Introduction
Software testing is an essential phase in the software development lifecycle, ensuring that the product meets the quality and functionality expectations. Among various testing methodologies, black box testing stands out due to its focus on the software's external behavior without considering internal code structures. This guide delves into the intricacies of black box testing, exploring its types, tools, benefits, and best practices.
What is Black Box Testing?
Black box testing is a method where testers evaluate the functionality of the software without looking into its internal code structure, implementation details, or internal paths. It treats the software as a "black box," focusing solely on input and output. This approach simulates the end-user experience, making it highly effective for detecting usability and functionality issues from a user perspective.
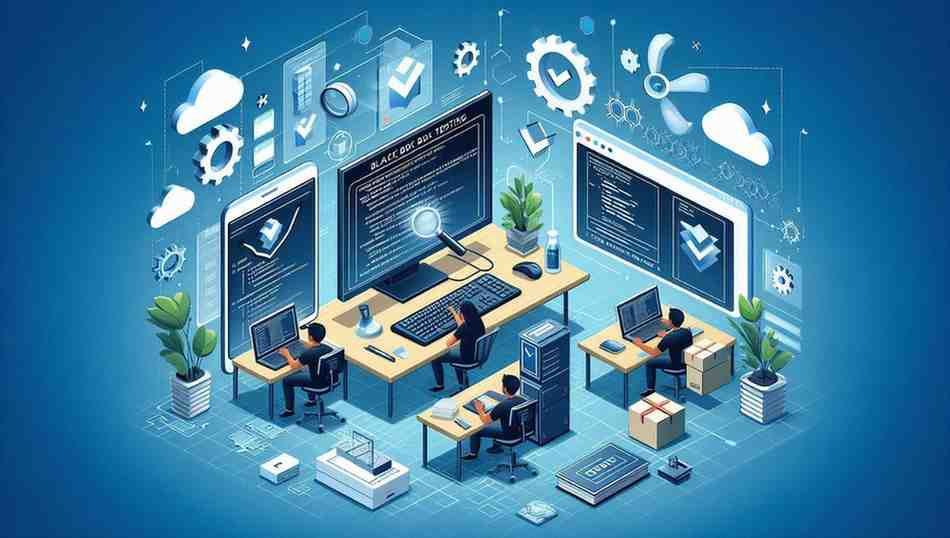
Key Characteristics of Black Box Testing:
Independent Testing: Conducted by testers who are not part of the development team, ensuring an unbiased perspective.
Requirements-Based Testing: Test cases are derived from the software's requirements and specifications.
Functional Testing Focus: Evaluate the software's functions to verify they work as expected.
No Internal Knowledge: Testers interact with the software through its user interfaces or APIs without access to source code.
Different Types of Black Box Testing
Functional Testing
Functional testing verifies that the software functions and features work as specified. Testers use functional test cases to validate inputs, outputs, and interactions without delving into the code.
Non-Functional Testing
Non-functional testing assesses aspects of the software not related to specific functions, such as performance, usability, security, scalability, and reliability.
Regression Testing
Regression testing ensures that recent changes or updates do not negatively affect existing functionality. It involves running a set of predefined test cases to check for new issues.
User Interface (UI) Testing
UI testing focuses on validating user interface elements like buttons, menus, forms, and layout, ensuring they are user-friendly and function correctly.
Usability Testing
Usability testing evaluates the software’s ease of use, navigation, visual appeal, and overall user experience.
Boundary Value Analysis (BVA)
BVA identifies defects at the boundaries of input values. Test cases are designed with values at the edges of input ranges to assess how the software handles minimum and maximum limits.
Equivalence Partitioning
This technique divides the input domain into groups that are expected to behave similarly, reducing redundant testing by focusing on representative test cases from each group.
Ad-Hoc Testing
An informal and unstructured approach where testers freely explore the software based on intuition and experience, helping to identify defects that formal test cases might miss.
Compatibility Testing
Compatibility testing evaluates how well the software performs across different environments, such as various browsers, operating systems, devices, and network configurations.
Security Testing
Security testing identifies vulnerabilities and weaknesses in the software’s security measures, simulating attacks to check for potential breaches.
Localization and Internationalization Testing
These tests ensure the software adapts to different languages, cultures, and regional settings, functioning correctly in various international environments.
Example of Black Box Testing
Let's consider a simple example of black box testing for the login functionality of a web application.
Test Case: Verify Successful Login with Valid Credentials
Test Steps:
Open the web browser.
Enter the URL of the application’s login page.
Enter a valid username in the username field.
Enter a valid password in the password field.
Click on the “Login” button.
Wait for the application to process the login request.
Expected Result: The user should be successfully logged into the application’s dashboard/homepage.
Test Case: Verify Unsuccessful Login with Invalid Credentials
Test Steps:
Open the web browser.
Enter the URL of the application’s login page.
Enter an invalid username (e.g., “invalid user”) in the username field.
Enter an invalid password (e.g., “wrong password”) in the password field.
Click on the “Login” button.
Wait for the application to process the login request.
Expected Result: The login attempt should fail, and an appropriate error message (e.g., “Invalid username or password”) should be displayed on the login page.
Features of Black Box Testing
Focus on External Behavior
Black box testing evaluates the software’s functionality from an end-user perspective, focusing on how the system behaves with different inputs and scenarios.
Independence from Internal Code
Testers do not require knowledge of the internal code or implementation details, making black-box testing suitable for testers without programming expertise.
Requirement-Based Testing
Test cases are designed based on the software’s requirements and specifications, ensuring that the application meets the intended functionality and business objectives.
Real-World Scenario Testing
Simulates real-user conditions to identify defects that might arise during actual usage of the software.
Validation of Interfaces
Effective in verifying the accuracy of the software’s interfaces, ensuring correct handling of inputs and outputs.
Identification of Interface-Level Bugs
Useful for detecting interface-level bugs such as incorrect error messages, data handling issues, or missing functionality.
User-Centric Testing
Ensures the application meets user expectations and delivers a satisfactory user experience by focusing on the end-user perspective.
Test Case Design Techniques
Employs various test case design techniques like equivalence partitioning, boundary value analysis, decision table testing, and state transition testing to ensure comprehensive test coverage.
Compatibility Testing
Assesses the software’s compatibility with different environments, browsers, operating systems, and devices.
Test Automation Support
Many black box testing tools support test automation, enabling efficient execution of repetitive test cases and reducing the testing cycle time.
Advantages and Limitations of Black Box Testing
Advantages
Independence from Internal Implementation: Suitable for non-technical testers who do not need access to the source code.
User-Centric Testing: Ensures the software meets user requirements and expectations by simulating real user scenarios.
Early Detection of Interface Issues: Uncovers interface-related defects like input validation errors and output discrepancies.
Effective at Integration Testing: Verifies interactions between different system components.
Test Case Design Flexibility: Various techniques like equivalence partitioning and boundary value analysis allow for effective test coverage.
Effective for Requirement Validation: Helps validate that the software meets the specified requirements.
Suitable for Large Projects: Scalable for different testing levels, from unit testing to acceptance testing.
Limitations
Limited Code Coverage: May not explore all possible code paths or internal logic, potentially leaving certain defects undetected.
Inability to Test Complex Algorithms: Not effective at validating complex algorithms or intricate business logic requiring internal code knowledge.
Redundant Testing: Some test cases may overlap, leading to redundant efforts.
Dependency on Requirements: Relies heavily on the accuracy and completeness of the provided requirements.
Inefficiency with Repetitive Tasks: Manual black box testing can be time-consuming and inefficient for repetitive tasks.
Inability to Assess Performance and Scalability: Performance-related issues and scalability problems may not be effectively identified.
Difficulty in Error Localization: Identifying the root cause of defects can be challenging without access to internal code.
Limited Security Testing: This may not comprehensively address all potential security issues.
Tools and Frameworks Used to Perform Black Box Testing
Selenium
Selenium is an open-source framework for automating web applications. It allows testers to automate browser interactions, simulating real user interactions and validating functionality without accessing internal code.
Appium
Appium is an open-source automation framework for testing native, hybrid, and mobile web applications on Android and iOS. It enables black box testing of mobile apps without accessing the internal code.
Cypress
Cypress is a test automation framework primarily used for front-end testing, including end-to-end (E2E) testing and user interface (UI) testing. It combines both black box and white box testing capabilities.
LoadRunner
LoadRunner is a performance testing tool that can simulate real user scenarios and interactions with the application. It helps in load testing and user experience testing from an end-user perspective.
SoapUI
SoapUI is an API testing tool that focuses on testing the functionality and behavior of APIs. It allows testers to create test cases that simulate API requests and responses without knowing the internal implementation.
Difference Between Black Box and White Box Testing
What is White Box Testing?
White box testing, also known as clear box testing or structural testing, involves examining and validating the internal code and logic of a software application. Testers have access to the source code and use this knowledge to design and execute test cases, verifying the correctness of the code and identifying logical errors.
Key Differences:
Code Access: White box testing requires access to the internal code, whereas black box testing does not.
Focus: White box testing focuses on internal code structure and logic, while black box testing focuses on external behavior.
Test Case Design: White box testing uses techniques like code coverage analysis and branch coverage, while black box testing uses techniques like equivalence partitioning and boundary value analysis.
Knowledge Required: White box testing requires programming knowledge, while black box testing can be performed by non-technical testers.
Testing Levels: White box testing is typically performed at unit and integration testing levels, whereas black box testing can be applied at various levels, including unit, integration, system, and acceptance testing.
Objective: White box testing aims to verify internal code correctness, while black box testing aims to validate software functionality from an end-user perspective.
Best Practices for Black Box Testing
Requirement Analysis
Thoroughly understand the software’s requirements and specifications to guide the creation of meaningful test cases.
Test Planning
Develop a comprehensive test plan outlining the testing scope, objectives, levels, resources, and timelines.
Test Case Design Techniques
Utilize various test case design techniques like equivalence partitioning, boundary value analysis, decision tables, and state transition testing to ensure comprehensive test coverage.
Test Data Management
Prepare diverse and relevant test data to cover various scenarios, including both valid and invalid inputs.
Positive and Negative Testing
Include test cases for both positive scenarios (valid inputs) and negative scenarios (invalid inputs).
Usability Testing
Focus on testing the user interface and overall user experience, ensuring the application is user-friendly and consistent.
Regression Testing
Perform regression testing to ensure that updates or fixes do not introduce new defects or impact existing functionality.
Boundary Value Analysis
Test the software’s behavior around input range boundaries to identify potential issues with boundary conditions.
Error Localization and Reporting
Clearly document and report any defects discovered during testing, including detailed steps to reproduce the problem.
Test Automation
Automate repetitive and time-consuming test cases to improve testing efficiency and repeatability.
Conclusion
Black box testing is a critical component of software quality assurance, focusing on the external behavior of the software to ensure it meets user expectations and requirements. By simulating real-world scenarios and user interactions, black box testing helps identify defects that might be missed by other testing approaches. Despite its limitations, when combined with white box testing and other testing strategies, it provides a comprehensive approach to software testing, enhancing the overall quality and reliability of the software.
Key Takeaways
User-Centric Approach: Black box testing focuses on the software’s external behavior, ensuring it meets user expectations.
Independent Testing: Suitable for testers without programming knowledge, providing an unbiased perspective.
Comprehensive Coverage: Uses various test case design techniques to ensure thorough testing of functionalities.
Early Detection: Helps identify interface-level bugs and usability issues early in the development process.
Scalability: Applicable at different testing levels, making it suitable for large projects.
Combined Approach: Best used in combination with white box testing for a more comprehensive testing strategy.
FAQs
What is black box testing?
Black box testing is a method where testers evaluate the software's functionality without looking into its internal code structure or implementation details. It focuses on the external behavior of the software.
What are the types of black box testing?
The main types of black box testing include functional testing, non-functional testing, regression testing, UI testing, usability testing, boundary value analysis, equivalence partitioning, ad-hoc testing, compatibility testing, security testing, and localization and internationalization testing.
What tools are used for black box testing?
Popular tools for black box testing include Selenium, Appium, Cypress, LoadRunner, and SoapUI.
How does black box testing differ from white box testing?
Black box testing focuses on external behavior without accessing internal code, while white box testing involves examining the internal code and logic. Black box testing is user-centric, while white box testing is code-centric.
What are the advantages of black box testing?
Advantages include independence from internal code, user-centric testing, early detection of interface issues, effective integration testing, flexible test case design, and suitability for large projects.
What are the limitations of black box testing?
Limitations include limited code coverage, inability to test complex algorithms, redundant testing, dependency on requirements, inefficiency with repetitive tasks, difficulty in error localization, and limited security testing.
Can black box testing be automated?
Yes, many black box testing tools support test automation, enabling efficient execution of repetitive test cases and reducing the testing cycle time.
Why is black box testing important?
Black box testing is important because it ensures that the software meets user expectations and requirements, identifies usability and functionality issues early, and provides an unbiased testing perspective.
Comments