Introduction
In the realm of JavaScript development, module systems play a crucial role in organizing and managing code. One of the prominent solutions that emerged to bridge the gap between different module formats is Universal Module Definition (UMD). This comprehensive guide explores what UMD is, its benefits, how it compares to other module systems like CommonJS, AMD, and ESM, and practical examples of its implementation.
What is Universal Module Definition (UMD)?
Universal Module Definition (UMD) is a versatile module format that aims to provide compatibility across different environments, including both browsers and server-side applications. Unlike purely browser-centric solutions like AMD or server-specific formats like CommonJS, UMD offers a unified approach. It checks the environment and adapts accordingly, making it suitable for various scenarios without requiring major adjustments.
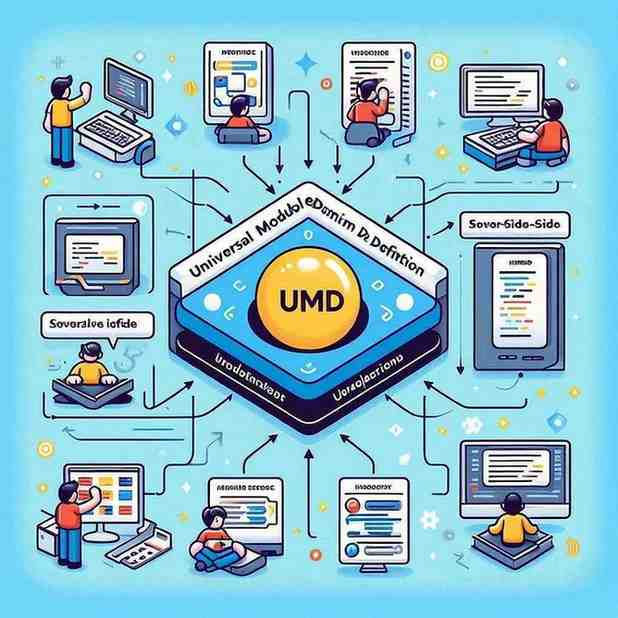
Benefits of Universal Module Definition (UMD)
UMD brings several advantages to JavaScript developers:
Compatibility: Supports both browser and server-side environments, making modules usable across different platforms.
Flexibility: Allows developers to choose between AMD, CommonJS, or global exports based on the environment, ensuring maximum compatibility.
Integration: Seamlessly integrates with existing module systems like CommonJS and AMD, enabling interoperability without extensive modifications.
How Universal Module Definition (UMD) Works
UMD achieves its universality through a specific pattern:
javascript:
(function (root, factory) {
if (type of define === 'function' && define.amd) {
// AMD module format
define(['dependency'], factory);
} else if (type of exports === 'object') {
// CommonJS module format
module.exports = factory(require('dependency'));
} else {
// Global variable (browser window)
root.ModuleName = factory(root.Dependency);
}
}(this, function (Dependency) {
// Module implementation
return {
// Exported functionality
};
}));
In this structure:
This approach ensures that the module can be used across different environments seamlessly.
Comparing Universal Module Definition (UMD) with Other Module Systems
CommonJS (CJS): Synchronous module system primarily used in Node.js for server-side applications.
Asynchronous Module Definition (AMD): Designed for browser-based asynchronous module loading, primarily used with tools like RequireJS.
ECMAScript Module (ESM): Standardized module system in modern JavaScript, supported natively by browsers and Node.js with import and export keywords.
UMD stands out by providing a hybrid approach that accommodates both synchronous and asynchronous loading, catering to diverse development needs.
Practical Examples of Universal Module Definition (UMD) Usage
javascript:
// Example using UMD format with a library like jQuery
(function (root, factory) {
if (type of define === 'function' && define.amd) {
define(['jquery'], function ($) {
return factory($);
});
} else if (typeof exports === 'object') {
module.exports = factory(require('jquery'));
} else {
root.MyLibrary = factory(root.jQuery);
}
}(typeof self !== 'undefined' ? self: this, function ($) {
// Module implementation using jQuery
return {
// Exported functionality
};
}));
This example demonstrates how UMD can integrate with jQuery, adapting to different module loading strategies based on the environment.
Conclusion
Universal Module Definition (UMD) bridges the gap between different JavaScript module systems by offering a flexible and interoperable format. Whether you're developing for browsers, server-side environments, or both, UMD provides a reliable solution. By understanding its structure, benefits, and applications, developers can effectively manage dependencies and enhance code modularity across diverse projects.
Key Takeaways from Universal Module Definition (UMD) Guide:
Versatility Across Environments: UMD serves as a versatile module format compatible with both browser and server-side environments, accommodating various JavaScript development needs without significant modifications.
Integration and Compatibility: It seamlessly integrates with existing module systems like AMD and CommonJS, providing flexibility to developers in choosing loading strategies based on the environment.
Adaptive Loading Strategy: UMD's conditional pattern ensures modules-load synchronously or asynchronously depending on the environment, optimizing performance and compatibility.
Continued Relevance: Despite the adoption of ECMAScript Modules (ESM), UMD remains relevant for projects requiring broad compatibility across different JavaScript environments, including legacy browsers and Node.js versions.
Practical Application: Developers can utilize UMD effectively in projects where interoperability and flexibility in module loading are essential, leveraging its structured approach to enhance code modularity and maintainability.
FAQs about Universal Module Definition (UMD)
Q: When should I use UMD?
A: UMD is ideal when you need a module format that works across different environments without requiring major adjustments.
Q: Can UMD modules be used with modern bundlers like Webpack or Rollup?
A: Yes, modern bundlers can handle UMD modules effectively. They can bundle UMD modules into a format suitable for deployment in browsers or Node.js.
Q: Is UMD still relevant with the rise of ECMAScript Modules (ESM)?
A: Yes, UMD remains relevant, especially for libraries and projects needing compatibility across a wide range of environments, including older browsers and Node.js versions.
Article Sources
Halil Atilla, "Explaining CJS vs AMD vs UMD vs ESM", Medium, Mar 16, 2023
"What Are CJS, AMD, UMD, and ESM in JavaScript?", July 21, 2019
Understanding Universal Module Definition (UMD) in JavaScript - SitePoint
UMD: The Universal Module Definition - GitHub repository for UMD
UMD Patterns - Universal Module Definition - David B. Calhoun's blog
This comprehensive guide equips you with the knowledge to leverage Universal Module Definition (UMD) effectively in your JavaScript projects, ensuring compatibility and flexibility across different platforms.
Comments