirm response times meet performance requirements
Extract and store data for subsequent requests
javascript
// Example test script to verify the successful response
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Response contains user data", function () {
const responseJson = pm.response.json();
pm.expect(responseJson).to.have.property('name');
pm.expect(responseJson).to.have.property('email');
});
3. Pre-Request Scripts
Pre-request scripts execute before an API request is sent. These scripts are useful for:
Generating dynamic request parameters
Setting environment variables
Creating test data
Implementing authentication logic
javascript
// Example pre-request script to set dynamic timestamp
pm.environment.set('timestamp', Date.now());
4. Environments
Environments allow you to define sets of variables that can be used across requests. They facilitate:
Testing across different deployment stages (development, staging, production)
Storing configuration parameters
Managing authentication credentials
Maintaining test data
Setting Up Your First Automated Test in Postman
Creating your first automated test in Postman involves several straightforward steps that build upon each other to create a comprehensive testing solution.
Step 1: Creating a Collection
Begin by organizing your API endpoints into a logical collection:
Click the "New" button in Postman
Select "Collection"
Name your collection descriptively
Add optional description and documentation
Save the collection
Step 2: Adding Requests to Your Collection
Once your collection is created, populate it with the API requests you want to test:
Click on your collection
Select "Add Request"
Configure the request method (GET, POST, PUT, DELETE, etc.)
Enter the request URL
Add any necessary headers, query parameters, or body content
Save the request with a descriptive name
Step 3: Writing Test Scripts
For each request, add test scripts to validate the expected behavior:
Select the "Tests" tab in your request
Write JavaScript test assertions using Postman's built-in testing framework
Use the pm.test() function to create named test cases
Implement assertions using the ChaiJS assertion library
Common test scenarios include:
Verifying correct status codes
Validating response structure
Checking specific data values
Testing error conditions
Measuring response times
javascript
// Example comprehensive test script
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Response time is acceptable", function() {
pm.expect(pm.response.responseTime).to.be.below(500);
});
pm.test("Content-Type header is present", function () {
pm.response.to.have.header("Content-Type");
});
pm.test("Response body contains required data", function () {
const responseJson = pm.response.json();
pm.expect(responseJson).to.be.an('object');
pm.expect(responseJson.users).to.be.an('array').that.is.not.empty;
pm.expect(responseJson.users[0]).to.have.property('id');
pm.expect(responseJson.users[0]).to.have.property('name');
});
Step 4: Setting Up Data-Driven Tests
To make your tests more robust, implement data-driven testing:
Create a CSV or JSON file with test data
Configure the collection runner to use this data file
Reference data variables in your requests and tests
Run the collection against multiple data sets
Advanced Postman Automation Techniques
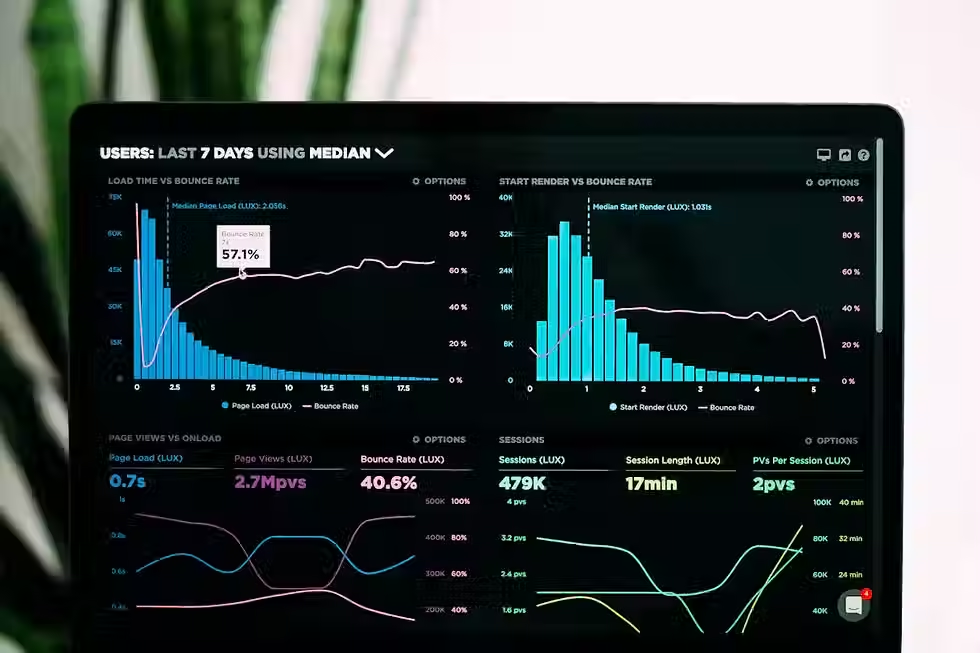
Once you've mastered the basics, explore these advanced automation techniques to enhance your API testing workflow.
Creating Test Workflows with Collection Runners
Collection runners execute multiple requests in sequence, allowing you to test complex user journeys:
Open the Collection Runner from the Postman interface
Select the collection to run
Configure iteration count and delay
Choose environment
Import data files if needed
Start the run and analyze the results
Implementing Request Chaining
Request chaining enables data to flow between requests in a collection:
Use the pm.environment.set() method to store response data
Reference stored values in subsequent requests using variables
Create dependencies between requests to test complex workflows
Example workflow:
Step | Request | Purpose | Data Flow |
1 | POST /login | Authenticate | Store the authentication token |
2 | GET /users | Retrieve users | Use an authentication token |
3 | POST /users | Create a new user | Use an authentication token |
4 | GET /users/{id} | Verify creation | Use ID from the previous response |
5 | PUT /users/{id} | Update user | Use ID from step 3 |
6 | DELETE /users/{id} | Delete user | Use ID from step 3 |
Automating with Newman
Newman is Postman's command-line collection runner that enables CI/CD integration:
Install Newman via npm: npm install -g newman
Export your Postman collection and environment
Run collections from the command line:
newman run collection.json -e environment.json
Generate test reports in various formats:
newman run collection.json -e environment.json -r cli,json,html
CI/CD Integration
Integrate Postman tests into your CI/CD pipeline for continuous API testing:
Export collections and environments
Add Newman to your build process
Configure test runs in your CI/CD platform (Jenkins, GitLab CI, GitHub Actions, etc.)
Set failure conditions based on test results
Archive test reports as build artifacts
Example GitHub Actions workflow:
yaml
name: API Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Newman
run: npm install -g newman
- name: Run API Tests
run: newman run postman/collection.json -e postman/environment.json -r cli,htmlextra
- name: Archive test results
uses: actions/upload-artifact@v2
with:
name: api-test-results
path: newman/
Best Practices for Postman Automation
Implementing these best practices will help you create more maintainable and effective API tests:
1. Organize Collections Logically
Group related requests together
Use folders for different functional areas
Name requests descriptively
Add documentation to collections and requests
2. Implement Robust Error Handling
Test both success and failure scenarios
Validate error responses and messages
Check for appropriate HTTP status codes
Test boundary conditions and edge cases
3. Keep Tests Focused and Independent
Each test should verify one specific aspect
Avoid dependencies between tests when possible
Use clear, descriptive test names
Follow the Arrange-Act-Assert pattern
4. Manage Environments Effectively
Create separate environments for different stages (dev, staging, prod)
Never hardcode sensitive values in requests
Use variables for all configurable parameters
Implement dynamic variable generation where appropriate
5. Version Control Your Postman Assets
Export collections and environments to JSON
Store in version control alongside application code
Include in code review processes
Track changes over time
Monitor API Performance with Postman
Beyond functional testing, Postman enables continuous API monitoring:
Create a monitor from existing collections
Define run frequency (minutes, hours, or days)
Configure alert notifications for failures
View historical performance data
Set up regional monitoring for global APIs
Monitoring provides crucial insights into:
API uptime and availability
Response time trends
Regression issues
Geographic performance variations
Conclusion
Postman automation transforms API testing from a manual, error-prone process into a streamlined, repeatable workflow. By mastering collections, test scripts, environments, and integration capabilities, you can build a comprehensive API testing strategy that catches issues earlier and ensures higher quality releases.
The true power of Postman automation lies in its flexibility—from simple request validation to complex workflow testing and CI/CD integration. As APIs continue to form the backbone of modern software systems, investing time in robust automation pays dividends through improved reliability, faster development cycles, and higher quality applications.
Whether you're just starting with API testing or looking to enhance your existing processes, Postman automation provides the tools and capabilities to meet your needs. Begin with simple tests, gradually implement more advanced techniques, and continuously refine your approach to create an API testing strategy that evolves with your applications.
Key Takeaways
Postman automation enables consistent, repeatable API testing that improves application quality and developer productivity.
Collections organize related requests and form the foundation of automated test suites.
Test scripts written in JavaScript validate API responses and behaviors
Pre-request scripts prepare test data and set up test conditions
Environments facilitate testing across different deployment stages
Newman enables command-line execution and CI/CD integration
Request chaining allows for testing complex API workflows and user journeys
Monitoring provides continuous visibility into API health and performance
Best practices include logical organization, error handling, test independence, and version control
Postman automation can scale from individual developers to enterprise-wide testing strategies
FAQ
How do I run automated tests in Postman?
To run automated tests in Postman, you can use the Collection Runner feature. Click on the Collection Runner button, select your collection, choose the appropriate environment, set the iteration count, and click "Run". Alternatively, you can use Newman, Postman's command-line tool, by installing it via npm and running commands like newman run collection.json -e environment.json.
Can Postman automate API authentication?
Yes, Postman can automate various authentication methods. You can handle authentication by setting up pre-request scripts to generate tokens, using environment variables to store credentials, or leveraging Postman's built-in auth helpers for OAuth, API Key, Bearer Token, and other authentication types. For dynamic token generation, implement the logic in pre-request scripts and store the token in environment variables.
How do I schedule automated tests in Postman?
Postman offers two approaches for scheduling tests. First, with a Postman account, you can create Monitors that run collections at specified intervals (hourly, daily, weekly) and send notifications upon failure. Second, for self-hosted solutions, use Newman with task schedulers like cron (Linux/Mac) or Task Scheduler (Windows), or integrate with CI/CD pipelines for event-based scheduling.
What's the difference between pre-request scripts and test scripts?
Pre-request scripts run before a request is sent, making them ideal for request preparation tasks like generating timestamps, creating test data, or handling authentication. Test scripts run after receiving the response and are used to validate the response through assertions on status codes, response body, headers, and timing. Both use JavaScript and can access Postman's pm.* API for managing variables and request/response data.
How can I extract data from one request to use in another?
To pass data between requests in a collection, use environment or collection variables. In the test script of the first request, extract the needed data and save it using pm.environment.set('variableName', value) or pm.collectionVariables.set('variableName', value). In subsequent requests, reference this variable using the syntax {{variableName}} in URLs, headers, or request bodies.
Can Postman automate file uploads and downloads?
Yes, Postman supports automating file operations. For uploads, use the "form-data" Body option and select file inputs. You can dynamically generate file paths in pre-request scripts. For downloads, you can verify file response headers and content, though storing downloaded files requires Newman or additional tooling. Binary response handling is supported through the pm.response.arrayBuffer() method in test scripts.
How do I integrate Postman tests with CI/CD pipelines?
Integrate Postman with CI/CD by using Newman, Postman's command-line runner. Export your collection and environment as JSON files, add them to your repository, install Newman in your CI environment, and configure your pipeline to run Newman commands. Most CI platforms (Jenkins, GitHub Actions, GitLab CI, CircleCI) can execute Newman tests and process the generated reports to fail builds when tests don't pass.
What are the limitations of Postman automation?
Postman automation has some limitations: complex UI interactions aren't supported (use Selenium or Cypress instead), performance testing is basic compared to specialized tools like JMeter, the JavaScript environment doesn't support all Node.js features, test organization becomes challenging for very large API suites, and advanced data generation might require external scripts. Free accounts also have limitations on collection runs and monitoring.
Commenti