Introduction
User interface (UI) testing is a crucial aspect of ensuring the quality and usability of any iOS application. With the rapid development cycles common in today’s software industry, automated testing has become indispensable for maintaining app stability and performance. XCUITest, a powerful UI testing framework integrated with Xcode, is the go-to tool for iOS developers looking to automate their UI testing efforts.
In this comprehensive guide, we’ll explore the ins and outs of XCUITest, detailing how to effectively use it for your iOS app testing. We’ll cover everything from setting up your test environment to writing and executing UI tests, ensuring you have the knowledge to leverage XCUITest to its full potential.
What is XCUI Test?
XCUI Test is an extension of the XCTest framework, specifically designed for UI testing in iOS apps. It allows developers to write tests that simulate user interactions with the app’s interface, verifying that the app behaves as expected in various scenarios. XCUITest works by interacting with the actual UI elements of the app, making it a reliable and effective tool for ensuring that the user interface is functional and user-friendly.
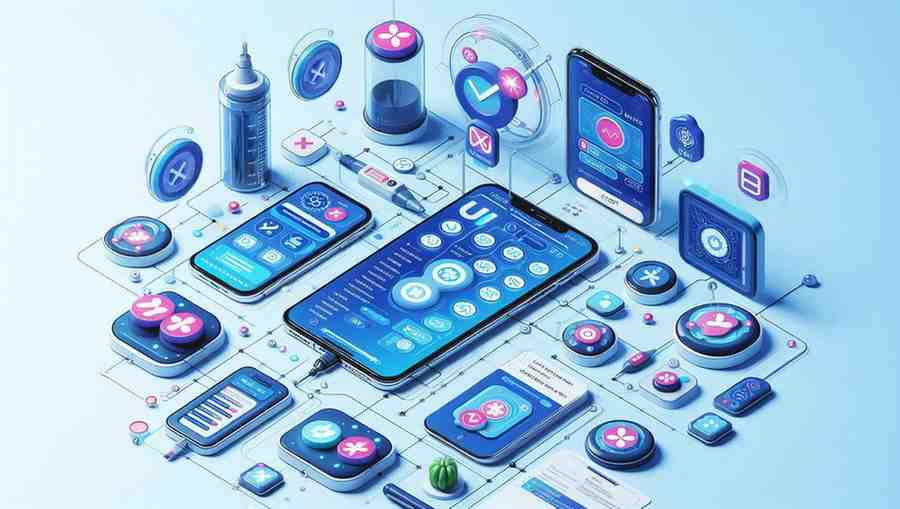
The Importance of UI Testing in iOS App Development
UI testing is vital for maintaining a high-quality user experience. It involves verifying that all elements of an app’s interface work as intended, including buttons, text fields, navigation flows, and more. Automated UI testing helps developers catch bugs early, reduce manual testing efforts, and ensure that the app provides a consistent experience across different devices and iOS versions.
By using XCUITest, developers can automate these tests, making it easier to run them frequently, such as in continuous integration/continuous deployment (CI/CD) pipelines. This automation not only saves time but also increases the reliability of the testing process, as it eliminates the potential for human error.
Setting Up XCUITest in Xcode
Getting started with XCUITest is straightforward, as it is integrated directly into Xcode, Apple’s official development environment for iOS apps. Here’s how to set up your testing environment:
1. Create a New Xcode Project
Open Xcode and create a new project or open an existing one.
Ensure that your app target includes a test target. If not, you can add one by navigating to your project settings and selecting Add Target.
2. Add a UI Testing Target
If your project does not already include a UI testing target, add one by going to File > New > Target.
Select iOS UI Testing Bundle and click Next.
Name your UI testing target and make sure it is associated with your app’s main target.
3. Install Required Dependencies
XCUITest is built into Xcode, so there’s no need to install any external dependencies. However, ensure your Xcode installation is up to date to access the latest features and improvements.
XCUITest and XCTest: Understanding the Basics
XCUITest is part of the larger XCTest framework, which is used for both unit testing and UI testing in iOS apps. The main components of XCUITest include:
XCUIApplication: A proxy for the application being tested. This class is used to launch, interact with, and terminate the app during testing.
XCUIElement: Represents a UI element in the app, such as a button, label, or text field. It provides methods for interacting with these elements, such as tapping, swiping, or typing text.
XCTestCase: The base class for all test cases in XCTest. Each UI test is a subclass of XCTestCase.
XCUIApplication
The XCUIApplication class is essential for interacting with your app during testing. It allows you to launch the app, navigate through its UI, and perform actions on various elements.
Example in Swift:
swift
let app = XCUIApplication()
app.launch()
This code snippet initializes the application object and launches the app, making it ready for testing.
XCUIElement
XCUIElement represents individual elements in the UI, such as buttons, labels, or text fields. You can perform various interactions on these elements, such as tapping or typing.
Example in Swift:
swift
let app = XCUIApplication()
let button = app.buttons["Continue"]
button.tap()
In this example, the code locates a button with the label "Continue" and simulates a tap action.
Xcode UI Test Recorder: Simplifying Test Creation
One of the standout features of XCUITest is the Xcode UI Test Recorder, a tool that allows you to generate test code by simply interacting with your app’s UI. This feature is especially useful for developers who are new to UI testing or those looking to speed up the test creation process.
Using Xcode UI Test Recorder
Open Xcode UI Test Recorder
To start recording a test, highlight a test method in your UI test class and click the record button at the bottom of the Xcode editor. The button will turn red, indicating that the recording is in progress.
Interact with the App
Once the recorder is active, interact with your app as you normally would. Every action, such as tapping a button or entering text, will be recorded and converted into test code.
Stop Recording
After completing your interactions, stop the recording. Xcode will automatically generate the corresponding test code, which you can review and edit.
Example of Recorded Test Code:
swift
func testExample() {
let app = XCUIApplication()
app.buttons["Start"].tap()
app.textFields["Name"].typeText("John Doe")
app.buttons["Submit"].tap()
}
This code was generated by simply interacting with the app’s UI during the recording session.
Implementing UI Tests with XCUITest
While the UI Test Recorder is an excellent tool for getting started, writing custom tests can provide greater control and flexibility. Here’s how to implement UI tests using XCUITest.
1. Accessing UI Elements
To access UI elements in your app, you can use various properties provided by XCUIElement. These include:
buttons: Access buttons in the UI.
textFields: Access text fields in the UI.
labels: Access labels in the UI.
Example:
swift
let app = XCUIApplication()
let continueButton = app.buttons["Continue"]
let nameField = app.textFields["Name"]
2. Performing Actions on UI Elements
XCUITest provides a range of methods for interacting with UI elements, such as tapping, typing, and swiping.
Tapping a Button:
swift
continueButton.tap()
Typing Text:
swift
nameField.tap()
nameField.typeText("John Doe")
Swiping an Element:
swift
let image = app.images["Profile Picture"]
image.swipeLeft()
3. Verifying UI Element Properties
You can also use XCUITest to verify that UI elements have the expected properties, such as labels or visibility.
Example:
swift
XCTAssertEqual(continueButton.label, "Continue")
XCTAssertTrue(nameField.exists)
These assertions check that the button’s label is "Continue" and that the text field exists in the UI.
Advanced XCUITest Features
As you become more comfortable with XCUITest, you can explore some of its more advanced features to create robust and comprehensive tests.
1. Working with XCUIElement Queries
XCUITest allows you to query for elements dynamically using predicates. This is useful when dealing with complex UI hierarchies or when elements are not always present.
Example:
swift
let predicate = NSPredicate(format: "label BEGINSWITH 'Welcome'")
let welcomeLabel = app.staticTexts.matching(predicate).element
XCTAssertTrue(welcomeLabel.exists)
This code snippet uses a predicate to find a label that begins with "Welcome" and verifies its existence.
2. Handling Alerts and Modals
XCUITest can interact with alerts and modals that appear during testing.
Example:
swift
let alert = app.alerts["Are you sure?"]
alert.buttons["Yes"].tap()
This code interacts with an alert by tapping the "Yes" button.
3. Parallel Testing on Multiple Devices
XCUITest supports parallel testing, allowing you to run your tests on multiple devices or simulators simultaneously. This is particularly useful for ensuring your app behaves consistently across different iOS versions and devices.
To run parallel tests, you can use Xcode’s xcodebuild command in the terminal with the -parallel-testing-enabled YES flag.
Best Practices for XCUITest
To get the most out of XCUITest, it’s essential to follow best practices that ensure your tests are maintainable, efficient, and reliable.
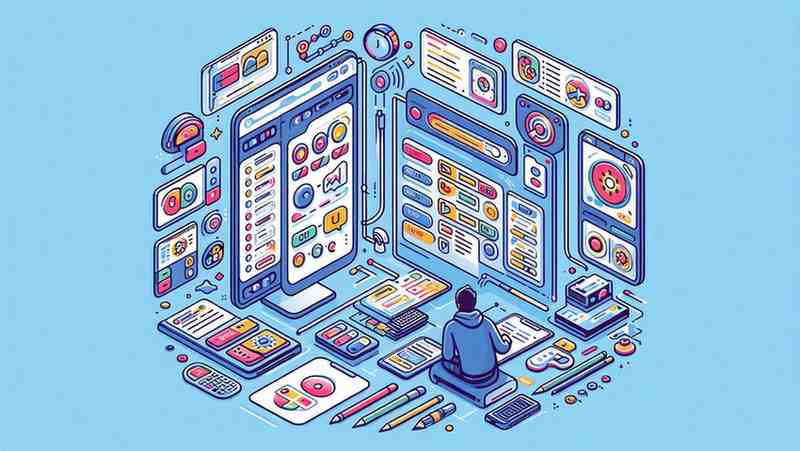
1. Keep Tests Modular and Reusable
Write tests in a modular way, so that individual components can be reused across multiple test cases. This approach not only saves time but also makes your tests easier to maintain.
2. Use Assertions Effectively
Assertions are crucial for validating the behavior of your app. Use them liberally to check that your app’s UI elements have the expected properties after interactions.
3. Avoid Hard-Coded Delays
Instead of using hard-coded delays (e.g., sleep(5)), rely on XCUITest’s built-in waiting mechanisms, such as waitForExistence(timeout:), to ensure your tests are reliable and not dependent on arbitrary timeouts.
4. Run Tests on Real Devices
While simulators are useful for initial testing, it’s important to run your tests on real devices to catch any issues that might only occur in real-world environments.
Conclusion
XCUITest is a powerful and versatile tool for automating UI testing in iOS apps. With its deep integration into Xcode, it provides developers with the ability to create, execute, and maintain comprehensive UI tests that ensure a high-quality user experience. Whether you’re a seasoned developer or new to iOS testing, mastering XCUITest can significantly improve the reliability and performance of your apps.
By following the best practices outlined in this guide, you’ll be well-equipped to leverage XCUITest to its full potential, automating your UI testing processes and delivering apps that meet the highest standards of quality.
Key Takeaways
XCUITest: Integrated with Xcode, XCUITest is essential for automating UI testing in iOS apps.
XCUIApplication & XCUIElement: These classes are the foundation of XCUITest, enabling interactions with app elements.
UI Test Recorder: Xcode’s UI Test Recorder simplifies the creation of test scripts by recording user interactions.
Advanced Features: XCUITest offers advanced capabilities, such as querying with predicates, handling alerts, and parallel testing.
Best Practices: Modular test design, effective use of assertions, avoiding hard-coded delays, and testing on real devices is crucial for success.
FAQs
1. What is XCUITest?
XCUITest is a UI testing framework integrated into Xcode that allows developers to write automated tests for iOS apps by interacting with the app's user interface.
2. How does XCUITest differ from XCTest?
XCTest is a broader testing framework that includes unit testing, while XCUITest is an extension of XCTest focused specifically on UI testing.
3. What is the purpose of the Xcode UI Test Recorder?
The Xcode UI Test Recorder allows developers to create UI test scripts by recording their interactions with the app, which are then converted into test code.
4. Can XCUITest handle dynamic UI elements?
Yes, XCUITest can handle dynamic UI elements using predicates and queries, allowing for flexible and robust test scripts.
5. What are XCUIApplication and XCUIElement?
XCUIApplication is a proxy for the app being tested, while XCUIElement represents individual UI elements within the app, such as buttons and text fields.
6. How can I run XCUITest on multiple devices simultaneously?
You can run XCUITest on multiple devices or simulators simultaneously using Xcode’s xcodebuild command with the parallel testing flag.
7. Is it necessary to run tests on real devices?
Yes, running tests on real devices is important to catch issues that might not be apparent on simulators, ensuring your app performs well in real-world environments.
8. What are some best practices for writing XCUITest scripts?
Best practices include keeping tests modular and reusable, using assertions effectively, avoiding hard-coded delays, and running tests on real devices.
Comments