API testing is a cornerstone of modern software development, ensuring systems can communicate effectively and deliver seamless user experiences. However, with frequent updates and feature enhancements, manual testing can quickly become overwhelming and prone to errors. Automating API tests with Postman is the perfect solution to streamline processes and ensure reliability.
This article provides a detailed guide on the steps to automate API tests in Postman, making your testing efforts faster, more efficient, and scalable.
What Is API Testing and Why Automate It?
API testing involves verifying that an application programming interface (API) works as expected and handling requests and responses correctly. Automation takes this process to the next level by reducing manual intervention and ensuring consistency in test execution.
Benefits of Automating API Tests
Time Savings: Automating repetitive tasks frees up valuable developer time.
Increased Accuracy: Reduces human errors that occur during manual testing.
Early Bug Detection: Integrating automated tests into CI/CD pipelines ensures issues are identified early in development.
Scalability: Handle complex test scenarios with ease as projects grow.
Postman is a popular choice for API test automation, thanks to its user-friendly interface, powerful features, and integration capabilities.
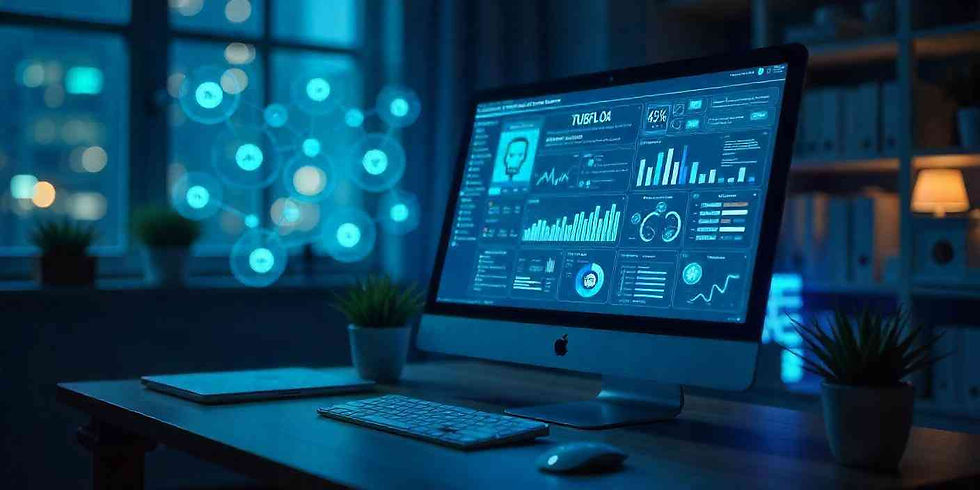
Steps to Automate API Tests in Postman
Automating API tests in Postman involves creating test cases, running them, and integrating them into your workflows. Let’s break it down into actionable steps:
Step 1: Install and Set Up Postman
To begin, download and install Postman on your system:
Visit the Postman website.
Download the appropriate version for your operating system.
Install and launch Postman.
Once installed, create a free Postman account to save your work and access your tests across devices.
Step 2: Create a New Collection
Postman organizes API requests into collections, which are essential for managing automated tests.
Open Postman and click New Collection.
Name the collection (e.g., "User Management API Tests").
Add a description to provide context.
Collections serve as containers for your API requests and test scripts.
Step 3: Add API Requests to the Collection
Click Add Request under your collection.
Enter the API endpoint (e.g., https://api.example.com/users).
Choose the appropriate HTTP method (GET, POST, PUT, DELETE, etc.).
Set headers, parameters, or body data as needed.
Test your API request by clicking Send to confirm that it works as expected.
Step 4: Write Test Scripts
Postman allows you to write JavaScript-based test scripts in the Tests tab of each request. These scripts automate the validation of API responses.
Basic Test Script Example:
javascript
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Response time is less than 300ms", function () {
pm.expect(pm.response.responseTime).to.be.below(300);
});
Assertions: Validate the response code, headers, and body content.
Dynamic Data: Extract data from responses for use in subsequent requests.
Step 5: Use Environment Variables
Environment variables make your tests reusable and adaptable to different environments (e.g., staging, production).
Steps to Set Up Variables:
Go to the Environment tab in Postman.
Define variables such as {{base_url}} or {{auth_token}}.
Replace hardcoded values in requests with these variables.
Example: Instead of https://api.example.com, use {{base_url}}/users.
Step 6: Configure Data-Driven Testing
Data-driven testing allows you to test multiple scenarios using a single request.
Steps to Implement:
Prepare a data file (CSV or JSON) with test inputs.
In the Collection Runner, upload the data file.
Use variables in your requests to dynamically substitute values from the file.
Example:
JSON
[
{ "username": "testuser1", "password": "pass123" },
{ "username": "testuser2", "password": "pass456" }
]
Step 7: Use the Collection Runner
Postman’s Collection Runner executes all requests in a collection sequentially.
Select your collection in the Collection Runner.
Choose the environment and data file, if applicable.
Set the number of iterations.
Click Run to execute your tests.
The Collection Runner provides real-time results and detailed logs for each request.
Step 8: Automate with Newman
Newman, Postman’s command-line tool, enables automation and integration into CI/CD pipelines.
Steps to Use Newman:
Export your collection and environment files from Postman. Install Newman via npm:
npm install -g newman
Run your collection using Newman: bash
newman run your_collection.json -e your_environment.json
Newman supports advanced features like HTML reporting, making it ideal for collaborative workflows.
Step 9: Integrate with CI/CD Pipelines
Integrate Postman tests into CI/CD tools like Jenkins, GitHub Actions, or Azure DevOps to ensure automated testing at every stage of development.
Sample Integration with Jenkins:
Add a new Jenkins job for your project.
Include a shell script to run Newman commands.
Trigger the job on code commits or pull requests.
This ensures regression testing is automated and bugs are caught early.
Step 10: Analyze Results and Refine Tests
Postman provides detailed reports on test results, including success rates, response times, and failure logs. Use this feedback to:
Identify and fix failing test cases.
Optimize API endpoints for better performance.
Expand test coverage to include additional scenarios.
Best Practices for Automating API Tests in Postman
Organize Requests Effectively Group related requests into folders within your collection for better readability.
Maintain Modular Test Scripts Write reusable functions and scripts to simplify updates and enhance maintainability.
Leverage Postman Monitors Use Postman’s monitoring feature to schedule automated tests at regular intervals.
Document Your Tests Provide detailed descriptions and comments for each test to aid collaboration and debugging.
Regularly Update Test Suites As APIs evolve, ensure your test cases reflect the latest changes and requirements.
FAQs
1. What is the purpose of automating API tests?
Automation reduces manual effort, increases test accuracy, and ensures faster feedback during development.
2. Can I perform data-driven testing in Postman?
Yes, Postman supports data-driven testing through the Collection Runner and external data files.
3. What is Newman, and why use it?
Newman is a command-line tool for running Postman collections, enabling integration with CI/CD pipelines.
4. Is coding experience necessary for Postman automation?
Basic knowledge of JavaScript is helpful for writing test scripts, but Postman’s interface is beginner-friendly.
5. Can Postman handle large test suites?
Yes, with proper organization, modular scripts, and environment variables, Postman can manage complex test suites.
6. How does Postman compare to other automation tools?
Postman is user-friendly and versatile, though tools like JMeter may be better for performance-heavy tests.
7. Is it possible to schedule automated tests in Postman?
Yes, Postman Monitors allows you to schedule tests and receive notifications for results.
8. How do I debug failing tests in Postman?
Use Postman’s detailed response logs, console output, and reporting features to identify and fix issues.
Conclusion
Automating API tests in Postman is a game-changer for modern software development. By following the outlined steps—creating collections, writing test scripts, leveraging environment variables, and integrating with CI/CD pipelines—you can streamline testing, enhance efficiency, and deliver high-quality applications. Postman’s robust features, combined with best practices, make it an indispensable tool for API automation.
Key Takeaways
Postman simplifies API test automation with its user-friendly interface and powerful features.
Automation reduces manual effort, increases accuracy, and scales effectively.
Newman and CI/CD integration enable seamless automated workflows.
Regularly updating and optimizing test suites ensures comprehensive coverage.
Comments