Introduction
WebdriverIO is a powerful and versatile tool for testing web applications using JavaScript. Its flexibility and comprehensive features make it a favorite among developers and testers alike. This guide will walk you through everything you need to know about WebdriverIO, from setting up your environment to leveraging advanced visual testing techniques with Applitools. Whether you are a beginner or an experienced tester, this article will provide valuable insights to enhance your testing capabilities.
Understanding WebdriverIO
What is WebdriverIO?
WebdriverIO is a JavaScript-based framework for testing web applications. It allows developers to write and run tests using WebDriver, which is a W3C standard for automating web browsers. WebdriverIO provides a robust and flexible platform for creating automated tests, making it an essential tool for modern web development.
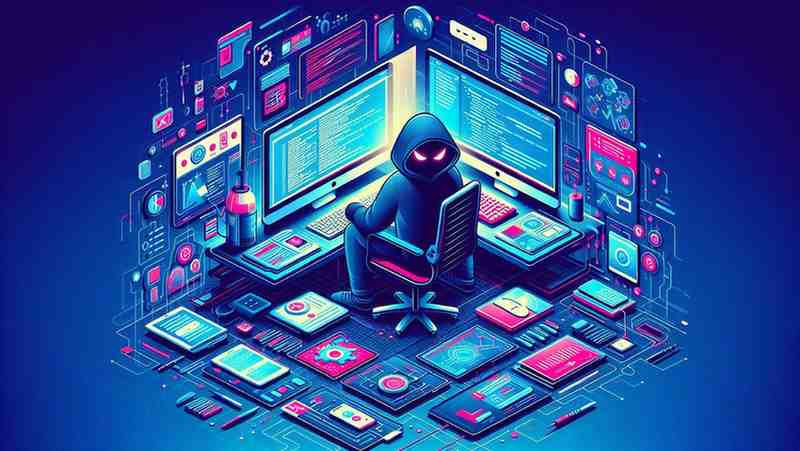
Key Features of WebdriverIO
Support for Multiple Browsers: WebdriverIO supports testing on all major browsers, including Chrome, Firefox, Safari, and Edge.
Cross-Platform Testing: It allows testing on various operating systems, ensuring compatibility and reliability.
Integration with Testing Frameworks: WebdriverIO seamlessly integrates with popular testing frameworks like Mocha, Jasmine, and Cucumber.
Advanced Debugging: It provides powerful debugging tools to help identify and fix issues quickly.
Rich API: WebdriverIO offers a comprehensive API for interacting with web elements, making test development intuitive and efficient.
Preparing Your Environment
Setting Up Your Environment
Before you start testing with WebdriverIO, you'll need to prepare your environment. Follow these steps to get started:
Create an Applitools Account: Register for a free Applitools account to leverage its visual testing capabilities.
Install Node.js: Ensure you have a recent version of Node.js installed on your machine.
Choose a Code Editor: Use a good JavaScript editor like Visual Studio Code for writing your tests.
Install Google Chrome: Ensure you have the latest version of Google Chrome installed.
Installing WebdriverIO
To install WebdriverIO and its dependencies, follow these commands:
bash
npm init -y
npm install webdriverio @wdio/cli --save-dev
npx wdio config
The wdio config command will guide you through setting up your configuration file. Select options that suit your testing needs, such as the testing framework (Mocha is a good default choice).
Cloning the Example Project
To help you get started quickly, clone the example project provided by Applitools:
bash
git clone https://github.com/applitools/example-webdriverio-javascript.git
cd example-webdriverio-javascript
If you don't have Git installed, you can download the project as a ZIP file and extract it.
Installing Dependencies
Install the project dependencies and ChromeDriver:
bash
npm install
npm install chromedriver --detect_chromedriver_version
If you want to add Applitools to another WebdriverIO project, run:
bash
npm install --save-dev @applitools/eyes-webdriverio
Getting Started with WebdriverIO
Basic WebdriverIO Test Example
Create a simple test to ensure everything is set up correctly. Create a file named test.js with the following content:
javascript
const { remote } = require('webdriverio');
(async () => {
const browser = await remote({
capabilities: { browserName: 'chrome' }
});
await browser.url('https://webdriver.io');
const title = await browser.getTitle();
console.log('Title is: ' + title);
await browser.deleteSession();
})();
Run the test using the following command:
bash
node test.js
You should see the title of the WebdriverIO homepage printed in the console.
Using Applitools for Visual Testing
Visual testing can catch problems that traditional automation might miss. To use Applitools with WebdriverIO, follow these steps:
1.Set Your API Key: Set your Applitools API key as an environment variable:bash
export APPLITOOLS_API_KEY=<your-api-key>
2.Add Visual Testing Code: Modify your test to include visual testing with Applitools:javascript
const { remote } = require('webdriverio');
const { Eyes, Target, ClassicRunner, Configuration, BatchInfo } = require('@applitools/eyes-webdriverio');
(async () => {
const browser = await remote({
capabilities: { browserName: 'chrome' }
});
const eyes = new Eyes(new ClassicRunner());
const config = new Configuration();
config.setApiKey(process.env.APPLITOOLS_API_KEY);
config.setBatch(new BatchInfo('WebdriverIO Test Batch'));
eyes.setConfiguration(config);
await eyes.open(browser, 'WebdriverIO Test App', 'My Test', { width: 1200, height: 800 });
await browser.url('https://webdriver.io');
await eyes.check('Main Page', Target.window().fully());
await eyes.close();
await browser.deleteSession();
})();
3.Run the Test: Execute the test with:bashnode test.js
Check your Applitools dashboard to see the visual test results.
Advanced Features of WebdriverIO
Cross-Browser Testing with Applitools Ultrafast Grid
The Applitools Ultrafast Grid allows you to run visual tests across multiple browsers and devices quickly. To use it, update your configuration:
javascript
const { remote } = require('webdriverio');
const { Eyes, VisualGridRunner, RunnerOptions, Target, Configuration, BatchInfo, BrowserType, DeviceName, ScreenOrientation } = require('@applitools/eyes-webdriverio');
(async () => {
const browser = await remote({
capabilities: { browserName: 'chrome' }
});
const runner = new VisualGridRunner(new RunnerOptions().testConcurrency(5));
const eyes = new Eyes(runner);
const config = new Configuration();
config.setApiKey(process.env.APPLITOOLS_API_KEY);
config.setBatch(new BatchInfo('WebdriverIO Ultrafast Grid Test'));
config.addBrowser(800, 600, BrowserType.CHROME);
config.addBrowser(1600, 1200, BrowserType.FIREFOX);
config.addBrowser(1024, 768, BrowserType.SAFARI);
config.addDeviceEmulation(DeviceName.Pixel_2, ScreenOrientation.PORTRAIT);
config.addDeviceEmulation(DeviceName.Nexus_10, ScreenOrientation.LANDSCAPE);
eyes.setConfiguration(config);
await eyes.open(browser, 'WebdriverIO Test App', 'My Test', { width: 1200, height: 800 });
await browser.url('https://webdriver.io');
await eyes.check('Main Page', Target.window().fully());
await eyes.close();
await browser.deleteSession();
})();
Run the test and view the results in the Applitools dashboard to see how your application performs across different browsers and devices.
Running Tests on Applitools Execution Cloud
Applitools Execution Cloud allows you to run Selenium WebDriver sessions remotely. To use it, request access from Applitools and update your setup:
javascript
const { remote } = require('webdriverio');
const { Eyes, VisualGridRunner, RunnerOptions, Target, Configuration, BatchInfo } = require('@applitools/eyes-webdriverio');
(async () => {
const executionCloudUrl = new URL(await Eyes.getExecutionCloudUrl());
const protocol_val = executionCloudUrl.protocol.substring(0, executionCloudUrl.protocol.length - 1);
const browser = await remote({
logLevel: 'trace',
protocol: protocol_val,
hostname: executionCloudUrl.hostname,
port: Number(executionCloudUrl.port),
capabilities: { browserName: 'chrome' }
});
const runner = new VisualGridRunner(new RunnerOptions().testConcurrency(5));
const eyes = new Eyes(runner);
const config = new Configuration();
config.setApiKey(process.env.APPLITOOLS_API_KEY);
config.setBatch(new BatchInfo('WebdriverIO Execution Cloud Test'));
eyes.setConfiguration(config);
await eyes.open(browser, 'WebdriverIO Test App', 'My Test', { width: 1200, height: 800 });
await browser.url('https://webdriver.io');
await eyes.check('Main Page', Target.window().fully());
await eyes.close();
await browser.deleteSession();
})();
Execute your tests and let Applitools Execution Cloud handle the infrastructure and locator healing.
Debugging and Troubleshooting
WebdriverIO offers robust debugging tools to help you troubleshoot issues:
Browser DevTools Integration: Use the devtools package to integrate WebdriverIO with Chrome DevTools for in-depth debugging.
Logging: Configure detailed logging to capture essential information during test execution.
Interactive Mode: Use WebdriverIO’s interactive mode to pause and inspect elements during test development.
Conclusion
WebdriverIO is a comprehensive tool for testing web applications, offering extensive features for both functional and visual testing. By integrating with Applitools, you can enhance your testing capabilities with advanced visual testing and cross-browser compatibility. Whether you are just starting with WebdriverIO or looking to refine your skills, this guide provides the knowledge and tools to succeed in your testing endeavors.
Key Takeaways
WebdriverIO Setup: Install and configure WebdriverIO for efficient testing.
Visual Testing with Applitools: Leverage Applitools for robust visual testing.
Cross-Browser Testing: Use Applitools Ultrafast Grid for quick cross-browser testing.
Execution Cloud: Utilize Applitools Execution Cloud for remote WebDriver sessions.
Debugging Tools: Take advantage of WebdriverIO’s debugging and troubleshooting features.
FAQs
What is WebdriverIO?
WebdriverIO is a JavaScript framework for automated testing of web applications, providing support for multiple browsers and seamless integration with various testing frameworks.
How do I install WebdriverIO?
Install WebdriverIO using npm with the following commands: npm init -y, npm install webdriverio @wdio/cli --save-dev, and npx wdio config.
What is Applitools?
Applitools is a visual testing platform that integrates with automation tools like WebdriverIO to provide advanced visual validation across different browsers and devices.
How can I perform cross-browser testing with WebdriverIO?
Use Applitools Ultrafast Grid to run visual tests across multiple browsers and devices efficiently. Configure your tests to include various browser types and device emulations.
What is Applitools Execution Cloud?
Applitools Execution Cloud allows you to run Selenium WebDriver sessions remotely, managing infrastructure and healing broken locators automatically.
How do I debug tests in WebdriverIO?
Use WebdriverIO’s integration with Chrome DevTools, configure detailed logging, and utilize interactive mode for in-depth debugging and troubleshooting.
Can I use WebdriverIO for mobile testing?
Yes, WebdriverIO supports mobile testing through Appium integration, allowing you to automate tests on both Android and iOS devices.
What are some best practices for writing WebdriverIO tests?
Ensure tests are independent and can run in any order, use clear and descriptive test names, and leverage visual testing tools like Applitools for comprehensive validation.
Comments